资源简介
GerberView源码,原来用vc6编译的,需要配置gtk+环境,亲测可正常运行。现在用vs2010打开,配置环境后,也可以正常运行。gtk+下载:(32bit https://download.csdn.net/download/wyq429703159/10437429,64bit https://download.csdn.net/download/wyq429703159/10437422),有需求,我们可以在交流,留言即可
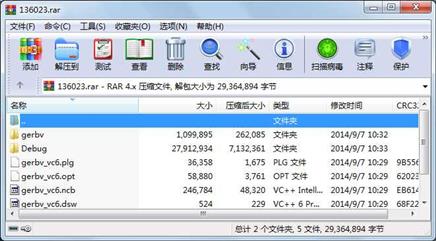
代码片段和文件信息
/*
* gEDA - GNU Electronic Design Automation
* This files is a part of gerbv.
*
* Copyright (C) 2000-2002 Stefan Petersen (spe@stacken.kth.se)
*
* $Id$
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not write to the Free Software
* Foundation Inc. 59 Temple Place Suite 330 Boston MA 02111 USA
*/
/** \file amacro.c
\brief Aperture macro parsing functions
\ingroup libgerbv
*/
#include
#include
#include
#include “gerbv.h“
#include “gerb_file.h“
#include “amacro.h“
/*
* Allocates a new instruction structure
*/
static gerbv_instruction_t *
new_instruction(void)
{
gerbv_instruction_t *instruction;
instruction = (gerbv_instruction_t *)malloc(sizeof(gerbv_instruction_t));
if (instruction == NULL) {
free(instruction);
return NULL;
}
memset(instruction 0 sizeof(gerbv_instruction_t));
return instruction;
} /* new_instruction */
/*
* Allocates a new amacro structure
*/
static gerbv_amacro_t *
new_amacro(void)
{
gerbv_amacro_t *amacro;
amacro = (gerbv_amacro_t *)malloc(sizeof(gerbv_amacro_t));
if (amacro == NULL) {
free(amacro);
return NULL;
}
memset(amacro 0 sizeof(gerbv_amacro_t));
return amacro;
} /* new_amacro */
/*
* Defines precedence of operators used in aperture macros
*/
static int
math_op_prec(gerbv_opcodes_t math_op)
{
switch (math_op) {
case GERBV_OPCODE_ADD:
case GERBV_OPCODE_SUB:
return 1;
case GERBV_OPCODE_MUL:
case GERBV_OPCODE_DIV:
return 2;
default:
;
}
return 0;
} /* math_op_prec */
/*
* Operations on the operator stack. The operator stack is used in the
* “shunting yard algorithm“ to achive precedence.
* Aperture macros has a very limited set of operators and matching precedence
* so it is solved by a small array and an index to that array.
*/
#define MATH_OP_STACK_SIZE 2
#define MATH_OP_PUSH(val) math_op[math_op_idx++] = val
#define MATH_OP_POP math_op[--math_op_idx]
#define MATH_OP_TOP (math_op_idx > 0)?math_op[math_op_idx - 1]:GERBV_OPCODE_NOP
#define MATH_OP_EMPTY (math_op_idx == 0)
/*
* Parses the definition of an aperture macro
*/
gerbv_amacro_t *
parse_aperture_macro(gerb_file_t *fd)
{
gerbv_amacro_t *amacro;
gerbv_instruction_t *ip = NULL;
int primitive = 0 c found_primitive = 0;
gerbv_opcodes_t math_op[MATH_OP_STACK_SIZE];
int math_op_idx = 0;
int comma = 0; /* Just read
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 9519 2014-09-07 10:29 gerbv_vc6.dsp
文件 524 2014-09-07 10:29 gerbv_vc6.dsw
文件 246784 2014-09-07 10:29 gerbv_vc6.ncb
文件 58880 2014-09-07 10:29 gerbv_vc6.opt
文件 36358 2014-09-07 10:29 gerbv_vc6.plg
文件 14931 2014-09-07 10:29 Debug\dynload.obj
文件 1022462 2014-09-07 10:30 Debug\dynload.sbr
文件 10380 2014-09-07 10:30 Debug\gerbv.res
文件 8414 2014-09-07 10:30 Debug\getopt.obj
文件 10507 2014-09-07 10:30 Debug\getopt.sbr
文件 91682 2014-09-07 10:30 Debug\libasprintf-0.dll
文件 215280 2014-09-07 10:30 Debug\libatk-1.0-0.dll
文件 972041 2014-09-07 10:30 Debug\libcairo-2.dll
文件 73310 2014-09-07 10:30 Debug\libcairo-gob
文件 208739 2014-09-07 10:30 Debug\libcairo-sc
文件 52957 2014-09-07 10:30 Debug\libcharset-1.dll
文件 338000 2014-09-07 10:30 Debug\libcroco-0.6-3.dll
文件 83622 2014-09-07 10:30 Debug\libffi-6.dll
文件 347810 2014-09-07 10:30 Debug\libfontconfig-1.dll
文件 752539 2014-09-07 10:30 Debug\libfreetype-6.dll
文件 93389 2014-09-07 10:30 Debug\libgailutil-3-0.dll
文件 870852 2014-09-07 10:30 Debug\libgdk-3-0.dll
文件 356005 2014-09-07 10:30 Debug\libgdk_pixbuf-2.0-0.dll
文件 1401627 2014-09-07 10:32 Debug\libgettextlib-0-18-2.dll
文件 398632 2014-09-07 10:30 Debug\libgettextpo-0.dll
文件 320913 2014-09-07 10:30 Debug\libgettextsrc-0-18-2.dll
文件 1789609 2014-09-07 10:32 Debug\libgio-2.0-0.dll
文件 1513572 2014-09-07 10:32 Debug\libglib-2.0-0.dll
文件 64399 2014-09-07 10:30 Debug\libgmodule-2.0-0.dll
文件 403969 2014-09-07 10:30 Debug\libgob
............此处省略107个文件信息
相关资源
- 基于MFC的TCP调试助手源码95706
- 移木块游戏,可以自编自玩,vc6.0编写
- C++纯文字DOS超小RPG游戏
- MFC数字钟(基于VC6.0)
- 安科瑞智能电能表MODBUS通讯程序 VC6
- VC6LineNumberAddin.dll
- 用VC6.0实现多边形扫描线填充算法
- VC助手 VC6.0助手
- 九齐单片机源码
- Qt画图工具源码(qgraphics draw)
- qt 串口助手源码
- modbus 主机源码
- 《LINUX C编程从初学到精通》光盘源码
- OLED驱动源码
- tm1650+stm32f103源码(board_tm1650.c)
- cheat engine 7.2源码
- CrySearch内存搜索器源码
- FTP客户端源码(c++)
- MFC视频播放器源码(支持avi/wma/mp3等格
- CreatBitmap图片合成源码
- vs2008 can总线通讯源码
- 宠物管理系统课程设计(源码+数据库
- Windows扩展命令程序(源码)
- c语言实现火车订票系统(控制台)源
- 鼠标连点器(附源码)
- c++ 简易贪吃蛇源码
- 杀毒软件源码
- 经典外汇智能交易程序Amazing3.1源码(
- 微型文件系统源码(FatFs)
- 海康私有流分析接口源码(附使用说
评论
共有 条评论