资源简介
java GUI awt 实现鼠标绘制矩形,鼠标拖动矩形,鼠标改变矩形大小功能. 其它图形的绘制方法参考: https://blog.csdn.net/xietansheng/article/details/55669157
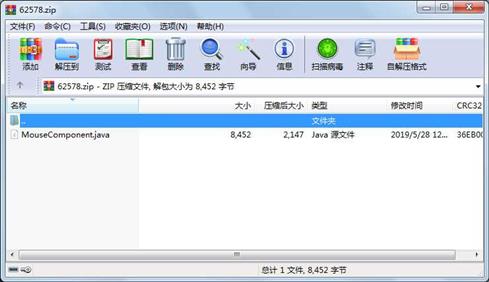
代码片段和文件信息
package com.test;
import java.awt.Cursor;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Rectangle;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseMotionListener;
import java.awt.geom.Point2D;
import java.util.ArrayList;
import javax.swing.JComponent;
import javax.swing.Jframe;
import javax.swing.JPanel;
public class MouseComponent extends JComponent implements MouseMotionListener {
private Rectangle rect;
private boolean isTopLeft;
private boolean isTop;
private boolean isTopRight;
private boolean isRight;
private boolean isBottomRight;
private boolean isBottom;
private boolean isBottomLeft;
private boolean isDrag;
private boolean isLeft;
//矩形最小宽和高为多少
private final static int RESIZE_WIDTH = 5;
private final static int MIN_WIDTH = 20;
private final static int MIN_HEIGHT = 20;
private static final int SIDELENGTH = 50;//单击增加的矩形大小
private ArrayList squares;//所有的矩形
private Rectangle current;
public MouseComponent() {
this.squares = new ArrayList();
this.rect = null;
addMouseMotionListener(this);
this.addMouseListener(new MouseHandle());// 监听鼠标点击事件
//this.addMouseMotionListener(new MouseMotionHandler());// 监听鼠标移动事件
}
// 如果鼠标单击屏幕的地方有矩形则不绘制矩形
public Rectangle find(Point2D p) {
for (Rectangle r : squares) {
if (r.contains(p))
return r;
}
return null;
}
// 绘制矩形
public void paintComponent(Graphics g) {
Graphics2D g2 = (Graphics2D) g;
for (Rectangle r : squares) {
g2.draw(r);
}
}
// 添加矩形到屏幕
public void add(Point2D p) {
double x = p.getX();
double y = p.getY();
rect = new Rectangle((int) (x - SIDELENGTH / 2) (int) (y - SIDELENGTH / 2) SIDELENGTH SIDELENGTH);
squares.add(rect);
repaint();
}
// 删除矩形
public void remove(Rectangle s) {
if (s == null)
return;
if (s == rect)
rect = null;
squares.remove(s);
repaint();
}
private class MouseHandle extends MouseAdapter {
@Override
public void mouseClicked(MouseEvent e) {
rect = find(e.getPoint());
// 当前位置如果有矩形,且点击大于2次,则删除矩形
if (rect != null && e.getClickCount() >= 2)
remove(rect);
}
@Override
public void mousePressed(MouseEvent e) {
rect = find(e.getPoint());
// 当前位置没有矩形则绘制
if (rect == null)
add(e.getPoint());
}
}
private class MouseMotionHandler implements MouseMotionListener {
@Override
public void mouseDragged(MouseEvent e) {
// 如果当前位置有矩形,进行拖拽。
if (rect
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 8452 2019-05-28 12:17 MouseComponent.java
相关资源
- jsonarray所必需的6个jar包.rar
- 三角网构TIN生成算法,Java语言实现
- java代码编写将excel数据导入到mysql数据
- Java写的cmm词法分析器源代码及javacc学
- JAVA JSP公司财务管理系统 源代码 论文
- JSP+MYSQL旅行社管理信息系统
- 推荐算法的JAVA实现
- 基于Java的酒店管理系统源码(毕业设
- java-图片识别 图片比较
- android毕业设计
- java23种设计模式+23个实例demo
- java Socket发送/接受报文
- JAVA828436
- java界面美化 提供多套皮肤直接使用
- 在线聊天系统(java代码)
- 基于Java的图书管理系统807185
- java中实现将页面数据导入Excel中
- java 企业销售管理系统
- java做的聊天系统(包括正规课程设计
- Java编写的qq聊天室
- 商店商品管理系统 JAVA写的 有界面
- JAVA开发聊天室程序
- 在linux系统下用java执行系统命令实例
- java期末考试试题两套(答案) 选择(
- JAVA3D编程示例(建模、交互)
- Java 文件加密传输
- java做的房产管理系统
- 基于jsp的bbs论坛 非常详细
- [免费]java实现有障碍物的贪吃蛇游戏
- java Servlet投票实例
评论
共有 条评论