资源简介
本资源包括:
1. 最新版的WinIO(v3.0). WinIo32.dll, WinIo32.sys, WinIO64.dll, WinIO64.sys.
2. DumpPort.exe和DumpPhys.exe实例.
3. C#调用源码.
4. VB6调用源码.
5. Help文件,所有函数讲解.
此版支持64位系统, 但不再支持Win9x.
关于WinIO v3.0:
The WinIo library allows 32-bit and 64-bit Windows applications to directly access I/O ports and physical memory.
Version 3.0 offers the following features:
Supports 32-bit and 64-bit platforms (Itanium excluded).
WinIo can now be used by multiple applications concurrently.
New C# samples.
Bug fixes in this version:
Fixed a bug that prevented accessing physical memory above 2GB.
Fixed a bug that led to the WinIo driver getting uninstalled when calling ShutdownWinIo if the driver was set to load during boot with InstallWinIoDriver.
Note: Support for Windows 9x/ME has been removed. If you need to support these operating systems, please use WinIo v2.
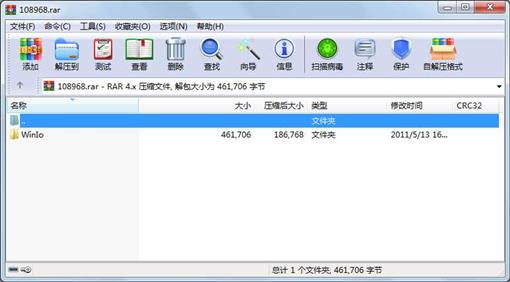
代码片段和文件信息
// ---------------------------------------------------- //
// WinIo v3.0 //
// Direct Hardware Access Under Windows //
// Copyright 1998-2010 Yariv Kaplan //
// http://www.internals.com //
// ---------------------------------------------------- //
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Runtime.InteropServices;
using System.Diagnostics;
namespace DumpPhys
{
public unsafe partial class Form1 : Form
{
[DllImport(“kernel32.dll“)]
private extern static IntPtr LoadLibrary(String DllName);
[DllImport(“kernel32.dll“)]
private extern static IntPtr GetProcAddress(IntPtr hModule String ProcName);
[DllImport(“kernel32“)]
private extern static bool FreeLibrary(IntPtr hModule);
[UnmanagedFunctionPointer(CallingConvention.StdCall)]
private delegate bool InitializeWinIoType();
[UnmanagedFunctionPointer(CallingConvention.StdCall)]
private delegate bool GetPhysLongType(IntPtr PhysAddr UInt32 *pPhysVal);
[UnmanagedFunctionPointer(CallingConvention.StdCall)]
private delegate bool SetPhysLongType(IntPtr PhysAddr UInt32 PhysVal);
[UnmanagedFunctionPointer(CallingConvention.StdCall)]
private delegate bool ShutdownWinIoType();
IntPtr hMod;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender EventArgs e)
{
// Check if this is a 32 bit or 64 bit system
if (IntPtr.Size == 4)
{
hMod = LoadLibrary(“WinIo32.dll“);
txtPhysAddr.MaxLength = 8;
txtPhysAddr.Text = “00000000“;
}
else if (IntPtr.Size == 8)
{
hMod = LoadLibrary(“WinIo64.dll“);
txtPhysAddr.MaxLength = 16;
txtPhysAddr.Text = “0000000000000000“;
}
if (hMod == IntPtr.Zero)
{
MessageBox.Show(“Can‘t find WinIo dll.\nMake sure the WinIo library files are located in the same directory as your executable file.“ “DumpPhys“ MessageBoxButtons.OK MessageBoxIcon.Error);
this.Close();
}
IntPtr pFunc = GetProcAddress(hMod “InitializeWinIo“);
