资源简介
本科毕业设计 用VC++实现工业组态软件一些常用的控件如指示灯、按钮、温度计、压力计等
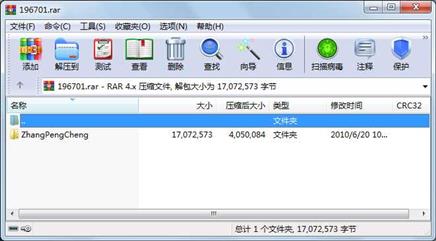
代码片段和文件信息
// 3DMeterCtrl.cpp : implementation file
//
#include “stdafx.h“
#include “math.h“
#include “3DMeterCtrl.h“
#include “MemDC.h“
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// C3DMeterCtrl
C3DMeterCtrl::C3DMeterCtrl()
{
m_dCurrentValue = 0.0 ;
m_dMaxValue = 5.0 ;
m_dMinValue = -5.0 ;
m_nScaleDecimals = 1 ;
m_nValueDecimals = 3 ;
m_strUnits.Format(“Volts“) ;
m_colorNeedle = RGB(255 0 0) ;
}
C3DMeterCtrl::~C3DMeterCtrl()
{
if ((m_pBitmapOldBackground) &&
(m_bitmapBackground.GetSafeHandle()) &&
(m_dcBackground.GetSafeHdc()))
m_dcBackground.Selectobject(m_pBitmapOldBackground);
}
BEGIN_MESSAGE_MAP(C3DMeterCtrl CStatic)
//{{AFX_MSG_MAP(C3DMeterCtrl)
ON_WM_PAINT()
ON_WM_SIZE()
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// C3DMeterCtrl message handlers
void C3DMeterCtrl::OnPaint()
{
CPaintDC dc(this); // device context for painting
// Find out how big we are
GetClientRect (&m_rectCtrl) ;
// make a memory dc
CMemDC memDC(&dc &m_rectCtrl);
// set up a memory dc for the background stuff
// if one isn‘t being used
if ((m_dcBackground.GetSafeHdc() == NULL) || (m_bitmapBackground.m_hobject == NULL))
{
m_dcBackground.CreateCompatibleDC(&dc) ;
m_bitmapBackground.CreateCompatibleBitmap(&dc m_rectCtrl.Width()
m_rectCtrl.Height()) ;
m_pBitmapOldBackground = m_dcBackground.Selectobject(&m_bitmapBackground) ;
// Fill this bitmap with the background.
// Note: This requires some serious drawing and calculating
// therefore it is drawn “once“ to a bitmap and
// the bitmap is stored and blt‘d when needed.
DrawMeterBackground(&m_dcBackground m_rectCtrl) ;
}
// drop in the background
memDC.BitBlt(0 0 m_rectCtrl.Width() m_rectCtrl.Height()
&m_dcBackground 0 0 SRCCOPY) ;
// add the needle to the background
DrawNeedle(&memDC) ;
// add the value to the background
DrawValue(&memDC) ;
}
void C3DMeterCtrl::DrawValue(CDC *pDC)
{
CFont *pFontOld ;
CString strTemp ;
// Pick up the font.
