资源简介
针对流媒体传输开发的rtp/rtcp协议源代码,底层通过UDP协议传输,上层封装rtp/rtcp控制,优化视频传输效果,可应用于视频聊天工具,视频会议软件,监控系统等的开发。
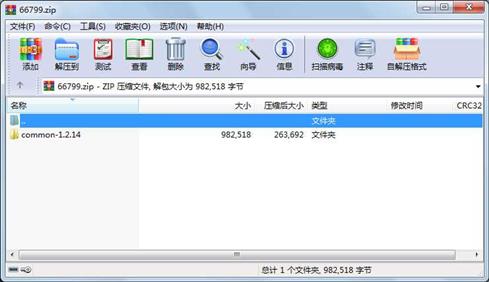
代码片段和文件信息
/*
* rtpdemo: A simple rtp application that sends and receives data.
*
* (C) 2000-2001 University College London.
*/
#include
#include
#include
#include
#include
#include
#include
#include “uclconf.h“
#include “config_unix.h“
#include “config_win32.h“
#include “debug.h“
#include “memory.h“
#include “rtp.h“
static void
usage()
{
printf(“Usage: rtpdemo [switches] address port\n“);
printf(“Valid switches are:\n“);
printf(“ -f\t\tFilter local packets out of receive stream.\n“);
printf(“ -l\t\tListen and do not transmit data.\n“);
exit(-1);
}
/* ------------------------------------------------------------------------- */
/* RTP callback related */
static void
sdes_print(struct rtp *session uint32_t ssrc rtcp_sdes_type stype) {
const char *sdes_type_names[] = {
“end“ “cname“ “name“ “email“ “telephone“
“location“ “tool“ “note“ “priv“
};
const uint8_t n = sizeof(sdes_type_names) / sizeof(sdes_type_names[0]);
if (stype > n) {
/* Theoretically impossible */
printf(“boo! invalud sdes field %d\n“ stype);
return;
}
printf(“SSRC 0x%08x reported SDES type %s - “ ssrc
sdes_type_names[stype]);
if (stype == RTCP_SDES_PRIV) {
/* Requires extra-handling not important for example */
printf(“don‘t know how to display.\n“);
} else {
printf(“%s\n“ rtp_get_sdes(session ssrc stype));
}
}
static void
packet_print(struct rtp *session rtp_packet *p)
{
printf(“Received data (payload %d timestamp %06d size %d) “ p->pt p->ts p->data_len);
if (p->ssrc == rtp_my_ssrc(session)) {
/* Unless filtering is enabled we are likely to see
out packets if sending to a multicast group. */
printf(“that I just sent.\n“);
} else {
printf(“from SSRC 0x%08x\n“ p->ssrc);
}
}
static void
rtp_event_handler(struct rtp *session rtp_event *e)
{
rtp_packet *p;
rtcp_sdes_item *r;
switch(e->type) {
case RX_RTP:
p = (rtp_packet*)e->data;
packet_print(session p);
xfree(p); /* xfree() is mandatory to release RTP packet data */
break;
case RX_SDES:
r = (rtcp_sdes_item*)e->data;
sdes_print(session e->ssrc r->type);
break;
case RX_BYE:
break;
case SOURCE_CREATED:
printf(“New source created SSRC = 0x%08x\n“ e->ssrc);
break;
case SOURCE_DELETED:
printf(“Source deleted SSRC = 0x%08x\n“ e->ssrc);
break;
case RX_SR:
case RX_RR:
case RX_RR_EMPTY:
case RX_RTCP_START:
case RX_RTCP_FINISH:
case RR_TIMEOUT:
case RX_APP:
break;
}
fflush(stdout);
}
/* ------------------------------------------------------------------------- */
/* Send and receive loop. Sender use 20ms audio mulaw packets */
#define MULAW_BYTES 4 * 160
#define MULAW_PAYLOAD 0
#define MULAW_MS 4 * 20
#define MAX_ROUNDS 100
static void
rxtx_loop(struct rtp* session int send_enable)
{
struct timeval t
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 525 2001-05-10 20:25 common-1.2.14\Makefile.in
文件 108 2001-03-10 14:54 common-1.2.14\.cvsignore
文件 1966 2000-01-04 13:27 common-1.2.14\COPYRIGHT
文件 20668 2003-05-23 12:43 common-1.2.14\MODS
文件 27653 1999-11-18 22:49 common-1.2.14\config.guess
文件 416 2001-03-10 14:54 common-1.2.14\README
文件 8 2003-05-23 12:42 common-1.2.14\VERSION
文件 535 1998-11-14 17:46 common-1.2.14\common.dsw
文件 10330 2003-04-02 12:03 common-1.2.14\configure.in
文件 20769 1999-11-18 22:49 common-1.2.14\config.sub
文件 85626 2003-04-02 12:16 common-1.2.14\configure
目录 0 2003-05-23 12:49 common-1.2.14\doc\
目录 0 2003-05-23 12:49 common-1.2.14\doc\html\
文件 3492 2001-04-01 23:18 common-1.2.14\doc\html\uclmmba
文件 2276 2001-04-01 23:18 common-1.2.14\doc\html\book1.html
文件 6138 2001-03-26 21:47 common-1.2.14\doc\html\uclmmba
文件 9108 2001-03-26 21:47 common-1.2.14\doc\html\uclmmba
文件 22600 2001-03-26 21:47 common-1.2.14\doc\html\uclmmba
文件 81144 2001-04-04 14:36 common-1.2.14\doc\html\uclmmba
文件 703 2001-03-10 14:54 common-1.2.14\doc\Makefile.in
文件 3123 2001-03-10 17:19 common-1.2.14\doc\README
目录 0 2003-05-23 12:49 common-1.2.14\doc\tmpl\
文件 1250 2001-03-10 14:54 common-1.2.14\doc\tmpl\config_unix.sgml
文件 299 2001-03-10 14:54 common-1.2.14\doc\tmpl\acconfig.sgml
文件 2237 2001-03-10 14:54 common-1.2.14\doc\tmpl\addrinfo.sgml
文件 291 2001-03-10 14:54 common-1.2.14\doc\tmpl\addrsize.sgml
文件 861 2001-03-10 14:54 common-1.2.14\doc\tmpl\asarray.sgml
文件 500 2001-03-10 14:54 common-1.2.14\doc\tmpl\ba
文件 416 2001-03-10 14:54 common-1.2.14\doc\tmpl\bittypes.sgml
文件 1043 2001-03-10 14:54 common-1.2.14\doc\tmpl\btree.sgml
文件 229 2001-03-10 14:54 common-1.2.14\doc\tmpl\cdecl_ext.sgml
............此处省略117个文件信息
- 上一篇:LPC2294开发板资源
- 下一篇:图书管理系统类图绘画的完整思路含图
评论
共有 条评论