资源简介
winform多线程与窗体数据交互 C# winform thread
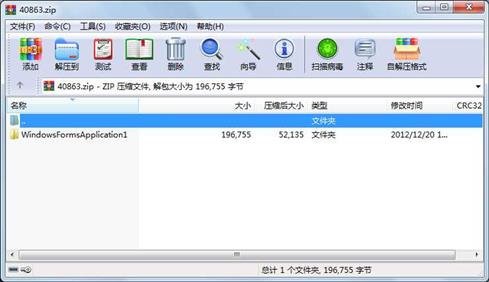
代码片段和文件信息
using System;
using System.Threading;
using System.ComponentModel;
namespace WindowsFormsApplication1
{
public interface IAsyncEventArgs
{
int action { get; }
object[] getobjects { get; }
}
///
/// 线程事件参数
///
internal class AsyncEventArgs : EventArgs IAsyncEventArgs
{
private readonly int m_action;
private readonly object[] m_objects;
public int action
{
get { return this.m_action; }
}
public object[] getobjects
{
get { return this.m_objects; }
}
public AsyncEventArgs(int action object[] objs)
{
this.m_action = action;
this.m_objects = objs;
}
}
///
/// 异步操作基类基类不需要修改,任务应该在子类中完成
/// 注意:只适用用winform开发
///
public abstract class Asyncbase
{
private Thread m_thread;
private readonly ISynchronizeInvoke m_Target;
private bool m_IsStop = false;
private bool[] m_isCancelFlag = new bool[]{falsefalse};
protected int m_action = 0;
public event EventHandler TaskBefore;
public event EventHandler TaskProgress;
public event EventHandler TaskDone;
public event EventHandler Complete;
public event EventHandler Error;
//开始执行任务之前
private void OnTaskBefore(object sender)
{
toTarget(TaskBefore new object[] { sender new AsyncEventArgs(this.m_action null) });
}
//执行任务进度
protected void OnTaskProgress(object sender int step)
{
toTarget(TaskProgress new object[] { sender new AsyncEventArgs(this.m_action new object[] { step }) });
}
//执行任务成功完成(只有任务成功才会执行,取消和异常均不会执行),传递给主线程的对象在这里设置
protected void OnTaskDone(object sender object[] objs)
{
toTarget(TaskDone new object[] { sender new AsyncEventArgs(this.m_action objs) });
}
//执行任务时发生异常
private void onerror(object sender Exception exp)
{
toTarget(Error new object[] { sender new AsyncEventArgs(this.m_action new object[] { exp }) });
}
//完成一个任务,无论如果都会执行
private void OnComplete(object sender)
{
toTarget(Complete new object[] { sender new AsyncEventArgs(this.m_action null) });
}
///
/// 构造方法
///
/// 调用目标
protected Asyncbase(ISynchronizeInvoke target)
{
this.m_Target = target;
this.m_thread = null;
}
~Asyncbase()
{
Stop();
}
//启动一个工作线程
public void Start()
{
try
{
this.m_thread = new Thread(new ThreadStart(Work));
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2012-12-20 13:48 WindowsFormsApplication1\
目录 0 2012-12-20 13:48 WindowsFormsApplication1\WindowsFormsApplication1\
文件 914 2012-12-20 10:45 WindowsFormsApplication1\WindowsFormsApplication1.sln
文件 24064 2012-12-20 13:47 WindowsFormsApplication1\WindowsFormsApplication1.suo
文件 6962 2012-12-20 13:37 WindowsFormsApplication1\WindowsFormsApplication1\Async.cs
文件 2383 2012-12-20 13:45 WindowsFormsApplication1\WindowsFormsApplication1\AsyncImport.cs
目录 0 2012-12-20 13:48 WindowsFormsApplication1\WindowsFormsApplication1\bin\
目录 0 2012-12-20 13:48 WindowsFormsApplication1\WindowsFormsApplication1\bin\Debug\
文件 14848 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\bin\Debug\WindowsFormsApplication1.exe
文件 38400 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\bin\Debug\WindowsFormsApplication1.pdb
文件 11608 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\bin\Debug\WindowsFormsApplication1.vshost.exe
文件 490 2010-03-17 22:39 WindowsFormsApplication1\WindowsFormsApplication1\bin\Debug\WindowsFormsApplication1.vshost.exe.manifest
文件 6008 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\Form1.cs
文件 4788 2012-12-20 13:45 WindowsFormsApplication1\WindowsFormsApplication1\Form1.Designer.cs
文件 5817 2012-12-20 11:24 WindowsFormsApplication1\WindowsFormsApplication1\Form1.resx
目录 0 2012-12-20 13:48 WindowsFormsApplication1\WindowsFormsApplication1\obj\
目录 0 2012-12-20 13:48 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\
目录 0 2012-12-20 13:48 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\
文件 6256 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\DesignTimeResolveAssemblyReferencesInput.cache
文件 686 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\GenerateResource-ResGen.read.1.tlog
文件 1222 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\GenerateResource-ResGen.write.1.tlog
目录 0 2012-12-20 10:45 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\TempPE\
文件 1443 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\WindowsFormsApplication1.csproj.FileListAbsolute.txt
文件 14848 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\WindowsFormsApplication1.exe
文件 180 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\WindowsFormsApplication1.Form1.resources
文件 38400 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\WindowsFormsApplication1.pdb
文件 180 2012-12-20 13:46 WindowsFormsApplication1\WindowsFormsApplication1\obj\x86\Debug\WindowsFormsApplication1.Properties.Resources.resources
文件 505 2012-12-20 10:45 WindowsFormsApplication1\WindowsFormsApplication1\Program.cs
目录 0 2012-12-20 13:48 WindowsFormsApplication1\WindowsFormsApplication1\Properties\
文件 1380 2012-12-20 10:45 WindowsFormsApplication1\WindowsFormsApplication1\Properties\AssemblyInfo.cs
文件 2896 2012-12-20 10:45 WindowsFormsApplication1\WindowsFormsApplication1\Properties\Resources.Designer.cs
............此处省略5个文件信息
相关资源
- Winform可视化打印模板设计工具含源码
- C# 软件版本更新
- C#屏幕软键盘源码,可以自己定制界面
- 智慧城市 智能家居 C# 源代码
- c#获取mobile手机的IMEI和IMSI
- C#实现简单QQ聊天程序
- 操作系统 模拟的 欢迎下载 C#版
- C#写的计算机性能监控程序
- 用C#实现邮件发送,有点类似于outlo
- MVC model层代码生成器 C#
- c#小型图书销售系统
- C# Socket Server Client 通讯应用 完整的服
- c# winform 自动登录 百度账户 源代码
- C#编写的16进制计算器
- C#TCP通信协议
- C# 数据表(Dataset)操作 合并 查询一
- C#语音识别系统speechsdk51,SpeechSDK51L
- 数据库备份还原工具1.0 C# 源码
-
[免费]xm
lDocument 节点遍历C# - EQ2008LEDc#开发实例
- DirectX.Capturec# winform 操作摄像头录像附
- c# 实现的最大最小距离方法对鸢尾花
- C#版保龄球记分代码
- C#自定义控件
- 基于c#的实验室设备管理系统621530
- C# 使用ListView控件实现图片浏览器(源
- C#简单窗体聊天程序
- C#指纹识别系统程序 报告
- c# 高校档案信息管理系统
- c#向word文件插入图片
评论
共有 条评论