资源简介
JGraphT is a free Java class library that provides mathematical graph-theory objects and algorithms. JGraphT supports a rich gallery of graphs and is designed to be powerful, extensible, and easy to use.
Packages
org.jgrapht:The front-end API's interfaces and classes, including Graph, DirectedGraph and UndirectedGraph.
org.jgrapht.alg:Algorithms provided with JGraphT.
org.jgrapht.alg.util:Utilities used by JGraphT algorithms.
org.jgrapht.demo:Demo programs that help to get started with JGraphT.
org.jgrapht.event:Event classes and listener interfaces, used to provide a change notification mechanism on graph modification events.
org.jgrapht.ext:Extensions and integration means to other products.
org.jgrapht.generate:Generators for graphs of various topologies.
org.jgrapht.graph:Implementations of various graphs.
org.jgrapht.traverse:Graph traversal means.
org.jgrapht.util:Non-graph-specific data structures, algorithms, and utilities used by JGraphT.
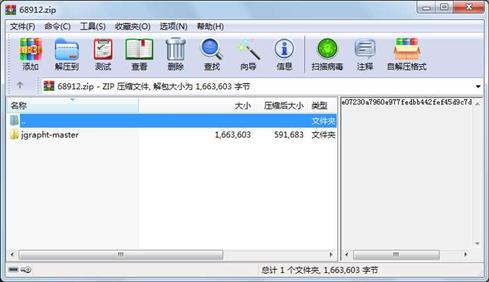
代码片段和文件信息
/* ==========================================
* JGraphT : a free Java graph-theory library
* ==========================================
*
* Project Info: http://jgrapht.sourceforge.net/
* Project Creator: Barak Naveh (http://sourceforge.net/users/barak_naveh)
*
* (C) Copyright 2003-2008 by Barak Naveh and Contributors.
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; either version 2.1 of the License or
* (at your option) any later version.
*
* This library is distributed in the hope that it will be useful but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
* License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this library; if not write to the Free Software Foundation
* Inc.
* 59 Temple Place Suite 330 Boston MA 02111-1307 USA.
*/
/* ------------------
* DirectedGraph.java
* ------------------
* (C) Copyright 2003-2008 by Barak Naveh and Contributors.
*
* Original Author: Barak Naveh
* Contributor(s): Christian Hammer
*
* $Id$
*
* Changes
* -------
* 24-Jul-2003 : Initial revision (BN);
* 11-Mar-2004 : Made generic (CH);
* 07-May-2006 : Changed from List to Set (JVS);
*
*/
package org.jgrapht;
import java.util.*;
/**
* A graph whose all edges are directed. This is the root interface of all
* directed graphs.
*
* See
* http://mathworld.wolfram.com/DirectedGraph.html for more on directed
* graphs.
*
* @author Barak Naveh
* @since Jul 14 2003
*/
public interface DirectedGraph
extends Graph
{
//~ Methods ----------------------------------------------------------------
/**
* Returns the “in degree“ of the specified vertex. An in degree of a vertex
* in a directed graph is the number of inward directed edges from that
* vertex. See
* http://mathworld.wolfram.com/Indegree.html.
*
* @param vertex vertex whose degree is to be calculated.
*
* @return the degree of the specified vertex.
*/
public int inDegreeOf(V vertex);
/**
* Returns a set of all edges incoming into the specified vertex.
*
* @param vertex the vertex for which the list of incoming edges to be
* returned.
*
* @return a set of all edges incoming into the specified vertex.
*/
public Set incomingEdgesOf(V vertex);
/**
* Returns the “out degree“ of the specified vertex. An out degree of a
* vertex in a directed graph is the number of outward directed edges from
* that vertex. See
* http://mathworld
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2012-03-07 13:53 jgrapht-master\
文件 223 2012-03-07 13:53 jgrapht-master\.gitignore
文件 23081 2012-03-07 13:53 jgrapht-master\README.html
目录 0 2012-03-07 13:53 jgrapht-master\etc\
文件 1941 2012-03-07 13:53 jgrapht-master\etc\build.properties.template
文件 26438 2012-03-07 13:53 jgrapht-master\etc\eclipse-formatter-settings.xm
文件 7058 2012-03-07 13:53 jgrapht-master\etc\graph-li
文件 3402 2012-03-07 13:53 jgrapht-master\etc\pom.xm
文件 6222 2012-03-07 13:53 jgrapht-master\etc\release-process.html
文件 32231 2012-03-07 13:53 jgrapht-master\etc\triemax-jalopy-settings.xm
目录 0 2012-03-07 13:53 jgrapht-master\jgrapht-core\
文件 599 2012-03-07 13:53 jgrapht-master\jgrapht-core\pom.xm
目录 0 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\
目录 0 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\
目录 0 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\
目录 0 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\
目录 0 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\
文件 3543 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\DirectedGraph.java
文件 2042 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\EdgeFactory.java
文件 17970 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\Graph.java
文件 1887 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\GraphHelper.java
文件 2651 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\GraphMapping.java
文件 3303 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\GraphPath.java
文件 14866 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\Graphs.java
文件 2688 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\ListenableGraph.java
文件 2248 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\UndirectedGraph.java
文件 1961 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\VertexFactory.java
文件 2165 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\WeightedGraph.java
目录 0 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\alg\
文件 5186 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\alg\AbstractPathElement.java
文件 5674 2012-03-07 13:53 jgrapht-master\jgrapht-core\src\main\java\org\jgrapht\alg\AbstractPathElementList.java
............此处省略345个文件信息
相关资源
- jsonarray所必需的6个jar包.rar
- 三角网构TIN生成算法,Java语言实现
- java代码编写将excel数据导入到mysql数据
- Java写的cmm词法分析器源代码及javacc学
- JAVA JSP公司财务管理系统 源代码 论文
- JSP+MYSQL旅行社管理信息系统
- 推荐算法的JAVA实现
- 基于Java的酒店管理系统源码(毕业设
- java-图片识别 图片比较
- android毕业设计
- java23种设计模式+23个实例demo
- java Socket发送/接受报文
- JAVA828436
- java界面美化 提供多套皮肤直接使用
- 在线聊天系统(java代码)
- 基于Java的图书管理系统807185
- java中实现将页面数据导入Excel中
- java 企业销售管理系统
- java做的聊天系统(包括正规课程设计
- Java编写的qq聊天室
- 商店商品管理系统 JAVA写的 有界面
- JAVA开发聊天室程序
- 在linux系统下用java执行系统命令实例
- java期末考试试题两套(答案) 选择(
- JAVA3D编程示例(建模、交互)
- Java 文件加密传输
- java做的房产管理系统
- 基于jsp的bbs论坛 非常详细
- [免费]java实现有障碍物的贪吃蛇游戏
- java Servlet投票实例
评论
共有 条评论