资源简介
图片旋转,放大,缩小,拖动,合并,截图等,此版本中图片取自数据
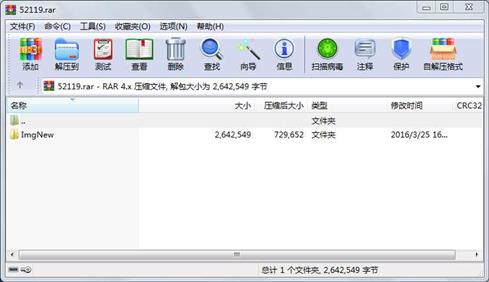
代码片段和文件信息
using System;
using System.Drawing;
using System.Drawing.Imaging;
using System.IO;
namespace ItcastCater
{
///
/// 图片处理
///
public class CImageLibrary
{
///
/// 检查图片返回的结果
///
public enum ValidateImageResult { OK InvalidFileSize InvalidImageSize }
///
/// 检查图片文件大小
///
/// 图片文件
/// 最大文件大小
/// 最大宽度
/// 最大高度
/// 返回检查结果
public static ValidateImageResult ValidateImage(string file int MAX_FILE_SIZE int MAX_WIDTH int MAX_HEIGHT)
{
byte[] bs = File.ReadAllBytes(file);
double size = (bs.Length / 1024);
//大于50KB
if (size > MAX_FILE_SIZE) return ValidateImageResult.InvalidFileSize;
Image img = null;
try
{
img = Image.FromFile(file);
if (img.Width > MAX_WIDTH || img.Height > MAX_HEIGHT)
return ValidateImageResult.InvalidImageSize;
else
return ValidateImageResult.OK;
}
finally
{
img.Dispose();
}
}
///
/// 按宽度比例缩小图片
///
/// 原始图片
/// 最大宽度
///
public static Image ResizeImage(Image imgSource int MAX_WIDTH int MAX_HEIGHT)
{
Image imgOutput = imgSource;
Size size = new Size(0 0); //用于存储按比例计算后的宽和高参数
if (imgSource.Width <= 3 || imgSource.Height <= 3) return imgSource; //3X3大小的图片不转换
//按宽度缩放图片
if (imgSource.Width > MAX_WIDTH) //计算宽度
{
double rate = MAX_WIDTH / (double)imgSource.Width; //计算宽度比例因子
size.Width = Convert.ToInt32(imgSource.Width * rate);
size.Height = Convert.ToInt32(imgSource.Height * rate);
imgOutput = imgSource.GetThumbnailImage(size.Width size.Height null IntPtr.Zero);
}
//按高度缩放图片
if (imgOutput.Height > MAX_HEIGHT)//计算高度
{
double rate = MAX_HEIGHT / (double)imgOutput.Height; //计算宽度比例因子
size.Width = Convert.ToInt32(imgOutput.Width * rate);
size.Height = Convert.ToInt32(imgOutput.Height * rate);
imgOutput = imgSource.GetThumbnailImage(size.Width size.Height null IntPtr.Zero);
}
return imgOutput;
}
///
/// 图片转换为数组
///
/// 图片文件
/// <
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 2569 2016-03-25 16:34 ImgNew\Img.sln
..A..H. 82944 2016-03-30 16:12 ImgNew\Img.suo
文件 157175 2016-03-02 09:27 ImgNew\pl\bin\Debug\FA20163292733.png
文件 83456 2016-03-28 17:21 ImgNew\pl\bin\Debug\ImageManage.exe
文件 91648 2016-03-28 17:21 ImgNew\pl\bin\Debug\ImageManage.pdb
文件 11600 2016-03-30 16:12 ImgNew\pl\bin\Debug\ImageManage.vshost.exe
文件 490 2014-01-13 21:31 ImgNew\pl\bin\Debug\ImageManage.vshost.exe.manifest
