资源简介
source code for Computer Graphics: Programming in OpenGL for Visual Communication,Steve Cunningham,计算机图形学(Steve Cunningham)一书源代码
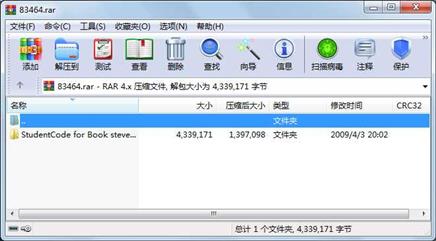
代码片段和文件信息
/*
Program to draw and animate a hypercube to illustrate higher-dimensional issues
Source file to be used with
Cunningham Computer Graphics: Programming in OpenGL for Visual Communication Prentice-Hall 2007
Intended for class use only
*/
#include
#include
#include
#include
// definitions of parameters for program
#define VCOUNT 16
#define ECOUNT 32
#define EPSILON .01570795 // PI/200 -- for the animate function
// vertex data
GLfloat vertices[VCOUNT][4] = { { 1.0 1.0 1.0 1.0 } // 0
{-1.0 1.0 1.0 1.0 } // 1
{ 1.0-1.0 1.0 1.0 } // 2
{-1.0-1.0 1.0 1.0 } // 3
{ 1.0 1.0-1.0 1.0 } // 4
{-1.0 1.0-1.0 1.0 } // 5
{ 1.0-1.0-1.0 1.0 } // 6
{-1.0-1.0-1.0 1.0 } // 7
{ 1.0 1.0 1.0-1.0 } // 8
{-1.0 1.0 1.0-1.0 } // 9
{ 1.0-1.0 1.0-1.0 } // 10
{-1.0-1.0 1.0-1.0 } // 11
{ 1.0 1.0-1.0-1.0 } // 12
{-1.0 1.0-1.0-1.0 } // 13
{ 1.0-1.0-1.0-1.0 } // 14
{-1.0-1.0-1.0-1.0 } }; // 15
// edge data -- the edges aren‘t oriented because we don‘t do faces
// yet. If we do we‘ll need to re-do this and add a face list
int edges[ECOUNT][2] = {{ 0 1}{ 0 2}{ 0 4}{ 1 3}{ 1 5}{ 2 3} // 3D cube
{ 2 6}{ 3 7}{ 4 5}{ 4 6}{ 5 7}{ 6 7} // W=0
{ 8 9}{ 810}{ 812}{ 911}{ 913}{1011} // 3D cube
{1014}{1115}{1213}{1214}{1315}{1415} // W=1
{ 0 8}{ 1 9}{ 210}{ 311}{ 412} // between
{ 513}{ 614}{ 715} }; // cubes
// data for interaction
GLint Tag1=1 Tag2=2 Tag3=3 Tag4=4 Tag5=5 Tag6=6 Tag7=7 Tag8=8 Tag9=9 selection;
GLfloat smallSine smallCosine;
GLfloat ep = 5.0; // eye point variable
static char ch; // character from keyboard that controls the rotation
typedef GLfloat point3[3];
GLfloat xAngle = 0. yAngle = 0. zAngle = 0.;
// function prototypes
void myinit(void);
void drawHyper(void);
void display(void);
void reshape(int int);
void keyboard(unsigned char int int);
void animate( void );
void myinit(void)
{
// set up overall light data including specular=ambient=light colors
GLfloat light_position[4]={ 10.0 10.0 -10.0 1.0 };
GLfloat light_color[4]={ 1.0 1.0 1.0 1.0 };
GLfloat ambient_color[4]={ 0.2 0.2 0.2 1.0 };
GLfloat specular_color[4]={ 1.0 1.0 1.0 1.0 };
GLfloat white[4]={ 1. 1. 1. 1. };
GLfloat shininess[1]={50.};
glClearColor( 0.75 0.75 1.0 0.0 );
glShadeModel(GL_SMOOTH);
glLightfv(GL_LIGHT0 GL_POSITION light_position );
glLightfv(GL_LIGHT0 GL_AMBIENT ambient_color );
glLightfv(GL_LIGHT0 GL_SPECULAR specular_color );
glLightfv(GL_LIGHT0 GL_DIFFUSE light_color );
// attributes
glEnable(GL_LIGHTING); // so lighting models are used
glEn
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 786432 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\bricks.512.512.raw
文件 8200 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Ch4Ex1.c
文件 61400 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\clouds.167
文件 616139 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\crater.167
文件 3232 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\cube.c
文件 4910 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure0.1.c
文件 5362 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure0.7.c
文件 12730 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure1.15.c
文件 12325 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure1.3.c
文件 10397 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure1.9.c
文件 11165 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure11.1.c
文件 9749 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure11.13.c
文件 9837 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure11.3.c
文件 7526 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure11.4.c
文件 2637 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure11.5.c
文件 3247 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure11.6.c
文件 15364 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure11.7left.c
文件 7334 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure11.7right.c
文件 22380 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure11.8.c
文件 3225 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure12.1.c
文件 5477 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure13.1.c
文件 5720 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure13.10.c
文件 4390 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure13.4.c
文件 5941 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure13.5.c
文件 7431 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure13.7.c
文件 14645 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure13.9.c
文件 2466 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure14.11.c
文件 3292 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure14.12.c
文件 3223 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure14.13.c
文件 6272 2007-01-02 13:31 StudentCode for Book steve Cunningham\Source code files\Figure14.6.c
............此处省略51个文件信息
- 上一篇:APPSCAN测试策略汇总
- 下一篇:方波等各种信号的傅里叶分解
相关资源
- 条码字体barcode128
- pscad近海风电模型 Fortran语言
- Xamarin forms 手势事件
- 弹塑性力学Fortran算例
- IAR For ARM 7.3最新注册机
- IAR for MSP430 v7.10.1 注册机
- IAR For ARM V5.5 注册机
- IAR for ARM 7.40 破解
- IAR For ARM 7.4 破解
- STM32蓝牙和串口程序
- 联想y470无线网卡驱动 for 32位64位
- 最新版TTF16.OCX Formula One v. 6.1.6.2 控件
- 通风网络解算程序 fortran90
- Winform倒计时器
- UNICODE GBK双向码表二进制文件
- WinForm属性编辑 propertyGrid示例 仿wind
- 常用编码(UnicodeUTF-8GBK)转换工具
- Winform调用系统的剪切,复制,粘贴文
- vc URL编解码类
- winform跨窗体传值
- 中文转化unicoder码的方法
- 关于角点检测算法HarrisForstner经典算子
- Source Insight 3.5 配置文件
- Fortran常用算法程序集-徐士良(配套程
- 大气科学常用FORTRAN程序
- 地固系惯性系坐标转换程序
- 视频处理控件TVideoGrabber.v6.7.5.For.Del
- The Impact of ETC System on Safety Performance
- Furan-BDOPV Donor-Acceptor Polymers with Plana
- Thermal stability and glass-forming ability of
评论
共有 条评论