资源简介
官方源码,解压后可以直接使用,对数据库开发非常有帮助,也很方便。
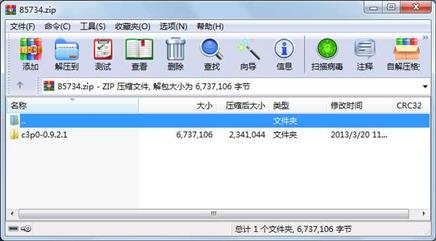
代码片段和文件信息
import java.sql.*;
import javax.naming.*;
import javax.sql.DataSource;
import com.mchange.v2.c3p0.DataSources;
/**
* This example shows how to acquire a c3p0 DataSource and
* bind it to a JNDI name service.
*/
public final class JndiBindDataSource
{
// be sure to load your database driver class either via
// Class.forName() [as shown below] or externally (e.g. by
// using -Djdbc.drivers when starting your JVM).
static
{
try
{ Class.forName( “org.postgresql.Driver“ ); }
catch (Exception e)
{ e.printStackTrace(); }
}
public static void main(String[] argv)
{
try
{
// let a command line arg specify the name we will
// bind our DataSource to.
String jndiName = argv[0];
// acquire the DataSource using default pool params...
// this is the only c3p0 specific code here
DataSource unpooled = DataSources.unpooledDataSource(“jdbc:postgresql://localhost/test“
“swaldman“
“test“);
DataSource pooled = DataSources.pooledDataSource( unpooled );
// Create an InitialContext and bind the DataSource to it in
// the usual way.
//
// We are using the no-arg version of InitialContext‘s constructor
// therefore the jndi environment must be first set via a jndi.properties
// file System properties or by some other means.
InitialContext ctx = new InitialContext();
ctx.rebind( jndiName pooled );
System.out.println(“DataSource bound to nameservice under the name \““ +
jndiName + ‘\“‘);
}
catch (Exception e)
{ e.printStackTrace(); }
}
static void attemptClose(ResultSet o)
{
try
{ if (o != null) o.close();}
catch (Exception e)
{ e.printStackTrace();}
}
static void attemptClose(Statement o)
{
try
{ if (o != null) o.close();}
catch (Exception e)
{ e.printStackTrace();}
}
static void attemptClose(Connection o)
{
try
{ if (o != null) o.close();}
catch (Exception e)
{ e.printStackTrace();}
}
private JndiBindDataSource()
{}
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs-oracle-thin\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs-oracle-thin\com\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs-oracle-thin\com\mchange\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs-oracle-thin\com\mchange\v2\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs-oracle-thin\com\mchange\v2\c3p0\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs-oracle-thin\com\mchange\v2\c3p0\dbms\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs-oracle-thin\resources\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\cfg\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\codegen\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\filter\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\impl\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\jboss\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\management\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\mbean\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\servlet\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\stmt\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\subst\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\test\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\test\junit\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\c3p0\util\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\com\mchange\v2\resourcepool\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\apidocs\resources\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\doc\old\
目录 0 2013-03-20 11:16 c3p0-0.9.2.1\examples\
............此处省略210个文件信息
评论
共有 条评论