资源简介
操作系统实验
1、先来先服务
2、时间片轮转
3、最短作业优先
4、最短剩余时间优先
5、非抢占的优先级调度
6、可抢占的优先级调度
7、高响应比调度
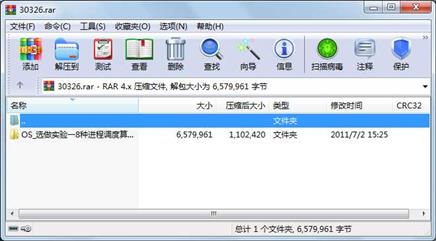
代码片段和文件信息
// Process Scheduling.cpp : Defines the entry point for the console application.
//
/*----------------------进程调度模拟程序--------------------*/
/* */
/* 姓名:万妮娜 学号:09301049 */
/* 2011.6.12 */
/* */
/*----------------------------------------------------------*/
#include “stdafx.h“
#include
#include
#include
#include
#include
#define inFileName “Input.txt“
#define outFileName “Output.txt“
//队列结点的定义
typedef struct PCBNode{
int proID;//进程序号
int arriveTime;//进程到达时间
int burstTime;//进程请求时间
int prior;//进程优先级
int finishTime;
float rate;//记录响应比
bool first;
bool tag;//标记是否已出等待队列
PCBNode* next;
} PCB;
PCB* frontw = NULL;
PCB* rearw = NULL;
PCB* frontr = NULL;
PCB* rearr = NULL;
int totalTime = 0;//已经过去的总时间
int totalWaitTime = 0;//总的等待时间
int totalTurnAroundTime = 0; //总的周转时间
int totalFinishTime = 0;
int proNum = 0;//进程总数
int wayNum = 0;//算法序号
bool rfirst = true;
/*----------------进入等待队列函数-----------------*/
void EnWaitQueue(PCB* p){
rearw->next = p;
rearw = p;
}
/*----------------进入就绪队列函数-----------------*/
void EnReadyQueue(PCB* p){
rearr->next = p;
rearr = p;
}
/*----------------出等待序列函数-----------------*/
bool DeWaitQueue(PCB &p){
if(frontw != rearw){
p = *(frontw->next);
frontw = frontw->next;
return true;
}else{
return false;
}
}
/*----------------出就绪序列函数-----------------*/
bool DeReadyQueue(PCB &p){
if(frontr != rearr){
p = *(frontr->next);
frontr = frontr->next;
return true;
}else{
return false;
}
}
/*----------------判断就绪队列是否为空-------------------*/
bool isReadyQueueEmpty(){
if(frontr == rearr){
return true;
}
return false;
}
/*---------判断等待队列中的进程是否全部进入就绪队列--------*/
bool AllWaitOut(){
PCB* p = (PCB*)malloc(sizeof(PCB));
p = frontw;
for(int i=0;i p=p->next;
if(!(p->tag)){//如果P没有移出了等待队列
return false;
}
}
return true;
}
/*----------------取等待队列队头元素-------------*/
bool GetWaitHead(PCB &p){
if(frontw != rearw){
p = *(frontw->next);
return true;
}else{
return false;
}
}
/*----------------取就绪队列队头元素-------------*/
bool GetReadyHead(PCB &p){
if(frontr != rearr){
p = *(frontr->next);
return true;
}else{
return false;
}
}
/*----------------读取进程数-----------------*/
void countProNum(){
std::ifstream inc;
std::string temp;
inc.open(inFileName);
while(getline(inctemp)){
proNum++;
}
inc.close();
}
/*------------读取进程信息构建等待序列----------*/
void readFile(){
std::ifstream inr;
inr.open(inFileName);
frontw = rearw = (PCB*)malloc(sizeof(PCB));
for(int i = 0;i PCB* temp = (PCB*)malloc(sizeof(PCB));
inr>>temp->proID;
inr>>temp->arriv
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 93184 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Debug\Process Scheduling.exe
文件 451480 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Debug\Process Scheduling.ilk
文件 732160 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Debug\Process Scheduling.pdb
文件 14024 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\BuildLog.htm
文件 67 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\mt.dep
文件 663 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\Process Scheduling.exe.em
文件 728 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\Process Scheduling.exe.em
文件 621 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\Process Scheduling.exe.intermediate.manifest
文件 204602 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\Process Scheduling.obj
文件 3211264 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\Process Scheduling.pch
文件 12657 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\stdafx.obj
文件 191488 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\vc90.idb
文件 299008 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug\vc90.pdb
文件 41 2011-05-04 20:20 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Input.txt
文件 934 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Output.txt
文件 31319 2011-06-12 09:45 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Process Scheduling.cpp
文件 4548 2011-05-04 19:34 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Process Scheduling.vcproj
文件 1415 2011-06-12 09:54 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Process Scheduling.vcproj.2010-VAIO.Administrator.user
文件 1368 2011-05-04 19:34 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\ReadMe.txt
文件 305 2011-05-04 19:34 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\stdafx.cpp
文件 320 2011-05-04 19:34 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\stdafx.h
文件 765 2011-05-04 19:34 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\targetver.h
文件 1313792 2011-06-12 09:54 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling.ncb
文件 920 2011-05-04 19:34 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling.sln
..A..H. 12288 2011-06-12 09:54 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling.suo
目录 0 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling\Debug
目录 0 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Debug
目录 0 2011-06-12 09:52 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling\Process Scheduling
目录 0 2011-06-12 09:53 OS_选做实验一8种进程调度算法模拟实现_09301049\Process Scheduling
目录 0 2011-07-02 15:25 OS_选做实验一8种进程调度算法模拟实现_09301049
............此处省略3个文件信息
- 上一篇:InnovaDSXP.OCX
- 下一篇:JPEG标准文档itu-t81
相关资源
- 操作系统实验——虚存管理实验
- 广工操作系统实验
- 广东工业大学操作系统实验四文件系
- Linux 操作系统实验(全)
- 操作系统实验综合设计【附代码】
- 湖南大学操作系统实验报告
- 操作系统实验报告哲学家就餐问题、
- 操作系统实验 广东工业大学[代码+文
- 西北农林科技大学操作系统实验一-
- 广东工业大学操作系统实验源码
- 广州大学--操作系统实验1-5实验报告
- Nachos进程调度 算法修改
- 操作系统实验指导
- 操作系统 进程调度
- 操作系统实验,生产者与消费者问题
- 操作系统实验进程的创建源代码及文
- 上海大学操作系统2实验报告
- UCOSII实时操作系统实验
- 山东大学操作系统实验报告
- NachOS 进程调度算法修改
- 操作系统实验完整版川大计科
- 杭电操作系统实验一--linux内核编译添
- 操作系统实验指导——基于Linux内核第
- 操作系统实验指导——基于Linux内核完
- 电子科技大学计算机操作系统实验代
- 计算机操作系统实验(5个详细实验)
- 广东工业大学操作系统实验程序及报
- 采用时间片轮转算法的进程调度程序
- 综合使用作业调度和进程调度模拟作
- 操作系统实验磁盘调度
评论
共有 条评论