资源简介
如何用Qt 的方法进行HTTP 请求下载文件,能够支持断点续传(断点续传即能够手动停止下载,下次可以从已经下载的部分开始继续下载未完成的部分,而没有必要从头开始上传下载),并且实时更新下载信息。整体代码考虑十分周到,对各种情况也做了相应的处理,并且有通俗易懂的注释。
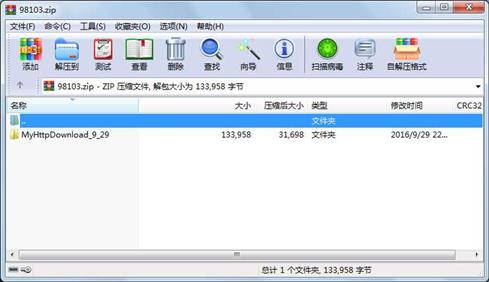
代码片段和文件信息
#include “downloadmanager.h“
#include
#include
#include
#include
#define DOWNLOAD_FILE_SUFFIX “_tmp“
DownLoadManager::DownLoadManager(Qobject *parent)
: Qobject(parent)
m_networkManager(NULL)
m_url(QUrl(““))
m_fileName(““)
m_isSupportBreakPoint(false)
m_bytesReceived(0)
m_bytesTotal(0)
m_bytesCurrentReceived(0)
m_isStop(true)
{
m_networkManager = new QNetworkAccessManager(this);
}
DownLoadManager::~DownLoadManager()
{}
// 设置是否支持断点续传;
void DownLoadManager::setDownInto(bool isSupportBreakPoint)
{
m_isSupportBreakPoint = isSupportBreakPoint;
}
QString DownLoadManager::getDownloadUrl()
{
return m_url.toString();
}
// 开始下载文件;
void DownLoadManager::downloadFile(QString url QString fileName)
{
// 防止多次点击开始下载按钮,进行多次下载,只有在停止标志变量为true时才进行下载;
if (m_isStop)
{
m_isStop = false;
m_url = QUrl(url);
// 从url 中获取文件名,但不是都有效;
// QFileInfo fileInfo(m_url.path());
// QString fileName = fileInfo.fileName();
// 将当前文件名设置为临时文件名,下载完成时修改回来;
m_fileName = fileName + DOWNLOAD_FILE_SUFFIX;
// 如果当前下载的字节数为0那么说明未下载过或者重新下载
// 则需要检测本地是否存在之前下载的临时文件,如果有则删除
if (m_bytesCurrentReceived <= 0)
{
removeFile(m_fileName);
}
QNetworkRequest request;
request.setUrl(m_url);
// 如果支持断点续传,则设置请求头信息;
if (m_isSupportBreakPoint)
{
QString strRange = QString(“bytes=%1-“).arg(m_bytesCurrentReceived);
request.setRawHeader(“Range“ strRange.toLatin1());
}
m_reply = m_networkManager->get(request);
connect(m_reply SIGNAL(downloadProgress(qint64 qint64)) this SLOT(onDownloadProgress(qint64 qint64)));
connect(m_reply SIGNAL(readyRead()) this SLOT(onReadyRead()));
connect(m_reply SIGNAL(finished()) this SLOT(onfinished()));
connect(m_reply SIGNAL(error(QNetworkReply::NetworkError)) this SLOT(onerror(QNetworkReply::NetworkError)));
}
}
// 下载进度信息;
void DownLoadManager::onDownloadProgress(qint64 bytesReceived qint64 bytesTotal)
{
if (!m_isStop)
{
m_bytesReceived = bytesReceived;
m_bytesTotal = bytesTotal;
// 更新下载进度;(加上 m_bytesCurrentReceived 是为了断点续传时之前下载的字节)
emit signalDownloadProcess(m_bytesReceived + m_bytesCurrentReceived m_bytesTotal + m_bytesCurrentReceived);
}
}
// 获取下载内容,保存到文件中;
void DownLoadManager::onReadyRead()
{
if (!m_isStop)
{
QFile file(m_fileName);
if (file.open(QIODevice::WriteOnly | QIODevice::Append))
{
file.write(m_reply->readAll());
}
file.close();
}
}
// 下载完成;
void DownLoadManager::onfinished()
{
m_isStop = true;
QVariant statusCode = m_reply->attribute(QNetworkRequest::HttpStatusCodeAttribute);
if (m_reply->error() == QNetworkReply::NoError)
{
// 重命名临时文件;
QFileInfo fileInfo(m_fileName);
if (fileInfo.exists())
{
int index = m_fileName.lastIndexOf(DOWNLOAD_FILE_SUFFIX);
QString realName = m_fileName.left(index);
QFile::rename(m_fileName realName);
