资源简介
超文本传输协议(HTTP,HyperText Transfer Protocol)是互联网上应用最为广泛的一种网络协议。所有的WWW文件都必须遵守这个标准。设计HTTP最初的目的是为了提供一种发布和接收HTML页面的方法。1960年美国人Ted Nelson构思了一种通过计算机处理文本信息的方法,并称之为超文本(hypertext),这成为了HTTP超文本传输协议标准架构的发展根基。Ted Nelson组织协调万维网协会(World Wide Web Consortium)和互联网工程工作小组(Internet Engineering Task Force )共同合作研究,最终发布了一系列的RFC,其中著名的RFC 2616定义了HTTP 1.1。
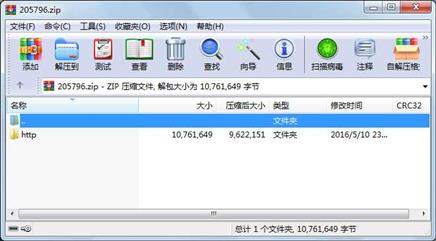
代码片段和文件信息
/*
* ====================================================================
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License Version 2.0 (the
* “License“); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing
* software distributed under the License is distributed on an
* “AS IS“ BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
* ====================================================================
*
* This software consists of voluntary contributions made by many
* individuals on behalf of the Apache Software Foundation. For more
* information on the Apache Software Foundation please see
* .
*
*/
package org.apache.http.client.fluent;
import java.util.linkedList;
import java.util.Queue;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import org.apache.http.concurrent.FutureCallback;
/**
* This example demonstrates how the he HttpClient fluent API can be used to execute multiple
* requests asynchronously using background threads.
*/
public class FluentAsync {
public static void main(String[] args)throws Exception {
// Use pool of two threads
ExecutorService threadpool = Executors.newFixedThreadPool(2);
Async async = Async.newInstance().use(threadpool);
Request[] requests = new Request[] {
Request.Get(“http://www.google.com/“)
Request.Get(“http://www.yahoo.com/“)
Request.Get(“http://www.apache.com/“)
Request.Get(“http://www.apple.com/“)
};
Queue> queue = new linkedList>();
// Execute requests asynchronously
for (final Request request: requests) {
Future future = async.execute(request new FutureCallback() {
public void failed(final Exception ex) {
System.out.println(ex.getMessage() + “: “ + request);
}
public void completed(final Content content) {
System.out.println(“Request completed: “ + request);
}
public void cancelled() {
}
});
queue.add(future);
}
while(!queue.isEmpty()) {
Future
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2016-05-10 23:03 http\
文件 3066205 2016-03-15 17:24 http\httpclient4.3.6.zip
文件 1235899 2016-05-06 23:26 http\httpcomponents-client-4.3.1-bin.zip
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\
文件 10349 2014-11-02 14:58 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\LICENSE.txt
文件 189 2014-11-02 14:58 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\NOTICE.txt
文件 2704 2014-11-02 14:58 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\README.txt
文件 79127 2014-11-02 14:58 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\RELEASE_NOTES.txt
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\client\
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\client\fluent\
文件 3232 2014-11-02 15:00 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\client\fluent\FluentAsync.java
文件 3336 2014-11-02 15:00 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\client\fluent\FluentExecutor.java
文件 1847 2014-11-02 15:00 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\client\fluent\FluentQuickStart.java
文件 2782 2014-11-02 15:00 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\client\fluent\FluentRequests.java
文件 4032 2014-11-02 15:00 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\client\fluent\FluentResponseHandling.java
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\
目录 0 2016-05-10 23:03 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\
文件 2451 2014-11-02 14:59 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\ClientAbortMethod.java
文件 2974 2014-11-02 14:59 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\ClientAuthentication.java
文件 3304 2014-11-02 14:59 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\ClientChunkEncodedPost.java
文件 13393 2014-11-02 14:59 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\ClientConfiguration.java
文件 3405 2014-11-02 14:59 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\ClientConnectionRelease.java
文件 3283 2014-11-02 14:59 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\ClientCustomContext.java
文件 3817 2014-11-02 14:59 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\ClientCustomSSL.java
文件 4678 2014-11-02 14:59 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\ClientEvictExpiredConnections.java
文件 2796 2014-11-02 14:59 http\httpcomponents-client-4.3.6-bin\httpcomponents-client-4.3.6\examples\org\apache\http\examples\client\ClientExecuteProxy.java
............此处省略49个文件信息
相关资源
- JNA所需要的jar包
- jsonarray所必需的6个jar包.rar
- utgard用到的jar包
- commons-beanutils-1.8.3.jar
- ehcache-core-2.5.1.jar
- android-support-v4.jar已打包进去源代码
- java实现小型函数画图板(附源代码、
- java读取DBF解决方案(可以解决javadb
- JavaHTTP协议实现
- jsp 统计在线人数利用HttpSessionListene
- Android v7的一些jar包
- windows 系统下启动与结束java的jar包的
- axis.jar及依赖jar包
- axis2需要的1.6.2jar
- commons-codec-1.3.jar和commons-httpclient-3.0
- Java HttpClient 4.x Jar包
-
ba
se64Encode编码,jar包源代码打包 - sqlserver2008连接所需jar包六个
- jdk和cglib动态代理的{jar包+源码}
- cglib-2.2.2.jar 和 asm-all-3.0.jar
- cglibjar包
- 传智itcast-bookstore所需jar包
- xalan-2.7.1.jar
- mysql-connector-java-5.0.8-bin驱动jar
- kettle连接数据库相关jar包
- 官方mysql-connector-java-5.1.28-bin.jar
- mysql-connector-java-5.1.17.jar
- Android直连Mysql数据库需要导入的jar包
- 音乐相关的jar
- oracle11g jar包for JDBC
评论
共有 条评论