文件 87552 2016-02-26 16:43 ImgNew\pl\bin\Debug\pl.pdb
文件 490 2014-01-13 21:31 ImgNew\pl\bin\Debug\pl.vshost.exe.manifest
文件 7412 2016-02-24 09:02 ImgNew\pl\CImageLibrary.cs
文件 3559 2016-03-01 17:27 ImgNew\pl\DevTreelist.cs
文件 40842 2016-03-28 17:00 ImgNew\pl\FrmImage.cs
文件 34014 2016-03-28 15:36 ImgNew\pl\FrmImage.Designer.cs
文件 25330 2016-03-28 15:36 ImgNew\pl\FrmImage.resx
文件 24147 2016-02-26 14:21 ImgNew\pl\ImageClass.cs
文件 5596 2016-03-25 16:44 ImgNew\pl\ImageManege.csproj
文件 9198 2016-03-02 09:01 ImgNew\pl\ImageWatermark.cs
文件 78386 2016-03-28 08:40 ImgNew\pl\obj\x86\Debug\DesignTimeResolveAssemblyReferences.cache
文件 7178 2016-03-28 17:21 ImgNew\pl\obj\x86\Debug\DesignTimeResolveAssemblyReferencesInput.cache
文件 83456 2016-03-28 17:21 ImgNew\pl\obj\x86\Debug\ImageManage.exe
文件 578 2016-03-28 17:21 ImgNew\pl\obj\x86\Debug\imagemanage.exe.licenses
文件 91648 2016-03-28 17:21 ImgNew\pl\obj\x86\Debug\ImageManage.pdb
文件 1081 2016-03-30 16:12 ImgNew\pl\obj\x86\Debug\ImageManege.csproj.FileListAbsolute.txt
文件 978 2016-03-28 15:37 ImgNew\pl\obj\x86\Debug\ImageManege.csproj.GenerateResource.Cache
文件 93031 2016-03-28 14:01 ImgNew\pl\obj\x86\Debug\ImageManege.csprojResolveAssemblyReference.cache
文件 3445 2016-02-26 17:10 ImgNew\pl\obj\x86\Debug\pl.csproj.FileListAbsolute.txt
文件 1036 2016-02-26 13:19 ImgNew\pl\obj\x86\Debug\pl.csproj.GenerateResource.Cache
文件 75903 2016-02-26 16:41 ImgNew\pl\obj\x86\Debug\pl.csprojResolveAssemblyReference.cache
文件 569 2016-02-26 16:43 ImgNew\pl\obj\x86\Debug\pl.exe.licenses
文件 180 2016-03-28 14:01 ImgNew\pl\obj\x86\Debug\pl.Properties.Resources.resources
............此处省略105个文件信息
- 上一篇:C#课设--图书管理系统
- 下一篇:FiddlerCore抓包
相关资源
- C# 使用ListView控件实现图片浏览器(源
- c#向word文件插入图片
- C#实现在picturebox内画矩形,并将局部
- C#处理png图片位深度和交错属性
- C# 无损压缩图片
- svg批量转jpg|png
- TCP通信(支持发送文本和图片文件)
- 图片二进制读取数据库(附数据库)
- C#图片取点获取对应坐标参数工具
- 纯色背景透明化/替换色工具(C# 源码
- 图像图片灰度、反色、二值化、腐蚀
- C# 图片亮度对比度,自动纠偏
- c# 多线程传图片
- 动态移动图片
- winform 界面图片轮播效果
- panel内容打印(图片打印)
- MVC图片上传实例58438
- c#车牌识别系统附30张测试图片
- 安卓和asp.net通过webservice上传图片到服
- C#绘图和图片放大缩小等功能代码
- winform图片标尺,画矩形
- C# PDF转图片
- C#做的一个图片浏览器源码
- 大图找小图 C#图片对比高速找图源码
- c# 图像旋转 winform 窗体 图片转动
- SQLserver+C#实现的KTV点歌系统 有数据库
- C#在图片中写入文字
- 用c#将pdf文件转换成图片文件
- C#源码:批量自动去除图片白边
- WinForm 实现图片滚动
评论
共有 条评论