资源简介
openCL编程指南 随书源代码
openCL programming Guide code
OpenCL领域公认的权威著作,由OpenCL核心设计人员亲自执笔,不仅全面而深刻地解读了OpenCL规范和编程模型,而且通过大量案例和代码演示了基于OpenCL编写并行程序和实现各种并行算法的原理、方法、流程和最佳实践,以及如何对OpenCL进行性能优化,如何对硬件进行探测和调整。
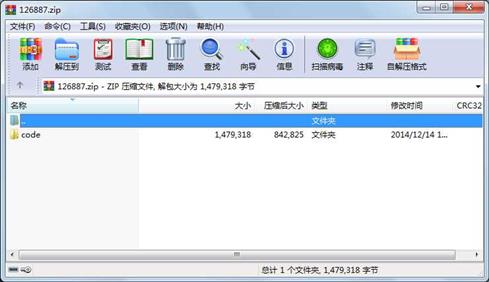
代码片段和文件信息
//
// Book: OpenCL(R) Programming Guide
// Authors: Aaftab Munshi Benedict Gaster Timothy Mattson James Fung Dan Ginsburg
// ISBN-10: 0-321-74964-2
// ISBN-13: 978-0-321-74964-2
// Publisher: Addison-Wesley Professional
// URLs: http://safari.informit.com/9780132488006/
// http://www.openclprogrammingguide.com
//
// GLinterop.cpp
//
// This is a simple example that demonstrates basic OpenCL setup and
// use.
#include
#include
#include
#ifdef __APPLE__
#include
#else
#include
#include
#endif
#ifdef _WIN32
#include
#endif
#include
#include
#ifdef __GNUC__
#include
#endif
///
// OpenGL/CL variables objects
//
GLuint tex = 0;
GLuint vbo = 0;
int vbolen;
int imWidth = 0;
int imHeight = 0;
cl_kernel kernel = 0;
cl_kernel tex_kernel = 0;
cl_mem cl_vbo_mem cl_tex_mem;
cl_context context = 0;
cl_command_queue commandQueue = 0;
cl_program program = 0;
///
// Forward declarations
void Cleanup();
cl_int computeVBO();
cl_int computeTexture();
///
// Render the vertex buffer object (VBO) contents
//
void renderVBO( int vbolen )
{
glColor3f(1.0f 1.0f 1.0f);
glLineWidth(2.0f);
// Draw VBO containing the point list coordinates to place GL_POINTS at feature locations
// bind VBOs for vertex array and index array
glBindBufferARB(GL_ARRAY_BUFFER_ARB vbo); // for vertex coordinates
glEnableClientState(GL_VERTEX_ARRAY); // activate vertex coords array
glVertexPointer( 2 GL_FLOAT 0 0 );
// draw lines with endpoints given in the array
glDrawArrays(GL_LINES 0 vbolen*2);
glDisableClientState(GL_VERTEX_ARRAY); // deactivate vertex array
// bind with 0 so switch back to normal pointer operation
glBindBufferARB(GL_ARRAY_BUFFER_ARB 0);
}
///
// Display the texture in the window
//
void displayTexture(int w int h)
{
glEnable(GL_TEXTURE_RECTANGLE_ARB);
glBindTexture(GL_TEXTURE_RECTANGLE_ARB tex );
glBegin(GL_QUADS);
glTexCoord2f(0 0);
glVertex2f(0 0);
glTexCoord2f(0 h);
glVertex2f(0 h);
glTexCoord2f(w h);
glVertex2f(w h);
glTexCoord2f(w 0);
glVertex2f(w 0);
glEnd();
glDisable(GL_TEXTURE_RECTANGLE_ARB);
}
void reshape(int width int height)
{
glViewport( 0 0 width height );
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0widthheight0);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
///
// Main rendering call for the scene
//
void renderScene(void)
{
glClearColor(0.0f 0.0f 1.0f 1.0f );
glClear( GL_COLOR_BUFFER_BIT );
computeTexture();
computeVBO();
displayTexture(imWidthimHeight);
