资源简介
第15章 粒子系统
1、SprayParticles
演示粒子系统的具体实现,粒子系统类的封装。
2、ComplexSample
一个综合性的示例程序,演示了摄影机类和场景漫游的实现,以及静态网格模型、动画网格模型、粒子系统的进一步封装和使用。
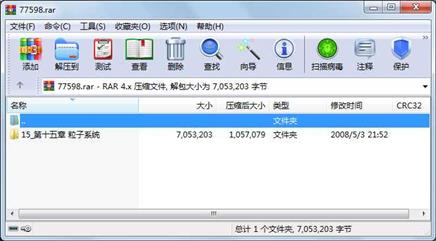
代码片段和文件信息
//=============================================================================
// Desc: 喷洒粒子系统类源文件
//=============================================================================
#include “dxstdafx.h“
#include “ParticleSystem.h“
//-----------------------------------------------------------------------------
// Desc: 构造函数
//-----------------------------------------------------------------------------
CParticleSystem::CParticleSystem( DWORD dwFlush DWORD dwDiscard)
{
m_dwbase = dwDiscard;
m_dwFlush = dwFlush;
m_dwDiscard = dwDiscard;
m_dwParticles = 0;
m_dwParticlesLim = 1000;
m_pParticles = NULL;
m_pParticlesFree = NULL;
m_pVB = NULL;
m_pTexture = NULL;
}
//-----------------------------------------------------------------------------
// Desc: 析构函数
//-----------------------------------------------------------------------------
CParticleSystem::~CParticleSystem()
{
while( m_pParticles )
{
PARTICLE* pSpark = m_pParticles;
m_pParticles = pSpark->m_pNext;
delete pSpark;
}
while( m_pParticlesFree )
{
PARTICLE *pSpark = m_pParticlesFree;
m_pParticlesFree = pSpark->m_pNext;
delete pSpark;
}
}
//-----------------------------------------------------------------------------
// Desc: 创建粒子纹理
//-----------------------------------------------------------------------------
HRESULT CParticleSystem::Create( LPDIRECT3DDEVICE9 pd3dDevice )
{
HRESULT hr;
V_RETURN(D3DXCreateTextureFromFile(pd3dDevice L“particle.bmp“ &m_pTexture));
return S_OK;
}
//-----------------------------------------------------------------------------
// Desc: 创建保存粒子的顶点缓冲区
//-----------------------------------------------------------------------------
HRESULT CParticleSystem::Restore( LPDIRECT3DDEVICE9 pd3dDevice )
{
HRESULT hr;
V_RETURN(pd3dDevice->CreateVertexBuffer( m_dwDiscard *
sizeof(POINTVERTEX) D3DUSAGE_DYNAMIC | D3DUSAGE_WRITEONLY | D3DUSAGE_POINTS
POINTVERTEX::FVF D3DPOOL_DEFAULT &m_pVB NULL ));
return S_OK;
}
//-----------------------------------------------------------------------------
// Desc: 更新当前粒子的属性
//-----------------------------------------------------------------------------
HRESULT CParticleSystem::Update( float fSecsPerframe)
{
PARTICLE *pParticle **ppParticle;
static float fTime = 0.0f;
fTime += fSecsPerframe;
//更新已存在粒子的属性值
ppParticle = &m_pParticles; //粒子链表
while( *ppParticle )
{
pParticle = *ppParticle; //取出当前粒子
//计算粒子的新位置
float fT = fTime - pParticle->m_fTime0; //当前粒子已存活的时间
float fGravity = -9.8f;
pParticle->m_vPos = pParticle->m_vVel0 * fT + pParticle->m_vPos0;
pParticle->m_vPos.y += (0.5f * fGravity) * (fT * fT);
pParticle->m_vVel.y = pParticle->m_vVel0.y + fGravity * fT;
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 25214 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\directx.ico
文件 365 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\dxstdafx.cpp
文件 3725 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\dxstdafx.h
文件 232916 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUT.cpp
文件 12597 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUT.h
文件 32543 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTenum.cpp
文件 7796 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTenum.h
文件 290442 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTgui.cpp
文件 49206 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTgui.h
文件 36086 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTMesh.cpp
文件 5575 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTMesh.h
文件 132261 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTmisc.cpp
文件 45173 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTmisc.h
文件 844456 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTRes.cpp
文件 602 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTRes.h
文件 53453 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTSettingsDlg.cpp
文件 5255 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTSettingsDlg.h
文件 55271 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTsound.cpp
文件 5936 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUTsound.h
文件 902 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUT_2003.sln
文件 3775 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUT_2003.vcproj
文件 872 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUT_2005.sln
文件 4429 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\Common\DXUT_2005.vcproj
文件 1142 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\particle.bmp
文件 9516 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\ParticleSystem.cpp
文件 2010 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\ParticleSystem.h
文件 457 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\resource.h
文件 16030 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\SprayParticles.cpp
文件 483328 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\SprayParticles.exe
文件 32027 2007-04-02 16:59 15_第十五章 粒子系统\01_SprayParticles\SprayParticles.jpg
............此处省略69个文件信息
评论
共有 条评论