资源简介
C#UDP通信+文件传输 源码
京华志&精华志出品 希望大家互相学习,互相进步 支持CSDN 支持微软
主要包括C# ASP.NET SQLDBA 源码 毕业设计 开题报告 答辩PPT等
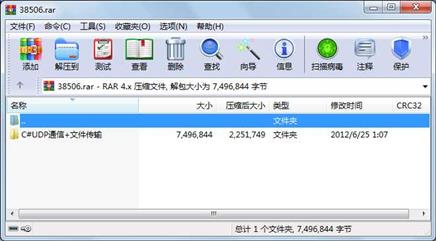
代码片段和文件信息
using System;
using System.Collections.Generic;
using System.Text;
using System.Net.Sockets;
using System.Threading;
using System.IO;
namespace BroadcastExample
{
public class ConnectionClient
{
Socket sokMsg;
DGShowMsg dgShowMsg;//负责 向主窗体文本框显示消息的方法委托
DGShowMsg dgRemoveConnection;// 负责 从主窗体 中移除 当前连接
Thread threadMsg;
#region 构造函数
///
///
///
/// 通信套接字
/// 向主窗体文本框显示消息的方法委托
public ConnectionClient(Socket sokMsg DGShowMsg dgShowMsg DGShowMsg dgRemoveConnection)
{
this.sokMsg = sokMsg;
this.dgShowMsg = dgShowMsg;
this.dgRemoveConnection = dgRemoveConnection;
this.threadMsg = new Thread(RecMsg);
this.threadMsg.IsBackground = true;
this.threadMsg.Start();
}
#endregion
bool isRec = true;
#region 02负责监听客户端发送来的消息
void RecMsg()
{
while (isRec)
{
try
{
byte[] arrMsg = new byte[1024 * 1024 * 2];
//接收 对应 客户端发来的消息
int length = sokMsg.Receive(arrMsg);
//将接收到的消息数组里真实消息转成字符串
string strMsg = System.Text.Encoding.UTF8.GetString(arrMsg 0 length);
//通过委托 显示消息到 窗体的文本框
dgShowMsg(strMsg);
}
catch (Exception ex)
{
isRec = false;
//从主窗体中 移除 下拉框中对应的客户端选择项,同时 移除 集合中对应的 ConnectionClient对象
dgRemoveConnection(sokMsg.RemoteEndPoint.ToString());
}
}
}
#endregion
#region 03向客户端发送消息
///
/// 向客户端发送消息
///
///
public void Send(string strMsg)
{
byte[] arrMsg = System.Text.Encoding.UTF8.GetBytes(strMsg);
byte[] arrMsgFinal = new byte[arrMsg.Length + 1];
arrMsgFinal[0] = 0;//设置 数据标识位等于0,代表 发送的是 文字
arrMsg.CopyTo(arrMsgFinal 1);
sokMsg.Send(arrMsgFinal);
}
#endregion
#region 04向客户端发送文件数据 +void SendFile(string strPath)
///
/// 04向客户端发送文件数据
///
/// 文件路径
public void SendFile(string strPath)
{
//通过文件流 读取文件内容
using (FileStream fs = new FileStream(strPath FileMode.OpenOrCreate))
{
byte[] arrFile = new byte[1024 * 1024 * 2];
//读取文件内容到字节数组,并 获得 实际文件大小
int length = fs.Read(arrFile 0 arrFile.Length);
//定义一个 新数组,长度为文件实际长度 +1
byte[] arrFileFina
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 59136 2012-06-25 01:07 C#UDP通信+文件传输\2000多商业程序 OA 程序下载 版权说明.txt
文件 59136 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\2000多商业程序 OA 程序下载 版权说明.txt
文件 17920 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\bin\Debug\BroadcastExample.exe
文件 42496 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\bin\Debug\BroadcastExample.pdb
文件 14328 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\bin\Debug\BroadcastExample.vshost.exe
文件 490 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\bin\Debug\BroadcastExample.vshost.exe.manifest
文件 3534 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\BroadcastExample.csproj
文件 4623 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\ConnectionClient.cs
文件 161 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\DGShowMsg.cs
文件 18495 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\Form1.cs
文件 6662 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\Form1.Designer.cs
文件 5814 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\Form1.resx
文件 1502 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\obj\BroadcastExample.csproj.FileListAbsolute.txt
文件 2254 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\obj\Debug\BroadcastExample.csproj.FileListAbsolute.txt
文件 847 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\obj\Debug\BroadcastExample.csproj.GenerateResource.Cache
文件 17920 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\obj\Debug\BroadcastExample.exe
文件 180 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\obj\Debug\BroadcastExample.Form1.resources
文件 42496 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\obj\Debug\BroadcastExample.pdb
文件 180 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\obj\Debug\BroadcastExample.Properties.Resources.resources
文件 4608 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\obj\Debug\TempPE\Properties.Resources.Designer.cs.dll
文件 491 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\Program.cs
文件 1204 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\Properties\AssemblyInfo.cs
文件 2856 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\Properties\Resources.Designer.cs
文件 5612 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\Properties\Resources.resx
文件 1114 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\Properties\Settings.Designer.cs
文件 249 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample\Properties\Settings.settings
文件 938 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample.sln
..A..H. 18944 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\BroadcastExample.suo
文件 3543552 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\DevComponents.DotNetBar2.dll
文件 1189 2012-06-25 01:07 C#UDP通信+文件传输\BroadcastExample\UpgradeLog.xm
............此处省略21个文件信息
- 上一篇:C#支付宝、微信扫码支付类库
- 下一篇:C#版KTV点歌系统源代码
相关资源
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 020ASP.NET车辆综合管理系统.zip
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
- C#自定义控件
- 基于c#的实验室设备管理系统621530
- C# 使用ListView控件实现图片浏览器(源
- C#简单窗体聊天程序
- C#指纹识别系统程序 报告
- c# 高校档案信息管理系统
- c#向word文件插入图片
评论
共有 条评论