资源简介
用于图像风格转化(image style transfer)的代码实现。
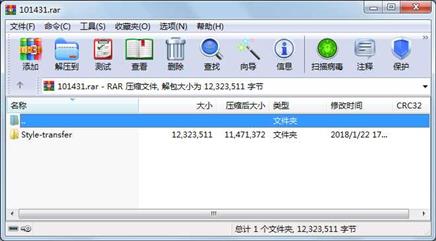
代码片段和文件信息
# # style Transfer
# In this notebook we will implement the style transfer technique from [“Image style Transfer Using Convolutional Neural Networks“ (Gatys et al. CVPR 2015)](http://www.cv-foundation.org/openaccess/content_cvpr_2016/papers/Gatys_Image_style_Transfer_CVPR_2016_paper.pdf).
#
# The general idea is to take two images and produce a new image that reflects the content of one but the artistic “style“ of the other. We will do this by first formulating a loss function that matches the content and style of each respective image in the feature space of a deep network and then performing gradient descent on the pixels of the image itself.
#
# The deep network we use as a feature extractor is [SqueezeNet](https://arxiv.org/abs/1602.07360) a small model that has been trained on ImageNet. You could use any network but we chose SqueezeNet here for its small size and efficiency.
#
# Here‘s an example of the images you‘ll be able to produce by the end of this notebook:
#
# 
#
#
# Set up
from scipy.misc import imread imresize
import numpy as np
import os
from scipy.misc import imread
import matplotlib.pyplot as plt
# Helper functions to deal with image preprocessing
from cs231n.image_utils import load_image preprocess_image deprocess_image
from cs231n.classifiers.squeezenet import SqueezeNet
import tensorflow as tf
def get_session():
“““Create a session that dynamically allocates memory.“““
# See: https://www.tensorflow.org/tutorials/using_gpu#allowing_gpu_memory_growth
config = tf.ConfigProto()
config.gpu_options.allow_growth = True
session = tf.Session(config=config)
return session
def rel_error(xy):
return np.max(np.abs(x - y) / (np.maximum(1e-8 np.abs(x) + np.abs(y))))
# Older versions of scipy.misc.imresize yield different results
# from newer versions so we check to make sure scipy is up to date.
def check_scipy():
import scipy
vnum = int(scipy.__version__.split(‘.‘)[1])
assert vnum >= 16 “You must install SciPy >= 0.16.0 to complete this notebook.“
check_scipy()
# Load the pretrained SqueezeNet model. This model has been ported from PyTorch see ‘cs231n/classifiers/squeezenet.py‘ for the model architecture.
#
# To use SqueezeNet you will need to first **download the weights** by changing into the ‘cs231n/datasets‘ directory and running ‘get_squeezenet_tf.sh‘ . Note that if you ran ‘get_assignment3_data.sh‘ then SqueezeNet will already be downloaded.
tf.reset_default_graph() # remove all existing variables in the graph
sess = get_session() # start a new Session
# Load pretrained SqueezeNet model
SAVE_PATH = ‘cs231n/datasets/squeezenet_tf/squeezenet.ckpt‘
# if not os.path.exists(SAVE_PATH):
# raise ValueError(“You need to download SqueezeNet!“)
model = SqueezeNet(save_path=SAVE_PATH sess=sess)
# Load data for testing
content_img_test = preprocess_image(load_image(‘styles/
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 9792 2018-01-22 17:45 st
文件 4741 2017-05-18 09:46 st
文件 3628 2018-01-22 17:42 st
文件 4941984 2017-05-04 11:57 st
文件 2293 2017-05-04 11:57 st
文件 5060877 2017-05-04 11:57 st
文件 2627 2017-12-26 14:33 st
文件 66274 2017-05-18 09:46 st
文件 66274 2017-05-18 09:46 st
文件 22417 2018-01-06 18:10 st
文件 202426 2017-05-18 09:46 st
文件 703587 2017-05-18 09:46 st
文件 613337 2017-05-18 09:46 st
文件 216723 2017-05-18 09:46 st
文件 406531 2017-05-18 09:46 st
目录 0 2018-01-22 17:42 st
目录 0 2018-01-22 17:38 st
目录 0 2018-01-22 17:42 st
目录 0 2018-01-22 17:38 st
目录 0 2018-01-22 17:45 st
目录 0 2018-01-22 17:44 st
目录 0 2018-01-22 17:38 st
目录 0 2018-01-22 17:45 st
----------- --------- ---------- ----- ----
12323511 23
相关资源
- 基于MFC扩展CListCtrl子项显示图片并叠
- img写盘工具(roadkil‘s diskimage) v1.
- 第三方控减
- cximage的linux版本源码
- Cocos2d-x 3.x 头像选择器功能扩展Image
- Facile hydrothermal synthesis of Tb2(MoO4)
-
易语言GDI自绘电梯st
yle源码 - PCNN TOOLBOX
- 双立方插值实现
- 图像处理 分析与机器视觉 源码
- 脉冲耦合神经网络工具箱PCNN-toolbox
- Image2Lcd+汉字取模,TFT助手
- PNG图片转Delphi中Image.Picture.data代码-工
- CImage 强大的图像处理类库
- 微软内部镜像封装工具:CDIMAGE 2.54 (版
- Modeling of rapeseed at maturity stage using 3
- ImageWatch2019.vsix
- Image Resizer
- swift-PSImageEditors一个简而至美的图片编
- ImageConverter(万能图片转换器)2009免
- ZedBoard REV_D的BOOT.BIN、devicetree.dtb、l
- Image Super-Resolution Via Sparse Representati
- Qt Openglwidget 显示图片纹理贴图
- 2-d and 3-d Image Registration: for Medical Re
- Universalimageloader
- stb_image库
- Markov random fields for vision and image proc
- 《图像分析中的马尔可夫随机场模型
- Nano/Microscale Heat Transfer
- NeatImagePS MAC
评论
共有 条评论