}
}
else
{
// 有错误输
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2016-09-29 22:09 MyHttpDownload_9_29\
目录 0 2016-09-29 22:07 MyHttpDownload_9_29\MyHttpDownload\
文件 988 2016-09-09 10:38 MyHttpDownload_9_29\MyHttpDownload.sln
文件 33280 2016-09-29 22:09 MyHttpDownload_9_29\MyHttpDownload.v12.suo
目录 0 2016-09-29 22:09 MyHttpDownload_9_29\MyHttpDownload\Debug\
文件 4965 2016-09-29 20:15 MyHttpDownload_9_29\MyHttpDownload\downloadmanager.cpp
文件 1106 2016-09-29 20:17 MyHttpDownload_9_29\MyHttpDownload\downloadmanager.h
目录 0 2016-09-09 11:19 MyHttpDownload_9_29\MyHttpDownload\GeneratedFiles\
目录 0 2016-09-09 11:19 MyHttpDownload_9_29\MyHttpDownload\GeneratedFiles\Debug\
文件 7393 2016-09-29 22:07 MyHttpDownload_9_29\MyHttpDownload\GeneratedFiles\Debug\moc_downloadmanager.cpp
文件 4574 2016-09-29 22:07 MyHttpDownload_9_29\MyHttpDownload\GeneratedFiles\Debug\moc_myhttpdownload.cpp
文件 35549 2016-09-29 22:07 MyHttpDownload_9_29\MyHttpDownload\GeneratedFiles\qrc_myhttpdownload.cpp
目录 0 2016-09-09 10:38 MyHttpDownload_9_29\MyHttpDownload\GeneratedFiles\Release\
文件 9612 2016-09-29 22:07 MyHttpDownload_9_29\MyHttpDownload\GeneratedFiles\ui_myhttpdownload.h
文件 744 2016-09-28 08:53 MyHttpDownload_9_29\MyHttpDownload\main.cpp
文件 5630 2016-09-29 22:07 MyHttpDownload_9_29\MyHttpDownload\myhttpdownload.cpp
文件 878 2016-09-29 14:04 MyHttpDownload_9_29\MyHttpDownload\myhttpdownload.h
文件 156 2016-09-29 14:48 MyHttpDownload_9_29\MyHttpDownload\myhttpdownload.qrc
文件 6026 2016-09-29 14:52 MyHttpDownload_9_29\MyHttpDownload\myhttpdownload.ui
文件 12900 2016-09-09 11:19 MyHttpDownload_9_29\MyHttpDownload\MyHttpDownload.vcxproj
文件 3213 2016-09-09 11:19 MyHttpDownload_9_29\MyHttpDownload\MyHttpDownload.vcxproj.filters
文件 677 2016-09-29 22:07 MyHttpDownload_9_29\MyHttpDownload\MyHttpDownload.vcxproj.user
目录 0 2016-09-29 14:48 MyHttpDownload_9_29\MyHttpDownload\Resources\
文件 3197 2016-09-29 14:47 MyHttpDownload_9_29\MyHttpDownload\Resources\progressbar.png
文件 3070 2016-09-29 14:46 MyHttpDownload_9_29\MyHttpDownload\Resources\progressbar_back.png
目录 0 2016-09-09 11:19 MyHttpDownload_9_29\Win32\
目录 0 2016-09-29 22:09 MyHttpDownload_9_29\Win32\Debug\
- 上一篇:计算机组成原理期末考试试题及答案筛选.doc
- 下一篇:Qt 之 实现简单截图功能一
相关资源
- 酒店管理系统基于Qt Creator5)
- vtk QT做的三维地质可视化系统2of2
- Qt局域网聊天软件
- Qt Creator opengl实现四元数鼠标控制轨迹
- QT局域网聊天系统(基于QT5.修改过)
- qt-电子点菜系统
- C 餐厅叫号系统(QT平)
- QT 实现文件下载
- qt图像处理
- QT,JPEG解码源代码(已完成)
- Qt 播放音频文件
- Qt 读取16进制的data文件
- MQTT+串口(usart)透传
- 易语言QQTEA算法源码
- VC工程转Qt工程文件的工具
- MQTT推送Demo
- 基于Linux、QT的视频监控系统的设计与
- Qt 百度地图 定位
- QT酷炫界面开发指南《QmlBook》
- Ubuntu下操作Excel,qt代码
- Qt图片浏览器 --基于Qt的Graphics View f
- qtnribbon2破解
- Qt软件开发 完整项目代码
- MQTT_3.1protocol_Specific中文版
- 在QT中使用RTP进行视频的采集和传输
- Mini6410 Qt4和Qtopia编程开发指南
- Qt实现Code39条形码
- qt_ffmpeg_mp4_export_and_import.zip
- QT5.5入门与项目实战
- Huffman Compress 霍夫曼编码 压缩 解压缩
评论
共有 条评论