资源简介
VS13 MFC工程代码, 示例如何使用glfw通过opengl显示xyz文件以及stl文件. 代码中使用到的glfw是进过稍微修改过后的, 可以直接支持将创建的窗口集成到MFC控件中. 代码结构清晰, 使用示例简单. 详情可以查看博客: http://blog.csdn.net/sunbibei/article/details/51783783
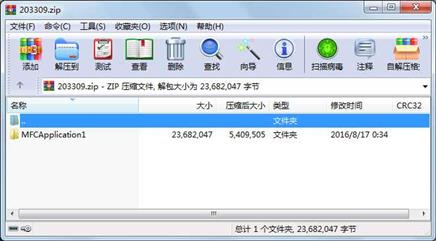
代码片段和文件信息
#include “stdafx.h“
#include “CloudWindow.h“
std::atomic g_cloud_window_scale_ = 1.0;
static void glfwScrollfun(GLFWwindow* window double xoffset double yoffset) {
std::cout << “GLFWscrollfun: “ << xoffset << “ “ << yoffset << std::endl;
g_cloud_window_scale_ = g_cloud_window_scale_ + 0.1*yoffset;
if (g_cloud_window_scale_ < 0) g_cloud_window_scale_ = 0;
}
CloudWindow::CloudWindow() {
this->is_open_ = false;
this->close_window_.clear();
this->lock_data_access_.clear();
this->glfw_points_.clear();
}
CloudWindow::~CloudWindow(){
this->Close();
}
void CloudWindow::Close(){
if (this->IsOpen()){
// Tell window to close
while (this->close_window_.test_and_set()){}
// Wait for window loop to finish processing
while (this->lock_data_access_.test_and_set()){}
// Clear all window data
this->is_open_ = false;
this->glfw_points_.clear();
this->lock_data_access_.clear();
this->close_window_.clear();
}
}
bool CloudWindow::Open(const std::string &title const unsigned int &width const unsigned int &height int hparent) { // Sized empty window
if (!this->IsOpen()){
this->is_open_ = true;
// If width or height equal 0 open fullscreen
if ((width == 0) || (height == 0)){
std::thread window_loop(&CloudWindow::Loop this title true 0 0 hparent);
window_loop.detach();
}
else{
// Open the window to a specific size
std::thread window_loop(&CloudWindow::Loop this title false width height hparent);
window_loop.detach();
}
// Wait 50ms and check that window opened
std::this_thread::sleep_for(std::chrono::milliseconds(50));
if (!this->is_open_) return false;
}
return true;
}
bool CloudWindow::Update(const std::vector& _cloud) {
// Check that window is open and the point cloud has points
if ((!this->IsOpen()) || (_cloud.size() == 0))
return false;
// the number of cloud is same don‘t need to update.
if (glfw_points_.size() == _cloud.size()) {
return true;
}
// Set the flag to copy the data
while (this->lock_data_access_.test_and_set()) {}
// Clear the old data
this->glfw_points_.clear();
// Grab the first point to start the min max calculations
this->limited[_X][_MIN] = _cloud[0].xx;
this->limited[_X][_MAX] = _cloud[0].xx;
this->limited[_Y][_MIN] = _cloud[0].yy;
this->limited[_Y][_MAX] = _cloud[0].yy;
this->limited[_Z][_MIN] = _cloud[0].zz;
this->limited[_Z][_MAX] = _cloud[0].zz;
this->limited[_X][_AVE] = 0;
this->limited[_Y][_AVE] = 0;
this->limited[_Z][_AVE] = 0;
// Find the minimum maximum and average
for (auto pt : _cloud) {
if (this->limited[_X][_MIN] > pt.xx) this->limi
