资源简介
jsoncpp1.8.4
修改对json项大小写敏感的比较,可自定义选择是否需要大小写敏感
修改json输出自动排序的规则,可自定义选择是否需要自动排序
新增json无格式化输出方法
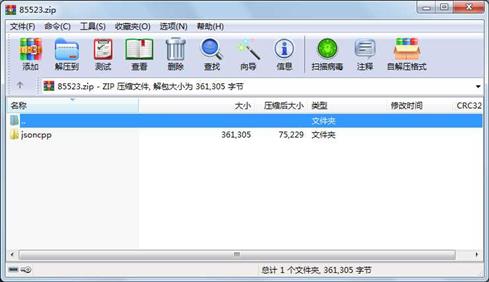
代码片段和文件信息
// Copyright 2007-2010 Baptiste Lepilleur and The JsonCpp Authors
// Distributed under MIT license or public domain if desired and
// recognized in your jurisdiction.
// See file LICENSE for detail or copy at http://jsoncpp.sourceforge.net/LICENSE
#if defined(__GNUC__)
#pragma GCC diagnostic push
#pragma GCC diagnostic ignored “-Wdeprecated-declarations“
#elif defined(_MSC_VER)
#pragma warning(disable : 4996)
#endif
/* This executable is used for testing parser/writer using real JSON files.
*/
#include // sort
#include
#include
#include
struct Options {
JSONCPP_STRING path;
Json::Features features;
bool parseOnly;
typedef JSONCPP_STRING (*writeFuncType)(Json::Value const&);
writeFuncType write;
};
static JSONCPP_STRING normalizeFloatingPointStr(double value) {
char buffer[32];
#if defined(_MSC_VER) && defined(__STDC_SECURE_LIB__)
sprintf_s(buffer sizeof(buffer) “%.16g“ value);
#else
snprintf(buffer sizeof(buffer) “%.16g“ value);
#endif
buffer[sizeof(buffer) - 1] = 0;
JSONCPP_STRING s(buffer);
JSONCPP_STRING::size_type index = s.find_last_of(“eE“);
if (index != JSONCPP_STRING::npos) {
JSONCPP_STRING::size_type hasSign =
(s[index + 1] == ‘+‘ || s[index + 1] == ‘-‘) ? 1 : 0;
JSONCPP_STRING::size_type exponentStartIndex = index + 1 + hasSign;
JSONCPP_STRING normalized = s.substr(0 exponentStartIndex);
JSONCPP_STRING::size_type indexDigit =
s.find_first_not_of(‘0‘ exponentStartIndex);
JSONCPP_STRING exponent = “0“;
if (indexDigit != JSONCPP_STRING::npos) // There is an exponent different
// from 0
{
exponent = s.substr(indexDigit);
}
return normalized + exponent;
}
return s;
}
static JSONCPP_STRING readInputTestFile(const char* path) {
FILE* file = fopen(path “rb“);
if (!file)
return JSONCPP_STRING(““);
fseek(file 0 SEEK_END);
long const size = ftell(file);
unsigned long const usize = static_cast(size);
fseek(file 0 SEEK_SET);
JSONCPP_STRING text;
char* buffer = new char[size + 1];
buffer[size] = 0;
if (fread(buffer 1 usize file) == usize)
text = buffer;
fclose(file);
delete[] buffer;
return text;
}
static void printValueTree(FILE* fout
Json::Value& value
const JSONCPP_STRING& path = “.“) {
if (value.hasComment(Json::commentBefore)) {
fprintf(fout “%s\n“ value.getComment(Json::commentBefore).c_str());
}
switch (value.type()) {
case Json::nullValue:
fprintf(fout “%s=null\n“ path.c_str());
break;
case Json::intValue:
fprintf(fout “%s=%s\n“ path.c_str()
Json::valueToString(value.asLargestInt()).c_str());
break;
case Json::uintValue:
fprintf(fout “%s=%s\n“ path.c_str()
Json::valueToString(value.asLargestUInt()).c_str());
break;
case Json::realValue:
fprintf(fout “%s=%s\n“ pa
