资源简介
内存加载动态库 MemoryLoadLibrary 有例子。
/*
* Memory DLL loading code
* Version 0.0.3
*
* Copyright (c) 2004-2013 by Joachim Bauch / mail@joachim-bauch.de
* http://www.joachim-bauch.de
*
* The contents of this file are subject to the Mozilla Public License Version
* 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* The Original Code is MemoryModule.h
*
* The Initial Developer of the Original Code is Joachim Bauch.
*
* Portions created by Joachim Bauch are Copyright (C) 2004-2013
* Joachim Bauch. All Rights Reserved.
*
*/
#ifndef __MEMORY_MODULE_HEADER
#define __MEMORY_MODULE_HEADER
#include
typedef void *HMEMORYMODULE;
typedef void *HMEMORYRSRC;
typedef void *HCUSTOMMODULE;
#ifdef __cplusplus
extern "C" {
#endif
typedef HCUSTOMMODULE (*CustomLoadLibraryFunc)(LPCSTR, void *);
typedef FARPROC (*CustomGetProcAddressFunc)(HCUSTOMMODULE, LPCSTR, void *);
typedef void (*CustomFreeLibraryFunc)(HCUSTOMMODULE, void *);
/**
* Load DLL from memory location.
*
* All dependencies are resolved using default LoadLibrary/GetProcAddress
* calls through the Windows API.
*/
HMEMORYMODULE MemoryLoadLibrary(const void *);
/**
* Load DLL from memory location using custom dependency resolvers.
*
* Dependencies will be resolved using passed callback methods.
*/
HMEMORYMODULE MemoryLoadLibraryEx(const void *,
CustomLoadLibraryFunc,
CustomGetProcAddressFunc,
CustomFreeLibraryFunc,
void *);
/**
* Get address of exported method.
*/
FARPROC MemoryGetProcAddress(HMEMORYMODULE, LPCSTR);
/**
* Free previously loaded DLL.
*/
void MemoryFreeLibrary(HMEMORYMODULE);
/**
* Find the location of
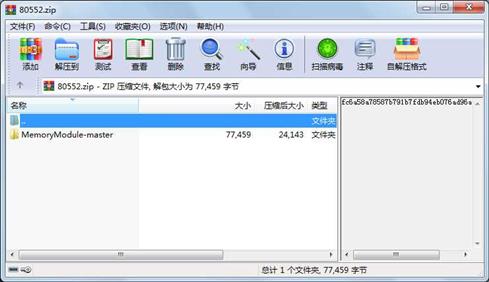
代码片段和文件信息
/*
* Memory DLL loading code
* Version 0.0.3
*
* Copyright (c) 2004-2013 by Joachim Bauch / mail@joachim-bauch.de
* http://www.joachim-bauch.de
*
* The contents of this file are subject to the Mozilla Public License Version
* 2.0 (the “License“); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an “AS IS“ basis
* WITHOUT WARRANTY OF ANY KIND either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* The Original Code is MemoryModule.c
*
* The Initial Developer of the Original Code is Joachim Bauch.
*
* Portions created by Joachim Bauch are Copyright (C) 2004-2013
* Joachim Bauch. All Rights Reserved.
*
*/
#ifndef __GNUC__
// disable warnings about pointer <-> DWORD conversions
#pragma warning( disable : 4311 4312 )
#endif
#ifdef _WIN64
#define POINTER_TYPE ULONGLONG
#else
#define POINTER_TYPE DWORD
#endif
#include
#include
#include
#ifdef DEBUG_OUTPUT
#include
#endif
#ifndef IMAGE_SIZEOF_base_RELOCATION
// Vista SDKs no longer define IMAGE_SIZEOF_base_RELOCATION!?