if (pFunc != IntPtr.Zero)
{
InitializeWinIoType InitializeWinIo = (InitializeWinIoType)Marshal.GetDelegateForFunctionPointer(pFunc typeof(InitializeWinIoType));
bool Result = InitializeWinIo();
if (!Result)
{
MessageBox.Show(“Error returned from InitializeWinIo.\nMake su
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 13312 2010-05-15 22:54 WinIo\Binaries\DumpPhys.exe
文件 13312 2010-05-15 23:34 WinIo\Binaries\DumpPort.exe
文件 45568 2010-05-15 23:56 WinIo\Binaries\WinIo32.dll
文件 6656 2010-05-08 23:16 WinIo\Binaries\WinIo32.sys
文件 44544 2010-05-15 23:56 WinIo\Binaries\WinIo64.dll
文件 10920 2010-05-08 23:46 WinIo\Binaries\WinIo64.sys
文件 60620 2010-01-19 02:40 WinIo\Help\WinIo.chm
文件 5879 2010-05-16 00:08 WinIo\Samples\DumpPhys\DumpPhys\DumpPhys.csproj
文件 389 2009-07-23 02:24 WinIo\Samples\DumpPhys\DumpPhys\DumpPhys.manifest
文件 5801 2010-01-04 00:38 WinIo\Samples\DumpPhys\DumpPhys\Form1.cs
文件 7705 2010-01-03 22:51 WinIo\Samples\DumpPhys\DumpPhys\Form1.Designer.cs
文件 5814 2010-01-03 22:51 WinIo\Samples\DumpPhys\DumpPhys\Form1.resx
文件 475 2010-01-03 01:53 WinIo\Samples\DumpPhys\DumpPhys\Program.cs
文件 1293 2010-01-15 02:10 WinIo\Samples\DumpPhys\DumpPhys\Properties\AssemblyInfo.cs
文件 2843 2010-05-15 22:52 WinIo\Samples\DumpPhys\DumpPhys\Properties\Resources.Designer.cs
文件 5612 2010-01-02 23:16 WinIo\Samples\DumpPhys\DumpPhys\Properties\Resources.resx
文件 1089 2010-05-15 22:52 WinIo\Samples\DumpPhys\DumpPhys\Properties\Settings.Designer.cs
文件 249 2010-01-02 23:16 WinIo\Samples\DumpPhys\DumpPhys\Properties\Settings.settings
文件 1268 2010-05-16 00:08 WinIo\Samples\DumpPhys\DumpPhys.sln
..A..H. 24576 2010-05-16 00:09 WinIo\Samples\DumpPhys\DumpPhys.suo
文件 5879 2010-05-16 00:09 WinIo\Samples\DumpPort\DumpPort\DumpPort.csproj
文件 389 2009-07-23 02:24 WinIo\Samples\DumpPort\DumpPort\DumpPort.manifest
文件 5581 2010-01-04 00:38 WinIo\Samples\DumpPort\DumpPort\Form1.cs
文件 7787 2010-01-04 00:33 WinIo\Samples\DumpPort\DumpPort\Form1.Designer.cs
文件 5814 2010-01-04 00:33 WinIo\Samples\DumpPort\DumpPort\Form1.resx
文件 475 2010-01-03 23:02 WinIo\Samples\DumpPort\DumpPort\Program.cs
文件 1293 2010-01-15 01:30 WinIo\Samples\DumpPort\DumpPort\Properties\AssemblyInfo.cs
文件 2843 2010-05-15 22:54 WinIo\Samples\DumpPort\DumpPort\Properties\Resources.Designer.cs
文件 5612 2010-01-03 23:02 WinIo\Samples\DumpPort\DumpPort\Properties\Resources.resx
文件 1089 2010-05-15 22:54 WinIo\Samples\DumpPort\DumpPort\Properties\Settings.Designer.cs
............此处省略43个文件信息
- 上一篇:C#封装的JqGrid插件
- 下一篇:人事管理系统SQL版
相关资源
- asp.net C#购物车源代码
- C#实时网络流量监听源码
- C#百度地图源码
- Visual C#.2010从入门到精通配套源程序
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
- C#自定义控件
- 基于c#的实验室设备管理系统621530
- C# 使用ListView控件实现图片浏览器(源
- C#简单窗体聊天程序
评论
共有 条评论