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2016-08-17 00:34 MFCApplication1\
目录 0 2016-08-16 23:51 MFCApplication1\glfw\
文件 100864 2016-08-05 17:18 MFCApplication1\glfw\glfw3.dll
文件 17966 2016-08-05 17:18 MFCApplication1\glfw\glfw3dll.lib
目录 0 2016-08-16 23:51 MFCApplication1\glfw\include\
目录 0 2016-08-16 23:51 MFCApplication1\glfw\include\GLFW\
文件 82334 2016-06-29 15:52 MFCApplication1\glfw\include\GLFW\glfw3.h
文件 5684 2015-01-28 00:33 MFCApplication1\glfw\include\GLFW\glfw3native.h
目录 0 2016-08-16 23:51 MFCApplication1\glfw\lib-vc2013\
文件 100864 2016-08-05 17:18 MFCApplication1\glfw\lib-vc2013\glfw3.dll
文件 238850 2016-06-02 21:54 MFCApplication1\glfw\lib-vc2013\glfw3.lib
文件 17966 2016-08-05 17:18 MFCApplication1\glfw\lib-vc2013\glfw3dll.lib
文件 242802 2005-10-19 15:55 MFCApplication1\glfw\lib-vc2013\opengl.lib
文件 336274 2005-10-19 15:55 MFCApplication1\glfw\lib-vc2013\OPENGL32.LIB
目录 0 2016-08-17 00:34 MFCApplication1\MFCApplication1\
文件 991 2016-08-16 23:48 MFCApplication1\MFCApplication1.sln
文件 30208 2016-08-17 00:34 MFCApplication1\MFCApplication1.v12.suo
文件 13917 2016-08-17 00:24 MFCApplication1\MFCApplication1\CloudWindow.cpp
文件 1611 2016-08-16 23:56 MFCApplication1\MFCApplication1\CloudWindow.h
目录 0 2016-08-17 00:33 MFCApplication1\MFCApplication1\data\
文件 509183 2016-08-10 11:30 MFCApplication1\MFCApplication1\data\01.xyz
文件 601070 2016-08-10 11:38 MFCApplication1\MFCApplication1\data\02.xyz
文件 429750 2016-08-10 11:39 MFCApplication1\MFCApplication1\data\03.xyz
文件 757449 2016-08-10 11:39 MFCApplication1\MFCApplication1\data\04.xyz
文件 454791 2016-08-10 11:39 MFCApplication1\MFCApplication1\data\05.xyz
文件 947131 2016-08-10 11:40 MFCApplication1\MFCApplication1\data\06.xyz
文件 955730 2016-08-10 11:40 MFCApplication1\MFCApplication1\data\07.xyz
文件 589941 2016-08-10 11:40 MFCApplication1\MFCApplication1\data\08.xyz
文件 488953 2016-08-10 11:40 MFCApplication1\MFCApplication1\data\09.xyz
文件 436933 2016-08-10 11:41 MFCApplication1\MFCApplication1\data\10.xyz
文件 390835 2016-08-10 11:41 MFCApplication1\MFCApplication1\data\11.xyz
............此处省略40个文件信息
相关资源
- 基于MFC的TCP调试助手源码95706
- 基于mfc的多线程文件传输
- MFC数字钟(基于VC6.0)
- VC++MFC小游戏实例教程(实例)+MFC类库
- ChartCtrl控件库(可在VS2019中使用)
- 商品库存管理系统 C++ MFC
- mfc 调用redis
- MFC视频播放器源码(支持avi/wma/mp3等格
- mfc绘图大全(画直线、矩形、椭圆)
- MFC控件重绘
- hook,捕获所有案件,查找所有窗口,
- (学习)VS2010之MFC入门到精通教程
- C++ STL实现
- MFC文档_视图_框架_模板结构体系深入
- 简单员工管理系统(适合初学MFC)
- MFC五子棋游戏
- MFC UDP编程
- MFC的异步网络通讯应用程序
- C++MFC模块讲解,黑发程序员课程整理
- 一个简单而强大的基于MFC的web server源
- 基于MFC的VC++仿QQ浏览器源码(雏形)
- MFCaccess.rar
- VC++MFC课程设计的学生成绩管理系统
- MFC 日历控件 万年历 Calendar自绘
- CrystalDiskInfo-HDD/SSD硬盘信息,SMART信息
- MFC自定义界面HUI,高效简单,含详细
- 仿射密码-Affine cipher
- c++MFC车牌自动识别定位,只能定位和
- mfc+sql 酒店客房管理系统
- 基于图割的图像分割OpenCV+MFC实现
评论
共有 条评论