-
大小: 53KB文件类型: .zip金币: 2下载: 0 次发布日期: 2021-05-29
- 语言: C#
- 标签: c# serialport sample
资源简介
http://blog.csdn.net/wuyazhe/archive/2010/05/17/5598945.aspx
博客文章的配套代码。希望能帮助到你。
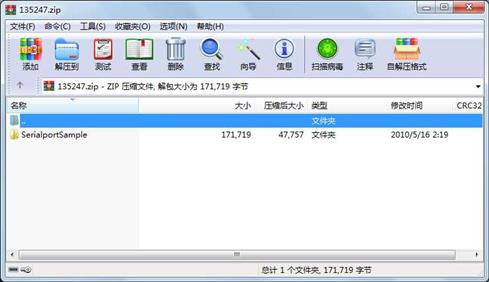
代码片段和文件信息
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO.Ports;
using System.Text.Regularexpressions;
namespace SerialportSample
{
public partial class SerialportSampleForm : Form
{
private SerialPort comm = new SerialPort();
private StringBuilder builder = new StringBuilder();//避免在事件处理方法中反复的创建,定义到外面。
private long received_count = 0;//接收计数
private long send_count = 0;//发送计数
public SerialportSampleForm()
{
InitializeComponent();
}
//窗体初始化
private void Form1_Load(object sender EventArgs e)
{
//初始化下拉串口名称列表框
string[] ports = SerialPort.GetPortNames();
Array.Sort(ports);
comboPortName.Items.AddRange(ports);
comboPortName.SelectedIndex = comboPortName.Items.Count > 0 ? 0 : -1;
comboBaudrate.SelectedIndex = comboBaudrate.Items.IndexOf(“9600“);
//初始化SerialPort对象
comm.NewLine = “\r\n“;
comm.RtsEnable = true;//根据实际情况吧。
//添加事件注册
comm.DataReceived += comm_DataReceived;
}
void comm_DataReceived(object sender SerialDataReceivedEventArgs e)
{
int n = comm.BytesToRead;//先记录下来,避免某种原因,人为的原因,操作几次之间时间长,缓存不一致
byte[] buf = new byte[n];//声明一个临时数组存储当前来的串口数据
received_count += n;//增加接收计数
comm.Read(buf 0 n);//读取缓冲数据
builder.Clear();//清除字符串构造器的内容
//因为要访问ui资源,所以需要使用invoke方式同步ui。
this.Invoke((EventHandler)(delegate
{
//判断是否是显示为16禁止
if (checkBoxHexView.Checked)
{
//依次的拼接出16进制字符串
foreach (byte b in buf)
{
builder.Append(b.ToString(“X2“) + “ “);
}
}
else
{
//直接按ASCII规则转换成字符串
builder.Append(Encoding.ASCII.GetString(buf));
}
//追加的形式添加到文本框末端,并滚动到最后。
this.txGet.AppendText(builder.ToString());
//修改接收计数
labelGetCount.Text = “Get:“ + received_count.ToString();
}));
}
private void buttonOpenClose_Click(object sender EventArgs e)
{
//根据当前串口对象,来判断操作
if (comm.IsOpen)
{
//打开时点击,则关闭串口
comm.Close();
}
else
{
//关闭时点击,则设置好端口,波特率后打开
comm.PortName = comboPortName.Text;
comm.BaudRate = int.Parse(comboBaudrate.Text);
try
{
comm.Open();
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2010-05-16 02:19 SerialportSample\
目录 0 2010-05-16 02:19 SerialportSample\SerialportSample\
文件 890 2010-05-16 02:19 SerialportSample\SerialportSample.sln
文件 19456 2010-05-17 01:20 SerialportSample\SerialportSample.suo
目录 0 2010-05-16 02:19 SerialportSample\SerialportSample\bin\
目录 0 2010-05-16 02:19 SerialportSample\SerialportSample\bin\Debug\
文件 15872 2010-05-17 01:19 SerialportSample\SerialportSample\bin\Debug\SerialportSample.exe
文件 26112 2010-05-17 01:19 SerialportSample\SerialportSample\bin\Debug\SerialportSample.pdb
文件 11600 2010-05-17 01:00 SerialportSample\SerialportSample\bin\Debug\SerialportSample.vshost.exe
文件 490 2010-03-17 22:39 SerialportSample\SerialportSample\bin\Debug\SerialportSample.vshost.exe.manifest
文件 6110 2010-05-17 01:19 SerialportSample\SerialportSample\Form1.cs
文件 15770 2010-05-17 01:06 SerialportSample\SerialportSample\Form1.Designer.cs
文件 5817 2010-05-17 01:06 SerialportSample\SerialportSample\Form1.resx
目录 0 2010-05-16 02:19 SerialportSample\SerialportSample\obj\
目录 0 2010-05-16 02:19 SerialportSample\SerialportSample\obj\x86\
目录 0 2010-05-16 02:19 SerialportSample\SerialportSample\obj\x86\Debug\
文件 3408 2010-05-17 00:44 SerialportSample\SerialportSample\obj\x86\Debug\DesignTimeResolveAssemblyReferences.cache
文件 6277 2010-05-17 01:19 SerialportSample\SerialportSample\obj\x86\Debug\DesignTimeResolveAssemblyReferencesInput.cache
文件 324 2010-05-17 01:19 SerialportSample\SerialportSample\obj\x86\Debug\GenerateResource.read.1.tlog
文件 812 2010-05-17 01:19 SerialportSample\SerialportSample\obj\x86\Debug\GenerateResource.write.1.tlog
文件 937 2010-05-17 01:19 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.csproj.FileListAbsolute.txt
文件 15872 2010-05-17 01:19 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.exe
文件 26112 2010-05-17 01:19 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.pdb
文件 180 2010-05-17 01:19 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.Properties.Resources.resources
文件 180 2010-05-17 01:19 SerialportSample\SerialportSample\obj\x86\Debug\SerialportSample.SerialportSampleForm.resources
目录 0 2010-05-16 02:19 SerialportSample\SerialportSample\obj\x86\Debug\TempPE\
文件 523 2010-05-16 03:24 SerialportSample\SerialportSample\Program.cs
目录 0 2010-05-16 02:19 SerialportSample\SerialportSample\Properties\
文件 1468 2010-05-16 02:19 SerialportSample\SerialportSample\Properties\AssemblyInfo.cs
文件 2858 2010-05-16 02:19 SerialportSample\SerialportSample\Properties\Resources.Designer.cs
文件 5612 2010-05-16 02:19 SerialportSample\SerialportSample\Properties\Resources.resx
............此处省略3个文件信息
- 上一篇:天猫推荐测试数据
- 下一篇:C# Ftp客户端源码
相关资源
- C# OCR数字识别实例,采用TessnetOcr,对
- 考试管理系统 - C#源码
- asp.net C#购物车源代码
- C#实时网络流量监听源码
- C#百度地图源码
- Visual C#.2010从入门到精通配套源程序
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
- C#自定义控件
- 基于c#的实验室设备管理系统621530
评论
共有 条评论