资源简介
使用WPF实现了流程图的绘制,初学WPF的建议参考一下,可以在此基础上改一改。
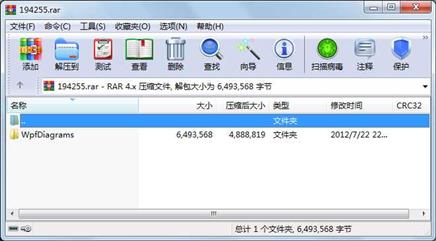
代码片段和文件信息
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Input;
namespace Aga.Diagrams
{
public static class AutoScrollDecorator
{
public static readonly DependencyProperty IsEnabledProperty =
DependencyProperty.RegisterAttached(
“IsEnabled“
typeof(bool)
typeof(AutoScrollDecorator) new Propertymetadata(false IsEnabledValueChanged));
public static void SetIsEnabled(Dependencyobject element bool value)
{
element.SetValue(IsEnabledProperty value);
}
public static bool GetIsEnabled(Dependencyobject element)
{
return (bool)element.GetValue(IsEnabledProperty);
}
public static readonly DependencyProperty SensivityProperty =
DependencyProperty.RegisterAttached(
“Sensivity“
typeof(double)
typeof(AutoScrollDecorator) new Propertymetadata(20.0));
public static void SetSensivity(Dependencyobject element double value)
{
element.SetValue(SensivityProperty value);
}
public static double GetSensivity(Dependencyobject element)
{
return (double)element.GetValue(SensivityProperty);
}
public static readonly DependencyProperty StepProperty =
DependencyProperty.RegisterAttached(
“Step“
typeof(double)
typeof(AutoScrollDecorator) new Propertymetadata(16.0));
public static void SetStep(Dependencyobject element double value)
{
element.SetValue(StepProperty value);
}
public static double GetStep(Dependencyobject element)
{
return (double)element.GetValue(StepProperty);
}
static void IsEnabledValueChanged(Dependencyobject depObj DependencyPropertyChangedEventArgs e)
{
var view = depObj as ScrollViewer;
if (view != null && e.NewValue is bool)
{
if ((bool)e.NewValue)
view.PreviewMouseMove += ViewPreviewMouseMove;
else
view.PreviewMouseMove -= ViewPreviewMouseMove;
}
}
private static void ViewPreviewMouseMove(object sender MouseEventArgs e)
{
var _scrollView = sender as ScrollViewer;
if (_scrollView == null || !(_scrollView.Content is Dependencyobject))
return;
if (!GetIsEnabled((Dependencyobject)_scrollView.Content))
return;
var point = e.GetPosition(_scrollView);
double sensivity = GetSensivity(_scrollView);
double step = GetStep(_scrollView);
double dx = 0;
double dy = 0;
if (point.X < sensivity)
dx = -step;
else if (point.X > _scrollView.ActualWidth - sensivity)
dx = +step;
if (point.Y < sensivity)
dy = -step;
else if (point.Y > _scrollView.ActualHeight - sensivity)
dy = +step;
_scrollView.ScrollToHorizontalOffset(_scrollView.HorizontalOffset + dx);
_scrollView.ScrollToVerticalOffset(_scrollView.VerticalOffset + dy);
}
}
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 1459 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Adorners\DragAdorner.cs
文件 1242 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Adorners\li
文件 667 2009-08-28 22:08 WpfDiagrams\Aga.Diagrams\Adorners\MoveResizeAdorner.cs
文件 973 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Adorners\RubberbandAdorner.cs
文件 941 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Adorners\SelectionAdorner.cs
文件 8998 2011-04-22 20:37 WpfDiagrams\Aga.Diagrams\Aga.Diagrams.csproj
文件 452 2011-04-22 20:37 WpfDiagrams\Aga.Diagrams\Aga.Diagrams.csproj.user
文件 2921 2009-07-31 04:39 WpfDiagrams\Aga.Diagrams\AutoScrollDecorator.cs
文件 64000 2012-07-22 21:47 WpfDiagrams\Aga.Diagrams\bin\Debug\Aga.Diagrams.dll
文件 192000 2012-07-22 21:47 WpfDiagrams\Aga.Diagrams\bin\Debug\Aga.Diagrams.pdb
文件 2949 2009-10-16 20:41 WpfDiagrams\Aga.Diagrams\Controls\ClassDiagram.cd
文件 3244 2009-10-16 20:41 WpfDiagrams\Aga.Diagrams\Controls\DiagramItem.cs
文件 910 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Controls\DragThumb.cs
文件 373 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Controls\DragThumbKinds.cs
文件 346 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Controls\li
文件 4285 2009-10-16 20:41 WpfDiagrams\Aga.Diagrams\Controls\li
文件 802 2009-10-16 22:47 WpfDiagrams\Aga.Diagrams\Controls\li
文件 188 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Controls\li
文件 412 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Controls\li
文件 2605 2009-10-19 22:40 WpfDiagrams\Aga.Diagrams\Controls\li
文件 227 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\Controls\Node\INode.cs
文件 2087 2009-10-19 22:40 WpfDiagrams\Aga.Diagrams\Controls\Node\Node.cs
文件 884 2009-10-19 22:40 WpfDiagrams\Aga.Diagrams\Controls\Ports\EllipsePort.cs
文件 346 2009-10-16 20:41 WpfDiagrams\Aga.Diagrams\Controls\Ports\IPort.cs
文件 3840 2009-10-19 22:40 WpfDiagrams\Aga.Diagrams\Controls\Ports\Portba
文件 864 2009-10-19 22:40 WpfDiagrams\Aga.Diagrams\Controls\Ports\RectPort.cs
文件 416 2009-09-15 21:11 WpfDiagrams\Aga.Diagrams\Controls\Selectionfr
文件 1859 2009-10-01 20:42 WpfDiagrams\Aga.Diagrams\DiagramScrollView.cs
文件 7652 2009-10-19 22:40 WpfDiagrams\Aga.Diagrams\DiagramView.cs
文件 1379 2009-10-01 20:12 WpfDiagrams\Aga.Diagrams\GlobalSuppressions.cs
............此处省略148个文件信息
- 上一篇:C# windows 服务学习
- 下一篇:Winform通过NPOI导出excel
相关资源
- 用WPF开发的多人聊天室 语言C#
- WPF控件库HandyControl
- WPF
- c# wpf实现的上位机
- VisionPro控件在WPF 应用
- WPF使用MVVM
- winform实现饼状图、柱状图、折线图(
- C#中WPF联合Halcon的一个学习(解决内存
- WPF CEFSHARP 支持 MP4
- WPF贝塞尔曲线
- WPF 简单控件集
- WPF鼠标拖动控件源码
- wpf开发教程
- AduMusic迷你音乐盒WPF源码
- WPF PDF封装(放大、缩小、单页、双页
- C# .NET5.0(net core)基于WPF(XAML)开发
- WPF MVVM 基础入门
- wpf echart
- windorm 加载WPF控件 ,实现dxf文件显示
- WPF Control Development
- wpf Dock window
- WPF 隐蔽查看股票行情工具
- WPF控件库(HandyControl)
- 别踩白块wpf 源码
- WPF贪吃蛇
- WPF Task 多任务
- WPF path动画
- WPF 最基础的组件拖动、改变大小
- WPF DATAGRID 数据绑定
- WPF绘制坐标系(可放大缩小)
评论
共有 条评论