#define IMAGE_SIZEOF_base_RELOCATION (sizeof(IMAGE_base_RELOCATION))
#endif
#include “MemoryModule.h“
typedef struct {
PIMAGE_NT_HEADERS headers;
unsigned char *codebase;
HCUSTOMMODULE *modules;
int numModules;
int initialized;
CustomLoadLibraryFunc loadLibrary;
CustomGetProcAddressFunc getProcAddress;
CustomFreeLibraryFunc freeLibrary;
void *userdata;
} MEMORYMODULE *PMEMORYMODULE;
typedef BOOL (WINAPI *DllEntryProc)(HINSTANCE hinstDLL DWORD fdwReason LPVOID lpReserved);
#define GET_HEADER_DICTIONARY(module idx) &(module)->headers->OptionalHeader.DataDirectory[idx]
#ifdef DEBUG_OUTPUT
static void
OutputLastError(const char *msg)
{
LPVOID tmp;
char *tmpmsg;
FormatMessage(FORMAT_MESSAGE_ALLOCATE_BUFFER | FORMAT_MESSAGE_FROM_SYSTEM | FORMAT_MESSAGE_IGNORE_INSERTS
NULL GetLastError() MAKELANGID(LANG_NEUTRAL SUBLANG_DEFAULT) (LPTSTR)&tmp 0 NULL);
tmpmsg = (char *)LocalAlloc(LPTR strlen(msg) + strlen(tmp) + 3);
sprintf(tmpmsg “%s: %s“ msg tmp);
OutputDebugString(tmpmsg);
LocalFree(tmpmsg);
LocalFree(tmp);
}
#endif
static void
CopySections(const unsigned char *data PIMAGE_NT_HEADERS old_headers PMEMORYMODULE module)
{
int i size;
unsigned char *codebase = module->codebase;
unsigned char *dest;
PIMAGE_SECTION_HEADER section = IMAGE_FIRST_SECTION(module->headers);
for (i=0; iheaders->FileHeader.NumberOfSections; i++ section++) {
if (section->SizeOfRawData == 0) {
// section doesn‘t contain data in the dll itself but may define
// uninitialized data
size = old_headers->OptionalHeader.SectionAlignment;
if (size > 0) {
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2013-03-12 09:00 MemoryModule-master\
文件 16 2013-03-12 09:00 MemoryModule-master\.gitignore
文件 16726 2013-03-12 09:00 MemoryModule-master\LICENSE.txt
文件 244 2013-03-12 09:00 MemoryModule-master\Makefile
文件 24810 2013-03-12 09:00 MemoryModule-master\MemoryModule.c
文件 2743 2013-03-12 09:00 MemoryModule-master\MemoryModule.h
目录 0 2013-03-12 09:00 MemoryModule-master\doc\
文件 18587 2013-03-12 09:00 MemoryModule-master\doc\readme.txt
目录 0 2013-03-12 09:00 MemoryModule-master\example\
目录 0 2013-03-12 09:00 MemoryModule-master\example\DllLoader\
文件 9 2013-03-12 09:00 MemoryModule-master\example\DllLoader\.gitignore
文件 2829 2013-03-12 09:00 MemoryModule-master\example\DllLoader\DllLoader.cpp
文件 3386 2013-03-12 09:00 MemoryModule-master\example\DllLoader\DllLoader.vcproj
文件 533 2013-03-12 09:00 MemoryModule-master\example\DllLoader\Makefile
文件 1483 2013-03-12 09:00 MemoryModule-master\example\DllMemory.sln
文件 256 2013-03-12 09:00 MemoryModule-master\example\Makefile
目录 0 2013-03-12 09:00 MemoryModule-master\example\SampleDLL\
文件 16 2013-03-12 09:00 MemoryModule-master\example\SampleDLL\.gitignore
文件 628 2013-03-12 09:00 MemoryModule-master\example\SampleDLL\Makefile
文件 106 2013-03-12 09:00 MemoryModule-master\example\SampleDLL\SampleDLL.cpp
文件 188 2013-03-12 09:00 MemoryModule-master\example\SampleDLL\SampleDLL.h
文件 686 2013-03-12 09:00 MemoryModule-master\example\SampleDLL\SampleDLL.rc
文件 3458 2013-03-12 09:00 MemoryModule-master\example\SampleDLL\SampleDLL.vcproj
文件 755 2013-03-12 09:00 MemoryModule-master\readme.txt
- 上一篇:在线图书销售系统
- 下一篇:R_apriori.txt脚本
评论
共有 条评论