资源简介
http://blog.csdn.net/tangjiarao/article/details/50467804
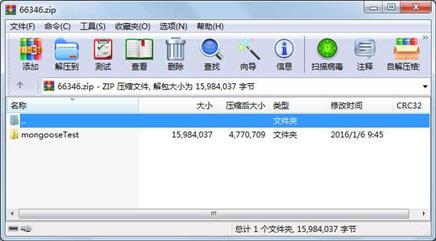
代码片段和文件信息
/**
* Copyright (c) 2006-2008 Apple Inc. All rights reserved.
*
* Licensed under the Apache License Version 2.0 (the “License“);
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing software
* distributed under the License is distributed on an “AS IS“ BASIS
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
**/
#include “base64.h“
#include
#include
#include
#include
void die2(const char *message) {
if(errno) {
perror(message);
} else {
printf(“ERROR: %s\n“ message);
}
exit(1);
}
// base64 tables
static char basis_64[] =
“ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/“;
static signed char index_64[128] =
{
-1-1-1-1 -1-1-1-1 -1-1-1-1 -1-1-1-1
-1-1-1-1 -1-1-1-1 -1-1-1-1 -1-1-1-1
-1-1-1-1 -1-1-1-1 -1-1-162 -1-1-163
52535455 56575859 6061-1-1 -1-1-1-1
-1 0 1 2 3 4 5 6 7 8 910 11121314
15161718 19202122 232425-1 -1-1-1-1
-1262728 29303132 33343536 37383940
41424344 45464748 495051-1 -1-1-1-1
};
#define CHAR64(c) (((c) < 0 || (c) > 127) ? -1 : index_64[(c)])
// base64_encode : base64 encode
//
// value : data to encode
// vlen : length of data
// (result) : new char[] - c-str of result
char *base64_encode(const unsigned char *value int vlen)
{
char *result = (char *)malloc((vlen * 4) / 3 + 5);
if(result == NULL) die2(“Memory allocation failed“);
char *out = result;
while (vlen >= 3)
{
*out++ = basis_64[value[0] >> 2];
*out++ = basis_64[((value[0] << 4) & 0x30) | (value[1] >> 4)];
*out++ = basis_64[((value[1] << 2) & 0x3C) | (value[2] >> 6)];
*out++ = basis_64[value[2] & 0x3F];
value += 3;
vlen -= 3;
}
if (vlen > 0)
{
*out++ = basis_64[value[0] >> 2];
unsigned char oval = (value[0] << 4) & 0x30;
if (vlen > 1) oval |= value[1] >> 4;
*out++ = basis_64[oval];
*out++ = (vlen < 2) ? ‘=‘ : basis_64[(value[1] << 2) & 0x3C];
*out++ = ‘=‘;
}
*out = ‘\0‘;
return result;
}
// base64_decode : base64 decode
//
// value : c-str to decode
// rlen : length of decoded result
// (result) : new unsigned char[] - decoded result
unsigned char *base64_decode(const char *value int *rlen)
{
*rlen = 0;
int c1 c2 c3 c4;
int vlen = strlen(value);
unsigned char *result =(unsigned char *)malloc((vlen * 3) / 4 + 1);
if(result == NULL) die2(“Memory allocation failed“);
u
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2016-01-06 09:45 mongooseTest\
文件 6148 2015-11-20 08:52 mongooseTest\.DS_Store
目录 0 2016-01-06 09:17 mongooseTest\.idea\
文件 5 2015-11-17 09:35 mongooseTest\.idea\.name
文件 448 2015-11-17 13:56 mongooseTest\.idea\E-WEB.iml
文件 263 2015-11-20 10:06 mongooseTest\.idea\jsLibraryMappings.xm
目录 0 2016-01-06 09:17 mongooseTest\.idea\libraries\
文件 413 2015-11-17 13:56 mongooseTest\.idea\libraries\E_WEB_node_modules.xm
文件 599 2015-11-17 09:35 mongooseTest\.idea\misc.xm
文件 262 2015-11-17 09:35 mongooseTest\.idea\modules.xm
目录 0 2016-01-06 09:17 mongooseTest\.idea\runConfigurations\
文件 394 2015-11-20 10:26 mongooseTest\.idea\runConfigurations\bin_www.xm
文件 164 2015-11-17 09:35 mongooseTest\.idea\vcs.xm
文件 143 2015-11-17 13:47 mongooseTest\.idea\watcherTasks.xm
文件 37964 2016-01-06 13:51 mongooseTest\.idea\workspace.xm
文件 854 2016-01-06 09:45 mongooseTest\app.js
目录 0 2016-01-06 09:43 mongooseTest\bin\
文件 1592 2016-01-06 09:43 mongooseTest\bin\www
目录 0 2016-01-06 09:17 mongooseTest\node_modules\
目录 0 2016-01-06 09:17 mongooseTest\node_modules\.bin\
文件 19 2015-11-17 09:35 mongooseTest\node_modules\.bin\jade
目录 0 2016-01-06 09:17 mongooseTest\node_modules\body-parser\
文件 9140 2015-08-01 02:59 mongooseTest\node_modules\body-parser\HISTORY.md
文件 2649 2015-06-15 07:07 mongooseTest\node_modules\body-parser\index.js
目录 0 2016-01-06 09:17 mongooseTest\node_modules\body-parser\lib\
文件 3512 2015-06-15 08:13 mongooseTest\node_modules\body-parser\lib\read.js
目录 0 2016-01-06 09:17 mongooseTest\node_modules\body-parser\lib\types\
文件 3608 2015-06-15 07:17 mongooseTest\node_modules\body-parser\lib\types\json.js
文件 1847 2015-06-15 06:42 mongooseTest\node_modules\body-parser\lib\types\raw.js
文件 2238 2015-06-15 07:16 mongooseTest\node_modules\body-parser\lib\types\text.js
文件 5414 2015-07-06 11:13 mongooseTest\node_modules\body-parser\lib\types\urlencoded.js
............此处省略2405个文件信息
评论
共有 条评论