资源简介
Visual C# and Databases is a tutorial that provides a detailed introduction to using Visual C# for accessing and maintaining databases. Topics covered include: database structure, database design, Visual C# project building, ADO .NET data objects, data bound controls, proper interface design, structured query language (SQL), and database reports.
Visual C# and Databases is presented using a combination of over 700 pages of course notes and actual Visual C# examples. No previous experience working with databases is presumed. It is assumed, however, that users of the course are familiar with the Visual C# environment and the steps involved in building a Visual C# application.
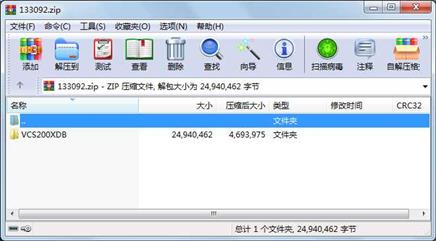
代码片段和文件信息
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace Example_1_1
{
public partial class frmMailingList : Form
{
public frmMailingList()
{
InitializeComponent();
}
DateTime lastNow;
TimeSpan elapsedTime;
private void btnStart_Click(object sender EventArgs e)
{
// Start button clicked
// Disable start and exit buttons
// Enabled pause button
btnStart.Enabled = false;
btnExit.Enabled = false;
btnPause.Enabled = true;
// Establish start time and start timer control
lastNow = DateTime.Now;
timSeconds.Enabled = true;
// Enable mailing list frame
grpMail.Enabled = true;
txtName.Focus();
}
private void btnPause_Click(object sender EventArgs e)
{
// Pause button clicked
// Disable pause button
// Enabled start and exit buttons
btnPause.Enabled = false;
btnStart.Enabled = true;
btnExit.Enabled = true;
// Stop timer
timSeconds.Enabled = false;
// Disable editing frame
grpMail.Enabled = false;
}
private void btnExit_Click(object sender EventArgs e)
{
this.Close();
}
private void timSeconds_Tick(object sender EventArgs e)
{
// Compute elapsed time and display
elapsedTime += DateTime.Now - lastNow;
txtElapsedTime.Text = Convert.ToString(new TimeSpan(elapsedTime.Hours elapsedTime.Minutes elapsedTime.Seconds));
lastNow = DateTime.Now;
}
private void txtInput_KeyPress(object sender KeyPressEventArgs e)
{
String boxName = ((TextBox) sender).Name;
// Check for return key
if ((int) e.KeyChar == 13)
{
switch (boxName)
{
case “txtName“:
txtAddress.Focus();
break;
case “txtAddress“:
txtCity.Focus();
break;
case “txtCity“:
txtState.Focus();
break;
case “txtState“:
txtZip.Focus();
break;
case “txtZip“:
btnAccept.Focus();
break;
}
}
// In zip text box make sure only numbers or backspace pressed
if (boxName.Equals(“txtZip“))
{
if ((e.KeyChar >= ‘0‘ && e.KeyChar <= ‘9‘) || (int) e.KeyChar == 8)
{
e.Handled = false;
}
else
{
e.Handled = true;
}
}
}
private void btnAccept_Click(object sender EventArgs e)
{
string s;
// Accept button clicked - form label and output in message box
// Make sure each text box has entry
if (txtName.Text.Equals(““) || txtAddress.Text.Equals(““) || txtCity.Text.Equals(““) || txtState.Text.Equals(““) || txtZip.Text.Equals
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2008-06-12 03:23 VCS200XDB\Code\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 1\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 1\Example 1-1\
文件 3660 2006-12-28 23:12 VCS200XDB\Code\Class 1\Example 1-1\Example 1-1.cs
文件 3296 2006-12-28 23:11 VCS200XDB\Code\Class 1\Example 1-1\Example 1-1.csproj
文件 11402 2006-12-28 23:12 VCS200XDB\Code\Class 1\Example 1-1\Example 1-1.Designer.cs
文件 6186 2006-12-28 09:16 VCS200XDB\Code\Class 1\Example 1-1\Example 1-1.resx
文件 910 2006-12-28 04:47 VCS200XDB\Code\Class 1\Example 1-1\Example 1-1.sln
文件 12288 2008-03-03 20:54 VCS200XDB\Code\Class 1\Example 1-1\Example 1-1.suo
文件 435 2006-12-28 23:12 VCS200XDB\Code\Class 1\Example 1-1\Program.cs
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 1\Example 1-1\Properties\
文件 1270 2006-12-28 23:11 VCS200XDB\Code\Class 1\Example 1-1\Properties\AssemblyInfo.cs
文件 2847 2006-12-28 23:11 VCS200XDB\Code\Class 1\Example 1-1\Properties\Resources.Designer.cs
文件 5612 2006-12-28 04:15 VCS200XDB\Code\Class 1\Example 1-1\Properties\Resources.resx
文件 1090 2006-12-28 23:11 VCS200XDB\Code\Class 1\Example 1-1\Properties\Settings.Designer.cs
文件 249 2006-12-28 04:15 VCS200XDB\Code\Class 1\Example 1-1\Properties\Settings.settings
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 10\
目录 0 2008-06-13 20:19 VCS200XDB\Code\Class 10\Example 10-1\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 10\Example 10-1\Properties\
目录 0 2008-06-13 20:20 VCS200XDB\Code\Class 10\Example 10-2\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 10\Example 10-2\Inventory Photos\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 10\Example 10-2\Properties\
目录 0 2008-06-13 19:57 VCS200XDB\Code\Class 10\Example 10-3\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 10\Example 10-3\HelpFile\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 10\Example 10-3\Properties\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 10\Setup 10-3\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 10\Setup 10-3\Debug\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 10\Setup 10-3\Release\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 11\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 11\Example 11-1\
目录 0 2008-06-12 03:22 VCS200XDB\Code\Class 11\Example 11-1\Properties\
............此处省略400个文件信息
- 上一篇:C# 图像处理
- 下一篇:asp.net校园二手交易中心
相关资源
- C# OCR数字识别实例,采用TessnetOcr,对
- 考试管理系统 - C#源码
- asp.net C#购物车源代码
- C#实时网络流量监听源码
- C#百度地图源码
- Visual C#.2010从入门到精通配套源程序
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
- C#自定义控件
- 基于c#的实验室设备管理系统621530
评论
共有 条评论