资源简介
Java语言程序设计与数据结构(基础篇)第15章课后习题代码
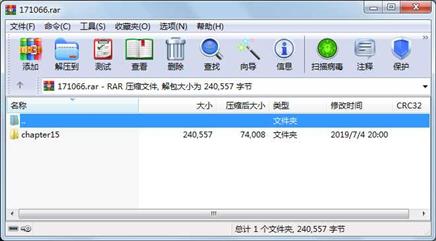
代码片段和文件信息
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.image.ImageView;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
import java.util.ArrayList;
public class Exercise15_01 extends Application {
@Override
// Override the start method in the Application class
public void start(Stage primaryStage) {
// There are two ways for shuffling. One is to use the hint in the book.
// The other way is to use the static shuffle method in the java.util.Collections class.
ArrayList list = new ArrayList<>();
for (int i = 1; i <= 52; i++) {
list.add(i);
}
java.util.Collections.shuffle(list);
HBox hBox = new HBox(5);
hBox.setAlignment(Pos.CENTER);
hBox.getChildren().add(new ImageView(“image/card/“ + list.get(0) + “.png“));
hBox.getChildren().add(new ImageView(“image/card/“ + list.get(1) + “.png“));
hBox.getChildren().add(new ImageView(“image/card/“ + list.get(2) + “.png“));
hBox.getChildren().add(new ImageView(“image/card/“ + list.get(3) + “.png“));
// Create a button
Button btRefresh = new Button(“Refresh“);
btRefresh.setOnAction(e -> {
java.util.Collections.shuffle(list);
hBox.getChildren().clear();
hBox.getChildren().add(new ImageView(“image/card/“ + list.get(0) + “.png“));
hBox.getChildren().add(new ImageView(“image/card/“ + list.get(1) + “.png“));
hBox.getChildren().add(new ImageView(“image/card/“ + list.get(2) + “.png“));
hBox.getChildren().add(new ImageView(“image/card/“ + list.get(3) + “.png“));
});
BorderPane pane = new BorderPane();
pane.setCenter(hBox);
pane.setBottom(btRefresh);
BorderPane.setAlignment(btRefresh Pos.TOP_CENTER);
// Create a scene and place it in the stage
Scene scene = new Scene(pane 250 150);
primaryStage.settitle(“Exercise15_01“); // Set the stage title
primaryStage.setScene(scene); // Place the scene in the stage
primaryStage.show(); // Display the stage
}
/**
* The main method is only needed for the IDE with limited
* JavaFX support. Not needed for running from the command line.
*/
public static void main(String[] args) {
launch(args);
}
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 867 2019-07-04 20:00 chapter15\chapter29\Exercise29_03.java
文件 2592 2019-07-04 20:00 chapter15\chapter29\Exercise29_04(1).java
文件 2592 2019-07-04 20:00 chapter15\chapter29\Exercise29_04.java
文件 2274 2019-07-04 20:00 chapter15\chapter29\Exercise29_05.java
文件 1544 2019-07-04 20:00 chapter15\chapter29\Exercise29_09.java
文件 1900 2019-07-04 20:00 chapter15\chapter29\Exercise29_10.java
文件 1612 2019-07-04 20:00 chapter15\chapter29\Exercise29_11.java
文件 4128 2019-07-04 20:00 chapter15\chapter29\Exercise29_12.java
文件 6167 2019-07-04 20:00 chapter15\chapter29\Exercise29_13.java
文件 6664 2019-07-04 20:00 chapter15\chapter29\Exercise29_14.java
文件 8446 2019-07-04 20:00 chapter15\chapter29\Exercise29_15.java
文件 7480 2019-07-04 20:00 chapter15\chapter29\Exercise29_16.java
文件 13770 2019-07-04 20:00 chapter15\chapter29\Exercise29_17.java
文件 24181 2019-07-04 20:00 chapter15\chapter29\Exercise29_18.java
文件 21827 2019-07-04 20:00 chapter15\chapter29\Exercise29_20.java
文件 2398 2019-07-04 20:00 chapter15\Exercise15_01(1).java
文件 2103 2019-07-04 20:00 chapter15\Exercise15_01Extra.java
文件 1575 2019-07-04 20:00 chapter15\Exercise15_02.java
文件 2784 2019-07-04 20:00 chapter15\Exercise15_02Extra.java
文件 2225 2019-07-04 20:00 chapter15\Exercise15_03.java
文件 1467 2019-07-04 20:00 chapter15\Exercise15_03Extra.java
文件 2677 2019-07-04 20:00 chapter15\Exercise15_04.java
文件 2729 2019-07-04 20:00 chapter15\Exercise15_04Extra.java
文件 2900 2019-07-04 20:00 chapter15\Exercise15_05.java
文件 8387 2019-07-04 20:00 chapter15\Exercise15_05Extra.java
文件 1172 2019-07-04 20:00 chapter15\Exercise15_06.java
文件 3363 2019-07-04 20:00 chapter15\Exercise15_06Extra.java
文件 1211 2019-07-04 20:00 chapter15\Exercise15_07.java
文件 5885 2019-07-04 20:00 chapter15\Exercise15_07Extra.java
文件 1170 2019-07-04 20:00 chapter15\Exercise15_08.java
............此处省略37个文件信息
- 上一篇:Louvain java实现
- 下一篇:java实现聊天的服务端
相关资源
- 微博系统(Java源码,servlet+jsp),适
- java串口通信全套完整代码-导入eclip
- jsonarray所必需的6个jar包.rar
- 三角网构TIN生成算法,Java语言实现
- java代码编写将excel数据导入到mysql数据
- Java写的cmm词法分析器源代码及javacc学
- JAVA JSP公司财务管理系统 源代码 论文
- JSP+MYSQL旅行社管理信息系统
- 推荐算法的JAVA实现
- 基于Java的酒店管理系统源码(毕业设
- java-图片识别 图片比较
- android毕业设计
- java23种设计模式+23个实例demo
- java Socket发送/接受报文
- JAVA828436
- java界面美化 提供多套皮肤直接使用
- 在线聊天系统(java代码)
- 基于Java的图书管理系统807185
- java中实现将页面数据导入Excel中
- java 企业销售管理系统
- java做的聊天系统(包括正规课程设计
- Java编写的qq聊天室
- 商店商品管理系统 JAVA写的 有界面
- JAVA开发聊天室程序
- 在linux系统下用java执行系统命令实例
- java期末考试试题两套(答案) 选择(
- JAVA3D编程示例(建模、交互)
- Java 文件加密传输
- java做的房产管理系统
- 基于jsp的bbs论坛 非常详细
评论
共有 条评论