资源简介
maven工程,解压导入eclipse中,直接maven install 到maven本地仓库
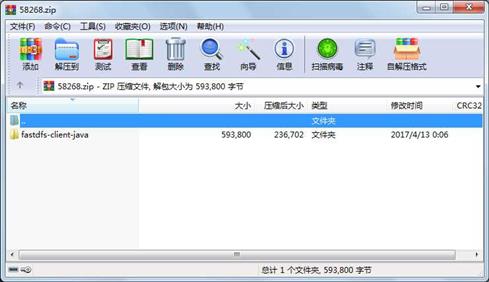
代码片段和文件信息
package org.csource.common;
import java.io.IOException;
/**
* Freeware from:
* Roedy Green
* Canadian Mind Products
* #327 - 964 Heywood Avenue
* Victoria BC Canada V8V 2Y5
* tel:(250) 361-9093
* mailto:roedy@mindprod.com
*/
/**
* Encode arbitrary binary into printable ASCII using base64 encoding.
* very loosely based on the base64 Reader by
* Dr. Mark Thornton
* Optrak Distribution Software Ltd.
* http://www.optrak.co.uk
* and Kevin Kelley‘s http://www.ruralnet.net/~kelley/java/base64.java
*
* base64 is a way of encoding 8-bit characters using only ASCII printable
* characters similar to UUENCODE. UUENCODE includes a filename where base64 does not.
* The spec is described in RFC 2045. base64 is a scheme where
* 3 bytes are concatenated then split to form 4 groups of 6-bits each; and
* each 6-bits gets translated to an encoded printable ASCII character via a
* table lookup. An encoded string is therefore longer than the original by
* about 1/3. The “=“ character is used to pad the end. base64 is used
* among other things to encode the user:password string in an
* Authorization: header for HTTP. Don‘t confuse base64 with
* x-www-form-urlencoded which is handled by
* Java.net.URLEncoder.encode/decode
* If you don‘t like this code there is another implementation at http://www.ruffboy.com/download.htm
* Sun has an undocumented method called sun.misc.base64Encoder.encode.
* You could use hex simpler to code but not as compact.
*
* If you wanted to encode a giant file you could do it in large chunks that
* are even multiples of 3 bytes except for the last chunk and append the outputs.
*
* To encode a string rather than binary data java.net.URLEncoder may be better. See
* printable characters in the Java glossary for a discussion of the differences.
*
* version 1.4 2002 February 15 -- correct bugs with uneven line lengths
* allow you to configure line separator.
* now need base64 object and instance methods.
* new mailing address.
* version 1.3 2000 September 12 -- fix problems with estimating output length in encode
* version 1.2 2000 September 09 -- now handles decode as well.
* version 1.1 1999 December 04 -- more symmetrical encoding algorithm.
* more accurate StringBuffer allocation size.
* version 1.0 1999 December 03 -- posted in comp.lang.java.programmer.
* Futures Streams or files.
*/
public class base64
{
/**
* how we separate lines e.g. \n \r\n \r etc.
*/
private String lineSeparator = System.getProperty( “line.separator“ );
/**
* max chars per line excluding lineSeparator. A multiple of 4.
*/
private int lineLength = 72;
private char[] valueToChar = new char[64];
/**
* binary value encoded by a given letter of the alphabet 0..
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2017-04-13 00:06 fastdfs-client-java\
文件 1504 2017-04-12 11:18 fastdfs-client-java\.classpath
文件 1332 2017-04-12 22:17 fastdfs-client-java\.project
目录 0 2017-04-13 00:06 fastdfs-client-java\.settings\
文件 430 2017-04-12 11:18 fastdfs-client-java\.settings\org.eclipse.jdt.core.prefs
文件 90 2017-04-12 11:15 fastdfs-client-java\.settings\org.eclipse.m2e.core.prefs
文件 333 2017-04-12 11:18 fastdfs-client-java\.settings\org.eclipse.wst.common.component
文件 172 2017-04-12 11:18 fastdfs-client-java\.settings\org.eclipse.wst.common.project.facet.core.xm
文件 395 2017-04-12 11:15 fastdfs-client-java\pom.xm
目录 0 2017-04-13 00:06 fastdfs-client-java\src\
目录 0 2017-04-13 00:06 fastdfs-client-java\src\main\
目录 0 2017-04-13 00:06 fastdfs-client-java\src\main\java\
目录 0 2017-04-13 00:06 fastdfs-client-java\src\main\java\org\
目录 0 2017-04-13 00:06 fastdfs-client-java\src\main\java\org\csource\
目录 0 2017-04-13 00:06 fastdfs-client-java\src\main\java\org\csource\common\
文件 16789 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\common\ba
文件 3721 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\common\IniFileReader.java
文件 502 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\common\MyException.java
文件 972 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\common\NameValuePair.java
目录 0 2017-04-13 00:06 fastdfs-client-java\src\main\java\org\csource\fastdfs\
文件 5122 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\ClientGlobal.java
文件 784 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\DownloadCallback.java
文件 1039 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\DownloadStream.java
文件 2649 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\FileInfo.java
文件 17377 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\ProtoCommon.java
文件 1135 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\ProtoStructDecoder.java
文件 1273 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\ServerInfo.java
文件 65791 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\StorageClient.java
文件 25319 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\StorageClient1.java
文件 1532 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\StorageServer.java
文件 1947 2017-04-12 11:23 fastdfs-client-java\src\main\java\org\csource\fastdfs\Structba
............此处省略90个文件信息
相关资源
- FastDFS相应的源码包以及安装教程Lin
- fastdfs断点续传代码
- fastdfs-java源码
- java上传文件到文件服务器源码和jar
- fastdfs_client-1.24.jar
- fastdfs-client-1.26上传
- FastDFS1.2jar包
- fastdfs_client-1.25.jar
- fastdfs-client-java-1.25.jar
- fastdfs-client-java的Maven工程
- fastdfs-client-java-1.27.jar
- Centos7上安装FastDFS并用-java7-实现上传
- fastdfs-client-java-1.27-SNAPSHOT.jar
评论
共有 条评论