资源简介
Ajax实现二级/三级联动下拉框---servlet版
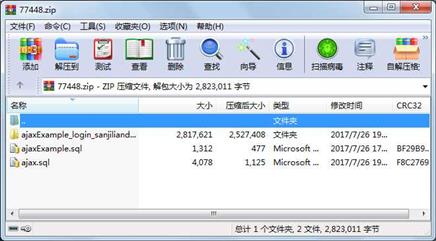
代码片段和文件信息
package yk.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import yk.entity.City;
import yk.util.JdbcUtil;
public class CityDao {
//根据外键查询数据
public List findByFKId(int id){
Connection connection = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
connection = JdbcUtil.getConnection();
String sql = “select * from city where province_id = ? “;
stmt = connection.prepareStatement(sql);
stmt.setInt(1 id);
rs = stmt.executeQuery();
List list = new ArrayList();
while (rs.next()) {
City city = new City();
city.setId(rs.getInt(“id“));
city.setName(rs.getString(“name“));
city.setProvince_id(rs.getInt(“province_id“));
list.add(city);
}
return list;
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException();
} finally{
try {
connection.close();
stmt.close();
rs.close();
} catch (Exception e2) {
throw new RuntimeException(e2);
}
}
}
public City findById(int id){
Connection connection = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
connection = JdbcUtil.getConnection();
String sql = “select * from city where id = ? “;
stmt = connection.prepareStatement(sql);
stmt.setInt(1 id);
rs = stmt.executeQuery();
City city = new City();
while (rs.next()) {
city.setId(rs.getInt(“id“));
city.setName(rs.getString(“name“));
city.setProvince_id(rs.getInt(“province_id“));
}
return city;
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException();
} finally{
try {
connection.close();
stmt.close();
rs.close();
} catch (Exception e2) {
throw new RuntimeException(e2);
}
}
}
public City findByName(String name){
Connection connection = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
connection = JdbcUtil.getConnection();
String sql = “select * from city where name = ? “;
stmt = connection.prepareStatement(sql);
stmt.setString(1 name);
rs = stmt.executeQuery();
City city = new City();
while (rs.next()) {
city.setId(rs.getInt(“id“));
city.setName(rs.getString(“name“));
city.setProvince_id(rs.getInt(“province_id“));
}
return city;
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException();
} finally{
try {
connection.close();
stmt.close();
rs.close();
} catch (Exception e2) {
throw new RuntimeException(e2);
}
}
}
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 4078 2017-07-26 19:47 ajax.sql
文件 1312 2017-07-26 17:19 ajaxExample.sql
目录 0 2017-07-26 19:27 ajaxExample_login_sanjiliandong_servletVersion\
文件 1093 2017-07-25 19:34 ajaxExample_login_sanjiliandong_servletVersion\.classpath
文件 1096 2017-07-25 19:34 ajaxExample_login_sanjiliandong_servletVersion\.project
目录 0 2017-07-26 19:27 ajaxExample_login_sanjiliandong_servletVersion\.settings\
文件 564 2017-07-25 19:34 ajaxExample_login_sanjiliandong_servletVersion\.settings\.jsdtscope
文件 364 2017-07-25 19:34 ajaxExample_login_sanjiliandong_servletVersion\.settings\org.eclipse.jdt.core.prefs
文件 597 2017-07-25 19:34 ajaxExample_login_sanjiliandong_servletVersion\.settings\org.eclipse.wst.common.component
文件 414 2017-07-25 19:34 ajaxExample_login_sanjiliandong_servletVersion\.settings\org.eclipse.wst.common.project.facet.core.xm
文件 49 2017-07-25 19:34 ajaxExample_login_sanjiliandong_servletVersion\.settings\org.eclipse.wst.jsdt.ui.superType.container
文件 6 2017-07-25 19:34 ajaxExample_login_sanjiliandong_servletVersion\.settings\org.eclipse.wst.jsdt.ui.superType.name
目录 0 2017-07-26 19:27 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\
文件 2503 2017-07-26 11:34 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\ErJiLianDongOne.jsp
文件 3058 2017-07-26 14:02 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\ErJiLianDongTwo.jsp
目录 0 2017-07-26 19:27 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\me
文件 39 2017-07-25 19:34 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\me
文件 5786 2017-07-26 18:36 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\Province_city_county.jsp
目录 0 2017-07-26 19:27 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\
目录 0 2017-07-26 19:27 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\
文件 429 2017-07-25 20:06 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\c3p0-config.xm
目录 0 2017-07-26 19:27 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\
目录 0 2017-07-26 19:27 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\dao\
文件 3753 2017-07-26 15:07 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\dao\CityDao.class
文件 3767 2017-07-26 18:52 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\dao\CountyDao.class
文件 5372 2017-07-26 11:15 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\dao\FirstSelect_twoDao.class
文件 3647 2017-07-26 15:01 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\dao\ProvinceDao.class
文件 4864 2017-07-26 14:52 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\dao\SecondSelect_twoDao.class
文件 6888 2017-07-26 11:07 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\dao\UserDao.class
目录 0 2017-07-26 19:27 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\entity\
文件 903 2017-07-26 14:53 ajaxExample_login_sanjiliandong_servletVersion\WebRoot\WEB-INF\classes\yk\entity\City.class
............此处省略72个文件信息
- 上一篇:OPENGL写的四面体纹理贴图
- 下一篇:图像分类数据
相关资源
- Servlet API中文文档
- jQuery ajax实现简单登录验证
- Ajax定时读取数据库(源代码发布)
- 仿126 网易 163 邮箱 界面
- AJAX下载
- 基于Servlet下的验证码功能的实现
- 医院项目行政管理系统源码
- 北大青鸟 易买网 源码
- 基于web的网上商城项目
- 基于ajax的web聊天室
- Json.net
- 清华大学计算机系网络课程之模式识
- Ajax访问dataSnap Rest服务器
- AjaxControlToolkit4.5
- 用ajax实现新闻发布系统
- ServletContextListener完成在线人数统计和
- 使用extjs4+servlet对extjs中grid数据进行填
- 校友信息管理系统,毕业论文
- 物联网,WEBSOCKET丰富案例
- easyui+ajax+json+servlet实现用户登录注册
- 学科竞赛系统
- AJax基础教程.pdf
- OA办公系统源代码+数据库
- e拍在线拍卖系统完整源代码及数据库
- cangkuTest.zip
- 当当网----全部代码
- 一个汽车管理系统实现增删改查功能
- 基于AJAX方式实现的STM32H7_WebServer网页
- ajax异步获取数据库数据绘制Echarts图表
- AjaxControlToolKit Ajax扩展控件
评论
共有 条评论