资源简介
哈工大计算机系统实验八动态内存分配器 实验报告和代码
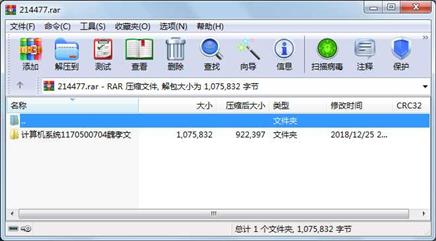
代码片段和文件信息
/*
* mm-naive.c - The fastest least memory-efficient malloc package.
*
* In this naive approach a block is allocated by simply incrementing
* the brk pointer. A block is pure payload. There are no headers or
* footers. Blocks are never coalesced or reused. Realloc is
* implemented directly using mm_malloc and mm_free.
*
* NOTE TO STUDENTS: Replace this header comment with your own header
* comment that gives a high level description of your solution.
*/
#include
#include
#include
#include
#include
#include “mm.h“
#include “memlib.h“
/*********************************************************
* NOTE TO STUDENTS: Before you do anything else please
* provide your team information in the following struct.
********************************************************/
team_t team = {
/* Team name */
“weixiaowen“
/* First member‘s full name */
“weixiaowen“
/* First member‘s email address */
“706461857@qq.com“
/* Second member‘s full name (leave blank if none) */
““
/* Second member‘s email address (leave blank if none) */
““
};
/* $begin mallocmacros */
/* Basic constants and macros */
#define WSIZE 4 /* word size (bytes) */
#define DSIZE 8 /* doubleword size (bytes) */
#define CHUNKSIZE (1<<12) /* initial heap size (bytes) */
#define OVERHEAD 8 /* overhead of header and footer (bytes) */
#define MAX(x y) ((x) > (y)? (x) : (y))
/* Pack a size and allocated bit into a word */
#define PACK(size alloc) ((size) | (alloc))
/* Read and write a word at address p */
#define GET(p) (*(size_t *)(p))
#define PUT(p val) (*(size_t *)(p) = (val))
/* Read the size and allocated fields from address p */
#define GET_SIZE(p) (GET(p) & ~0x7)
#define GET_ALLOC(p) (GET(p) & 0x1)
/* Given block ptr bp compute address of its header and footer */
#define HDRP(bp) ((char *)(bp) - WSIZE)
#define FTRP(bp) ((char *)(bp) + GET_SIZE(HDRP(bp)) - DSIZE)
/* Given block ptr bp compute address of next and previous blocks */
#define NEXT_BLKP(bp) ((char *)(bp) + GET_SIZE(((char *)(bp) - WSIZE)))
#define PREV_BLKP(bp) ((char *)(bp) - GET_SIZE(((char *)(bp) - DSIZE)))
/* $end mallocmacros */
/* Global variables */
static char *heap_listp; /* pointer to first block */
#ifdef NEXT_FIT
static char *rover; /* next fit rover */
#endif
/* function prototypes for internal helper routines */
static void *extend_heap(size_t words);
static void place(void *bp size_t asize);
static void *find_fit(size_t asize);
static void *coalesce(void *bp);
static void printblock(void *bp);
static void checkblock(void *bp);
/*
* mm_init - Initialize the memory manager
*/
/* $begin mminit */
int mm_init(void)
{
/* create the initial empty heap */
if ((heap_listp = mem_sbrk(4*WSIZE)) == NULL)
return -1;
PUT(heap_listp 0); /* alignment padding */
PUT(heap_listp+WSIZE P
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 792556 2018-12-24 23:14 计算机系统1170500704魏孝文\1170500704魏孝文.pdf
文件 273920 2018-12-24 23:13 计算机系统1170500704魏孝文\HITICS-lab8实验报告1170500704魏孝文.doc
文件 9356 2018-12-11 21:23 计算机系统1170500704魏孝文\mm.c
目录 0 2018-12-25 21:26 计算机系统1170500704魏孝文
----------- --------- ---------- ----- ----
1075832 4
相关资源
- 信号奇异点Lipschitz指数计算
- IBM Rational Software Architect 9.0破解文件
- Born-Infeld-dilaton-Lifshitz全息超导体的光
- 具有非线性电动力学的Lifshitz黑洞中铁
- The Existence of Optimal Control for Fully Cou
- Game Engine Architecture游戏引擎架构.pdf
- csapp
- UPnP-av-AVArchitecture-v1-中文
- Beyond Software Architecture - Creating and Su
- 12 More Essential Skills for Software Architec
- CSAPP bomblab实验报告
- csapp第三版蓝光版
- 一篇自动白平衡论文,论述了多种A
- ibm的Rational System Architect使用指导ppt
-
em
bedded Deep Learning_ Algorithms Architec - Executable UML: A Foundation for Model-Driven
- Computer Organization and Architecture 第8版答
- Deep Learning for Computer Architects 无水印
- Software Architecture in Practice(3rd) 无水
- Monitoring with Graphite azw3
- Neo4j-ai-graph-technology-white-paper-EN-A4-CN
- arm v8 体系架构 官方手册
- 贝尔链白皮书1.2_PC-中文
- 高级FPGA设计结构、实现和优化(英文
- Computer Architecture A Quantitative Approach
- CSAPP课件-中科大
- App Architecture
- Libra_WhitePaperV2_April2020.pdf
- automotive electronic architectures of integra
- The Architecture of Open Source Applications 2
评论
共有 条评论