资源简介
一些开发小游戏的程序的方案和代码实例,希望能够帮助到你们
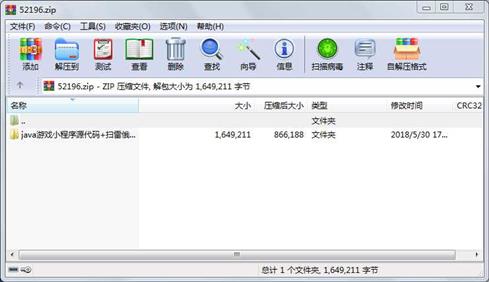
代码片段和文件信息
/*
* Copyright (c) 2000 David Flanagan. All rights reserved.
* This code is from the book Java Examples in a Nutshell 2nd Edition.
* It is provided AS-IS WITHOUT ANY WARRANTY either expressed or implied.
* You may study use and modify it for any non-commercial purpose.
* You may distribute it non-commercially as long as you retain this notice.
* For a commercial use license or to purchase the book (recommended)
* visit http://www.davidflanagan.com/javaexamples2.
*/
package com.davidflanagan.examples.applet;
import java.applet.*; // Don‘t forget this import statement!
import java.awt.*; // Or this one for the graphics!
import java.util.Date; // To obtain the current time
import java.text.DateFormat; // For displaying the time
/**
* This applet displays the time and updates it every second
**/
public class Clock extends applet implements Runnable {
Label time; // A component to display the time in
DateFormat timeFormat; // This object converts the time to a string
Thread timer; // The thread that updates the time
volatile boolean running; // A flag used to stop the thread
/**
* The init method is called when the browser first starts the applet.
* It sets up the Label component and obtains a DateFormat object
**/
public void init() {
time = new Label();
time.setFont(new Font(“helvetica“ Font.BOLD 12));
time.setAlignment(Label.CENTER);
setLayout(new BorderLayout());
add(time BorderLayout.CENTER);
timeFormat = DateFormat.getTimeInstance(DateFormat.MEDIUM);
}
/**
* This browser calls this method to tell the applet to start running.
* Here we create and start a thread that will update the time each
* second. Note that we take care never to have more than one thread
**/
public void start() {
running = true; // Set the flag
if (timer == null) { // If we don‘t already have a thread
timer = new Thread(this); // Then create one
timer.start(); // And start it running
}
}
/**
* This method implements Runnable. It is the body of the thread. Once
* a second it updates the text of the Label to display the current time
**/
public void run() {
while(running) { // Loop until we‘re stopped
// Get current time convert to a String and display in the Label
time.setText(timeFormat.format(new Date()));
// Now wait 1000 milliseconds
try { Thread.sleep(1000); }
catch (InterruptedException e) {}
}
// If the thread exits set it to null so we can create a new one
// if start() is called again.
timer = null;
}
/**
* The browser calls this method to tell the applet that it is not visible
* and should not run. It sets a flag that tells the run() method to exit
**/
public void stop() { running = false; }
/**
* Returns information about the applet for display by th
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2018-05-30 17:16 java游戏小程序源代码+扫雷俄罗斯方块游戏\
目录 0 2008-03-04 20:35 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\
目录 0 2008-03-04 20:36 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\
目录 0 2000-08-18 15:31 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 118 2000-06-22 15:52 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 3130 2000-08-18 12:48 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 213 2000-06-22 15:52 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 2355 2000-08-18 12:48 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 125 2000-06-22 15:54 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 5135 2000-08-18 12:48 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 125 2000-06-22 15:52 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 863 2000-08-18 12:48 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 122 2000-06-22 15:52 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 2282 2000-08-18 12:48 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 1531 2000-07-13 13:31 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 6141 2000-08-18 12:48 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 1227 2000-06-22 15:50 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
文件 13945 2000-07-13 13:19 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\ap
目录 0 2000-08-18 15:31 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\
文件 1048 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\Echo.java
文件 1757 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\FactComputer.java
文件 1695 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\FactQuoter.java
文件 1050 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\Factorial.java
文件 961 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\Factorial2.java
文件 1732 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\Factorial3.java
文件 2340 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\Factorial4.java
文件 1394 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\Fibonacci.java
文件 1583 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\FizzBuzz.java
文件 1722 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\FizzBuzz2.java
文件 927 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\Hello.java
文件 1198 2000-08-18 12:51 java游戏小程序源代码+扫雷俄罗斯方块游戏\java小程序源代码\164个JAVA完美程序\basics\Reverse.java
............此处省略291个文件信息
相关资源
- 局域网聊天程序,群聊。私聊。发文
- 在线聊天系统(java代码)
- java做的聊天系统(包括正规课程设计
- Java编写的qq聊天室
- JAVA开发聊天室程序
- 超级好的纯jsp写的聊天室
- Java实现的聊天室,具有群聊和私聊功
-
Java Jfr
ame简单聊天程序 - java版多人聊天室
- 俄罗斯方块具体设计(Java描述)带详
- 基于java聊天室(gui)
- Java版聊天程序(UDP TCP 多线程)
- 简单qq聊天(Java socket实现)
- 用Java编写的扫雷游戏源代码
- 采用TCP SOCKET技术编写C/S模式的java聊天
- 简单多线程一对一聊天程序
- Android游戏源码基于蓝牙的坦克大战和
- Swing俄罗斯方块
- windows经典扫雷游戏Java版
- JAVA局域网聊天系统微仿QQ
- JAVA实训 俄罗斯方块(带源程序和实
- Android仿QQ聊天系统Android
- zw_java视频聊天anychat.zip
- android扫雷小游戏源码
- Android的模仿聊天QQ
- java/swing编写的第一个扫雷程序
- java多人聊天室可私聊
- android仿微信聊天软件源代码
- android 仿微信语音聊天demo
- 计算机网络课程设计报告_DH算法_Wir
评论
共有 条评论