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2018-11-13 10:46 jsoncpp\1.8.4\
目录 0 2018-11-13 10:46 jsoncpp\1.8.4\include\
文件 113 2018-11-13 10:46 jsoncpp\1.8.4\include\CMakeLists.txt
目录 0 2018-12-12 13:17 jsoncpp\1.8.4\include\json\
文件 2499 2018-11-13 10:46 jsoncpp\1.8.4\include\json\allocator.h
文件 2644 2018-11-13 10:46 jsoncpp\1.8.4\include\json\assertions.h
文件 686 2018-11-13 10:46 jsoncpp\1.8.4\include\json\autoli
文件 6820 2018-11-13 10:46 jsoncpp\1.8.4\include\json\config.h
文件 1784 2018-11-13 10:46 jsoncpp\1.8.4\include\json\features.h
文件 782 2018-11-13 10:46 jsoncpp\1.8.4\include\json\forwards.h
文件 444 2018-11-13 10:46 jsoncpp\1.8.4\include\json\json.h
文件 13977 2018-11-13 10:46 jsoncpp\1.8.4\include\json\reader.h
文件 29382 2018-12-12 13:17 jsoncpp\1.8.4\include\json\value.h
文件 786 2018-11-13 10:46 jsoncpp\1.8.4\include\json\version.h
文件 12398 2018-11-13 10:54 jsoncpp\1.8.4\include\json\writer.h
目录 0 2018-11-13 09:25 jsoncpp\1.8.4\src\
目录 0 2018-11-13 09:29 jsoncpp\1.8.4\src\jsontestrunner\
文件 9871 2018-11-13 09:25 jsoncpp\1.8.4\src\jsontestrunner\main.cpp
目录 0 2018-12-12 14:01 jsoncpp\1.8.4\src\lib_json\
文件 59211 2018-11-13 09:25 jsoncpp\1.8.4\src\lib_json\json_reader.cpp
文件 3828 2018-11-13 09:25 jsoncpp\1.8.4\src\lib_json\json_tool.h
文件 52100 2018-12-12 14:01 jsoncpp\1.8.4\src\lib_json\json_value.cpp
文件 5272 2018-11-13 09:25 jsoncpp\1.8.4\src\lib_json\json_valueiterator.inl
文件 38028 2018-12-11 13:01 jsoncpp\1.8.4\src\lib_json\json_writer.cpp
文件 2646 2018-12-12 14:34 jsoncpp\1.8.4\src\lib_json\test.cpp
文件 826 2018-11-13 09:25 jsoncpp\1.8.4\src\lib_json\version.h.in
目录 0 2018-11-13 09:29 jsoncpp\1.8.4\src\test_lib_json\
文件 12767 2018-11-13 09:25 jsoncpp\1.8.4\src\test_lib_json\jsontest.cpp
文件 10819 2018-11-13 09:25 jsoncpp\1.8.4\src\test_lib_json\jsontest.h
文件 93546 2018-11-13 09:25 jsoncpp\1.8.4\src\test_lib_json\main.cpp
文件 76 2018-12-12 14:38 jsoncpp\编译说明.txt
............此处省略0个文件信息
相关资源
- SVN1.8.11中文语言包 TortoiseSVN-1.8.11.26
- geth-windows-amd64-1.8.2-b8b9f7f4.exe
- Termite最新版1.8.4.zip
-
chromeFOR.COM_vxg-media-pla
yer_v1.8.41 - pdfjs-1.8.188 pdf 在线浏览工具可以查看
- EPLAN_Fluid_1.8.4液压气动符号库.rar
- hdf5-1.8.11.tar.gz
- quartz-1.8.6完整内容
- jsoncpp-src-0.6.0-rc2
- cvsnt-2.5.03.2260.msi 和TortoiseCVS-1.8.26
- FlexGraphics_Full_1.8.zip
- MAC版uTorrent 1.8.7 43796 破解去广告版本
- GDAL,geos联合编译的库,版本为1.8.0
- SmartAdmin 最新 1.8.2 Angular 版
- synergy-v1.8.8-stable-linux & windows
- 迅雷V7.1.8.2302破解高速通道JayXon精简版
- JByteMod-Beta-1.8.2
- TortoiseSVN-1.8.10.26129-x64-svn-1.8.11 简体中
- SpeedPan1.8.1.137.zip
- 2217601TankWar1.8.14.rar
- tcpdump(tcpdump-4.9.2.tar.gz & libpcap-1.8.1
- 付费阅读小程序1.8.2开源
- Windows10+VS2015+PCL1.8.1配置
- Windows支持SSL的Haproxy 1.8.17
- gitblit-1.8.0.zip
- arduino-1.8.1-windows
- SVN1.8.8.25755客户端与汉化安装包
- Tortoise1.8.X对应的SVN1.10.X
- jre32 1.8.0_172 x32 库
- Final IK 1.8.unitypackage
评论
共有 条评论