// Note: the font was determined in the drawing
// of the background
pFontOld = pDC->Selectobject(&m_fontValue) ;
// set the colors based on the system colors
pDC->SetTextColor(m_colorText) ;
pDC->SetBkColor(m_colorButton) ;
// draw the text in the recessed rectangle
pDC->SetTextAlign(TA_CENTER|TA_baseLINE) ;
strTemp.Format(“%.*f“ m_nValueDecimals m_dCurrentValue) ;
pDC->TextOut(m_nValueCenter m_nValuebaseline strTemp) ;
// restore the color and the font
pDC->SetBkColor(m_colorWindow) ;
pDC->Selectobject(pFontOld) ;
}
void C3DMeterCtrl::UpdateNeedle(double dValue)
{
m_dCurrentValue = dValue ;
Invalidate() ;
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 15555 2010-05-28 09:38 ZhangPengCheng\3DMeterCtrl.cpp
文件 2452 2010-05-28 09:38 ZhangPengCheng\3DMeterCtrl.h
文件 7991 2010-05-28 14:27 ZhangPengCheng\Compass.cpp
文件 1834 2010-05-28 14:27 ZhangPengCheng\Compass.h
文件 46704 2010-06-20 10:34 ZhangPengCheng\Debug\3DMeterCtrl.obj
文件 0 2010-06-20 10:34 ZhangPengCheng\Debug\3DMeterCtrl.sbr
文件 24913 2010-06-20 10:34 ZhangPengCheng\Debug\Compass.obj
文件 0 2010-06-20 10:34 ZhangPengCheng\Debug\Compass.sbr
文件 22492 2010-06-20 10:34 ZhangPengCheng\Debug\DynamicLED.obj
文件 0 2010-06-20 10:34 ZhangPengCheng\Debug\DynamicLED.sbr
文件 57390 2010-06-20 10:34 ZhangPengCheng\Debug\Meter.obj
文件 0 2010-06-20 10:34 ZhangPengCheng\Debug\Meter.sbr
文件 4782 2010-06-20 10:34 ZhangPengCheng\Debug\PIDControl.obj
文件 0 2010-06-20 10:34 ZhangPengCheng\Debug\PIDControl.sbr
文件 78061 2010-06-20 10:34 ZhangPengCheng\Debug\RoundSliderCtrl.obj
文件 0 2010-06-20 10:34 ZhangPengCheng\Debug\RoundSliderCtrl.sbr
文件 43265 2010-06-20 10:35 ZhangPengCheng\Debug\ScaleUnit.obj
文件 0 2010-06-20 10:35 ZhangPengCheng\Debug\ScaleUnit.sbr
文件 37050 2010-06-20 10:34 ZhangPengCheng\Debug\StaticTime.obj
文件 0 2010-06-20 10:34 ZhangPengCheng\Debug\StaticTime.sbr
文件 106080 2010-06-20 10:34 ZhangPengCheng\Debug\StdAfx.obj
文件 1374958 2010-06-20 10:34 ZhangPengCheng\Debug\StdAfx.sbr
文件 16654 2010-06-20 10:34 ZhangPengCheng\Debug\SwitchStatic.obj
文件 0 2010-06-20 10:34 ZhangPengCheng\Debug\SwitchStatic.sbr
文件 238592 2010-06-20 10:40 ZhangPengCheng\Debug\vc60.idb
文件 380928 2010-06-20 10:39 ZhangPengCheng\Debug\vc60.pdb
文件 5481472 2010-06-20 10:39 ZhangPengCheng\Debug\ZhangPengCheng.bsc
文件 315493 2010-06-20 10:39 ZhangPengCheng\Debug\ZhangPengCheng.exe
文件 513652 2010-06-20 10:39 ZhangPengCheng\Debug\ZhangPengCheng.ilk
文件 19453 2010-06-20 10:35 ZhangPengCheng\Debug\ZhangPengCheng.obj
............此处省略58个文件信息
相关资源
- VC++ 多线程文件读写操作
- 移木块游戏,可以自编自玩,vc6.0编写
- VC++MFC小游戏实例教程(实例)+MFC类库
- VC++实现CMD命令执行与获得返回信息
- VC++基于OpenGL模拟的一个3维空间模型
- 基于VC++的SolidWorks二次开发SolidWorks
- 派克变换VC++源码(附文档)
- VC++ 串口
- VC++ 大富翁4_大富翁游戏源码
- VC++ 摄像头视频采集与回放源程序
- 转 VC++ 实现电子邮件(Email)发送
- 基于MFC的VC++仿QQ浏览器源码(雏形)
- VC++ 服务程序编写及安装与卸载
- VC++6.0番茄西红柿VAXvirsual assist X完美破
- 基于改进的fcm算法的图像分割vc++
- VC++6.0 绿色版,免安装,非常好用。
- Microsoft Visual C++ 2005 Redistributable Pack
- VC++MFC课程设计的学生成绩管理系统
- 大智慧365DLL插件设计
- VC++6.0汉化包
- VC++完整商业界面源码(再上传)
- VC++编程技术600个大型项目源码.rar
- VC++实现RSA加密算法
- VC++ 中国象棋经典游戏源代码
- 郁金香VC++游戏辅助视频教程
- C语言进阶源码---基于graphics实现图书
- 摄影测量相对定向VC++程序
- VC++数字图像处理典型算法及实现
- VC++酒店客房管理系统 MFC
- 车站计算机联锁vc++6.0程序代码
评论
共有 条评论