资源简介
窗口截图C#代码
可后台截取DX窗口
使用SharpDX库
GraphicsCapture Win32.DesktopDuplication
也可以抓取桌面
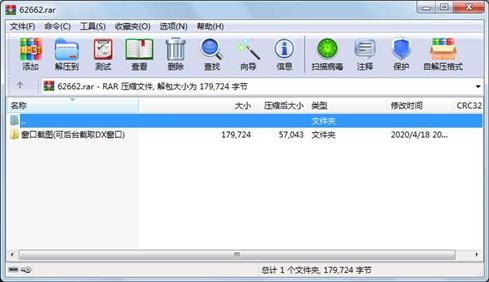
代码片段和文件信息
using System;
using System.Drawing;
using System.Drawing.Imaging;
using SharpDX;
using SharpDX.Direct3D11;
using SharpDX.DXGI;
using Win32.BitBlt.Interop;
using Win32.Shared;
using Win32.Shared.Interfaces;
using Device = SharpDX.Direct3D11.Device;
using Rectangle = System.Drawing.Rectangle;
namespace Win32.BitBlt
{
internal class BitBlt : ICaptureMethod
{
private IntPtr _hWnd;
public void Dispose()
{
// Nothing to do
}
public bool IsCapturing { get; private set; }
public void StartCapture(IntPtr hWnd Device device Factory factory)
{
var picker = new WindowPicker();
_hWnd = picker.PickCaptureTarget(hWnd);
if (_hWnd == IntPtr.Zero)
return;
IsCapturing = true;
}
public Texture2D TryGetNextframeAsTexture2D(Device device)
{
if (_hWnd == IntPtr.Zero)
return null;
var hdcSrc = NativeMethods.GetDCEx(_hWnd IntPtr.Zero DeviceContextValues.Window | DeviceContextValues.Cache | DeviceContextValues.LockWindowUpdate);
var hdcDest = NativeMethods.CreateCompatibleDC(hdcSrc);
NativeMethods.GetWindowRect(_hWnd out var rect);
var (width height) = (rect.Right - rect.Left rect.Bottom - rect.Top);
var hBitmap = NativeMethods.CreateCompatibleBitmap(hdcSrc width height);
var hOld = NativeMethods.Selectobject(hdcDest hBitmap);
NativeMethods.BitBlt(hdcDest 0 0 width height hdcSrc 0 0 TernaryRasterOperations.SRCCOPY);
NativeMethods.Selectobject(hdcDest hOld);
NativeMethods.DeleteDC(hdcDest);
NativeMethods.ReleaseDC(_hWnd hdcSrc);
using var img = Image.FromHbitmap(hBitmap);
NativeMethods.Deleteobject(hBitmap);
using var bitmap = img.Clone(Rectangle.FromLTRB(0 0 width height) PixelFormat.Format32bppArgb);
var bits = bitmap.LockBits(Rectangle.FromLTRB(0 0 width height) ImageLockMode.ReadOnly img.PixelFormat);
var data = new DataBox { DataPointer = bits.Scan0 RowPitch = bits.Width * 4 SlicePitch = bits.Height };
var texture2dDescription = new Texture2DDescription
{
ArraySize = 1
BindFlags = BindFlags.ShaderResource | BindFlags.RenderTarget
CpuAccessFlags = CpuAccessFlags.None
Format = Format.B8G8R8A8_UNorm
Height = height
MipLevels = 1
SampleDescription = new SampleDescription(1 0)
Usage = ResourceUsage.Default
Width = width
};
var texture2d = new Texture2D(device texture2dDescription new[] { data });
bitmap.UnlockBits(bits);
return texture2d;
}
public void StopCapture()
{
_hWnd = IntPtr.Zero;
}
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 5653 2020-04-18 20:37 窗口截图(可后台截取DX窗口)\Source\CSharp窗口截图.sln
....... 184 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\App.config
....... 3005 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\BitBlt.cs
....... 2872 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\Interop\DeviceContextValues.cs
....... 1460 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\Interop\NativeMethods.cs
....... 399 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\Interop\RECT.cs
....... 1711 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\Interop\TernaryRasterOperations.cs
文件 9696 2020-04-18 20:36 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\obj\Debug\Win32.BitBlt.csprojAssemblyReference.cache
....... 520 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\packages.config
....... 327 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\Program.cs
....... 457 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\Shader.fx
....... 4419 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.BitBlt\Win32.BitBlt.csproj
....... 184 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DesktopDuplication\App.config
....... 2208 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DesktopDuplication\DesktopDuplication.cs
文件 2924 2020-04-18 20:36 窗口截图(可后台截取DX窗口)\Source\Win32.DesktopDuplication\obj\Debug\Win32.DesktopDuplication.csprojAssemblyReference.cache
....... 284 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DesktopDuplication\packages.config
....... 347 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DesktopDuplication\Program.cs
....... 457 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DesktopDuplication\Shader.fx
....... 3242 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DesktopDuplication\Win32.DesktopDuplication.csproj
....... 184 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmSharedSurface\App.config
....... 2286 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmSharedSurface\DwmSharedSurface.cs
....... 994 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmSharedSurface\Interop\NativeMethods.cs
文件 8486 2020-04-18 20:36 窗口截图(可后台截取DX窗口)\Source\Win32.DwmSharedSurface\obj\Debug\Win32.DwmSharedSurface.csprojAssemblyReference.cache
....... 520 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmSharedSurface\packages.config
....... 347 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmSharedSurface\Program.cs
....... 457 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmSharedSurface\Shader.fx
....... 4273 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmSharedSurface\Win32.DwmSharedSurface.csproj
....... 184 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmThumbnail\App.config
....... 381 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmThumbnail\App.xaml
....... 340 2019-11-18 20:04 窗口截图(可后台截取DX窗口)\Source\Win32.DwmThumbnail\App.xaml.cs
............此处省略96个文件信息
- 上一篇:c# winform 串口电子秤集成开发
- 下一篇:command模式的撤销重做
相关资源
- C#百度地图源码
- Visual C#.2010从入门到精通配套源程序
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
- C#自定义控件
- 基于c#的实验室设备管理系统621530
- C# 使用ListView控件实现图片浏览器(源
- C#简单窗体聊天程序
- C#指纹识别系统程序 报告
- c# 高校档案信息管理系统
评论
共有 条评论