资源简介
求解大规模具有能力约束的车辆路径问题(Capacitated Vehicle Routing Problem, CVRP)求解大规模具有能力约束的车辆路径问题(Capacitated Vehicle Routing Problem, CVRP)求解大规模具有能力约束的车辆路径问题(Capacitated Vehicle Routing Problem, CVRP)求解大规模具有能力约束的车辆路径问题(Capacitated Vehicle Routing Problem, CVRP)
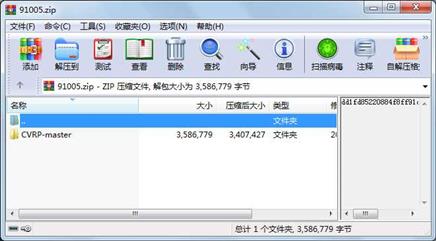
代码片段和文件信息
import java.io.*;
import java.awt.Point;
/**
* CVRPData.java
*
* This class reads in a CVRP data file and provides “get methods“ to
* access the demand and distance information. It does some
* parameter checking to make sure that nodes passed to the get
* methods are within the valid range.
*
* It should be initialised by calling readFile().
*
* @author Tim Kovacs
* @version 20/10/2013
*/
public class CVRPData {
/** The capacity that each vehicle has */
public static int VEHICLE_CAPACITY;
/** The number of nodes in the fruitybun CVRP i.e. the depot and the customers */
public static int NUM_NODES;
/** This is only used for testing. Normally the class is initialised by calling readFile() */
public static void main(String[] dataFileName) throws FileNotFoundException IOException {
if (dataFileName.length != 1)
quit(“I need exactly 1 argument: the name of the data file to read“);
readFile(dataFileName[0]);
}
/** Return the demand for a given node. */
public static int getDemand(int node) {
if (!nodeIsValid(node)) {
System.err.println(“Error: demand for node “ + node +
“ was requested from getDemand() but only nodes 1..“ + NUM_NODES + “ exist“);
System.exit(-1);
}
return demand[node];
}
/** Return the Euclidean distance between the two given nodes */
public static double getDistance(int node1 int node2) {
if (!nodeIsValid(node1)) {
System.err.println(“Error: distance for node “ + node1 +
“ was requested from getDistance() but only nodes 1..“ + NUM_NODES + “ exist“);
System.exit(-1);
}
if (!nodeIsValid(node2)) {
System.err.println(“Error: distance for node “ + node2 +
“ was requested from getDistance() but only nodes 1..“ + NUM_NODES + “ exist“);
System.exit(-1);
}
int x1 = coords[node1][X_COORDINATE];
int y1 = coords[node1][Y_COORDINATE];
int x2 = coords[node2][X_COORDINATE];
int y2 = coords[node2][Y_COORDINATE];
// compute Euclidean distance
return Math.sqrt(Math.pow((x1-x2)2) + Math.pow((y1-y2)2));
// For example the distance between 3344 and 4413 is:
// 33-44 = -11 and 11^2 = 121
// 44-13 = 31 and 31^2 = 961
// 121 + 961 = 1082
// The square root of 1082 = 32.893768...
}
//* Return the absolute co-ordinates of the given node */
public static Point getLocation(int node) {
if (!nodeIsValid(node)) {
System.err.println(“Error: Request for location of non-existent node “ + node + “.“);
System.exit(-1);
}
return new Point(coords[node][X_COORDINATE] coords[node][Y_COORDINATE]);
}
/** Return true if the given node is within the valid range (1..NUM_NODES) false otherwise */
private static boolean nodeIsValid(int node) {
if (node < 1 || node > NUM_NODES)
return false;
else
return true;
}
private static final int X_COORDINATE = 0; // x-axis coordinate is dimension 0 in coords[][]
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2016-11-17 21:00 CVRP-master\
文件 121190 2016-11-17 21:00 CVRP-master\COMSM0305-1.pdf
文件 148903 2016-11-17 21:00 CVRP-master\COMSM0305-2.pdf
文件 112511 2016-11-17 21:00 CVRP-master\COMSM0305-2print.pdf
目录 0 2016-11-17 21:00 CVRP-master\CVRP\
文件 6356 2016-11-17 21:00 CVRP-master\CVRP\CVRPData.java
文件 17752 2016-11-17 21:00 CVRP-master\CVRP\CVRPValidater.jar
文件 4260 2016-11-17 21:00 CVRP-master\CVRP\fruitybun250.vrp
文件 3175807 2016-11-17 21:00 CVRP-master\SuttonBook.pdf
相关资源
- jsonarray所必需的6个jar包.rar
- 三角网构TIN生成算法,Java语言实现
- java代码编写将excel数据导入到mysql数据
- Java写的cmm词法分析器源代码及javacc学
- JAVA JSP公司财务管理系统 源代码 论文
- JSP+MYSQL旅行社管理信息系统
- 推荐算法的JAVA实现
- 基于Java的酒店管理系统源码(毕业设
- java-图片识别 图片比较
- android毕业设计
- java23种设计模式+23个实例demo
- java Socket发送/接受报文
- JAVA828436
- java界面美化 提供多套皮肤直接使用
- 在线聊天系统(java代码)
- 基于Java的图书管理系统807185
- java中实现将页面数据导入Excel中
- java 企业销售管理系统
- java做的聊天系统(包括正规课程设计
- Java编写的qq聊天室
- 商店商品管理系统 JAVA写的 有界面
- JAVA开发聊天室程序
- 在linux系统下用java执行系统命令实例
- java期末考试试题两套(答案) 选择(
- JAVA3D编程示例(建模、交互)
- Java 文件加密传输
- java做的房产管理系统
- 基于jsp的bbs论坛 非常详细
- [免费]java实现有障碍物的贪吃蛇游戏
- java Servlet投票实例
评论
共有 条评论