renderVBO( vbolen );
glutSwapBuffers();
}
///
// Keyboard events handler
//
void KeyboardGL(unsigned char key int x int y)
{
switch(key)
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2014-12-14 17:58 code\
文件 342 2014-12-14 17:58 code\CMakeLists.txt
目录 0 2014-12-14 17:57 code\cmake\
文件 1491 2014-12-14 17:56 code\cmake\FindDirectX.cmake
文件 1768 2014-12-14 17:57 code\cmake\FindGLEW.cmake
文件 3555 2014-12-14 17:57 code\cmake\FindOpenCL.cmake
目录 0 2014-12-14 17:54 code\khronos\
目录 0 2014-12-14 17:59 code\khronos\CL\
文件 112900 2014-12-14 17:59 code\khronos\CL\cl.hpp
文件 4859 2014-12-14 17:59 code\khronos\CL\cl_d3d10.h
目录 0 2014-12-14 18:39 code\src\
文件 463 2014-12-14 18:39 code\src\CMakeLists.txt
目录 0 2014-12-14 18:10 code\src\Chapter_10\
目录 0 2014-12-14 18:11 code\src\Chapter_10\GLinterop\
文件 839 2014-12-14 18:10 code\src\Chapter_10\GLinterop\CMakeLists.txt
文件 733 2014-12-14 18:11 code\src\Chapter_10\GLinterop\GLinterop.cl
文件 18429 2014-12-14 18:11 code\src\Chapter_10\GLinterop\GLinterop.cpp
目录 0 2014-12-14 18:12 code\src\Chapter_11\
目录 0 2014-12-14 18:13 code\src\Chapter_11\D3Dinterop\
文件 664 2014-12-14 18:12 code\src\Chapter_11\D3Dinterop\CMakeLists.txt
文件 668 2014-12-14 18:12 code\src\Chapter_11\D3Dinterop\D3Dinterop.cl
文件 25008 2014-12-14 18:12 code\src\Chapter_11\D3Dinterop\D3Dinterop.cpp
文件 1381 2014-12-14 18:13 code\src\Chapter_11\D3Dinterop\D3Dinterop.fx
目录 0 2014-12-14 17:55 code\src\Chapter_12\
目录 0 2014-12-14 18:14 code\src\Chapter_12\Sinewave\
文件 172 2014-12-14 18:13 code\src\Chapter_12\Sinewave\CMakeLists.txt
文件 991 2014-12-14 18:14 code\src\Chapter_12\Sinewave\sinewave.cl
文件 13584 2014-12-14 18:14 code\src\Chapter_12\Sinewave\sinewave.cpp
目录 0 2014-12-14 18:15 code\src\Chapter_12\VectorAdd\
文件 88 2014-12-14 18:14 code\src\Chapter_12\VectorAdd\CMakeLists.txt
文件 4597 2014-12-14 18:15 code\src\Chapter_12\VectorAdd\vecadd.cpp
............此处省略67个文件信息
- 上一篇:qt activemq mqtt 动态库
- 下一篇:实用取色器工具.zip
相关资源
- opencl编程指南随书代码
- OPENCL编程指南随书源码
- x264源码及其配置文件,用于配置树莓
- 论文研究 - 采用多GPU计算的陡峭三维
- 利用GPU破解rar密码工具
- GPU精粹1-中文版.pdf《GPU精粹:实时图形
- eetop.cn_OpenCL.Parallel Computing on the GPU
- GPU+编程与CG+语言之阳春白雪下里巴人
- MD5GPU.rar
- 数据融合代码-ESTARFM
- GPU高性能运算之CUDA源代码
- win10 vs2015 编译nms和gpunms
- GPU结构概述
- OpenCL48_CN.pdf
- GPU编程与CG语言之阳春白雪下里巴人
- NVIDIA GPU Computing SDK
- 《GPU高性能计算之CUDA》书中源代码
- 基于D3D的YV12视频渲染 更新
- _CPU_GPU协同并行计算研究综述_cuda_op
- GPU编程与CG语言之阳春白雪下里巴人
- GPU gems 1 pdf
- amd中文opencl教程
- matconvnet的gpu编译版本(cuda7.5)
- OpenCL规范1.2正体中文版 beta1
- 英伟达显卡虚拟化部署手册(M60 vGP
- GPUImage多种滤镜的使用示范以及美颜滤
- 基于OpenCL并行加速算法研究及其FPGA实
- CUDA && GPU 数据传输测试
- 国外CPU_GPU_FPGA性能测试比较结果
- OpenCL中文入门完整教程
评论
共有 条评论