资源简介
国密算法SM2,SM3,SM4,有源码,VC工程,基于OPENSSL.
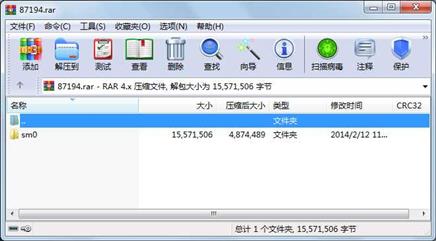
代码片段和文件信息
// \file:sm2.c
//SM2 Algorithm
//depending:opnessl library
//SM2 Standards: http://www.oscca.gov.cn/News/201012/News_1197.htm
#include
#include
#include
#include
#include
#include
#include
#include “kdf.h“
#define NID_X9_62_prime_field 406
static void BNPrintf(BIGNUM* bn)
{
char *p=NULL;
p=BN_bn2hex(bn);
printf(“%s“p);
OPENSSL_free(p);
}
static int sm2_sign_setup(EC_KEY *eckey BN_CTX *ctx_in BIGNUM **kp BIGNUM **rp)
{
BN_CTX *ctx = NULL;
BIGNUM *k = NULL *r = NULL *order = NULL *X = NULL;
EC_POINT *tmp_point=NULL;
const EC_GROUP *group;
int ret = 0;
if (eckey == NULL || (group = EC_KEY_get0_group(eckey)) == NULL)
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUP ERR_R_PASSED_NULL_PARAMETER);
return 0;
}
if (ctx_in == NULL)
{
if ((ctx = BN_CTX_new()) == NULL)
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUPERR_R_MALLOC_FAILURE);
return 0;
}
}
else
ctx = ctx_in;
k = BN_new(); /* this value is later returned in *kp */
r = BN_new(); /* this value is later returned in *rp */
order = BN_new();
X = BN_new();
if (!k || !r || !order || !X)
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUP ERR_R_MALLOC_FAILURE);
goto err;
}
if ((tmp_point = EC_POINT_new(group)) == NULL)
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUP ERR_R_EC_LIB);
goto err;
}
if (!EC_GROUP_get_order(group order ctx))
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUP ERR_R_EC_LIB);
goto err;
}
do
{
/* get random k */
do
if (!BN_rand_range(k order))
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUP ECDSA_R_RANDOM_NUMBER_GENERATION_FAILED);
goto err;
}
while (BN_is_zero(k));
/* compute r the x-coordinate of generator * k */
if (!EC_POINT_mul(group tmp_point k NULL NULL ctx))
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUP ERR_R_EC_LIB);
goto err;
}
if (EC_METHOD_get_field_type(EC_GROUP_method_of(group)) == NID_X9_62_prime_field)
{
if (!EC_POINT_get_affine_coordinates_GFp(group
tmp_point X NULL ctx))
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUPERR_R_EC_LIB);
goto err;
}
}
else /* NID_X9_62_characteristic_two_field */
{
if (!EC_POINT_get_affine_coordinates_GF2m(group
tmp_point X NULL ctx))
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUPERR_R_EC_LIB);
goto err;
}
}
if (!BN_nnmod(r X order ctx))
{
ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUP ERR_R_BN_LIB);
goto err;
}
}
while (BN_is_zero(r));
/* compute the inverse of k */
// if (!BN_mod_inverse(k k order ctx))
// {
// ECDSAerr(ECDSA_F_ECDSA_SIGN_SETUP ERR_R_BN_LIB);
// goto err;
// }
/* clear old values if necessary */
if (*rp != NULL)
BN_clear_free(*rp);
if (*kp != NULL)
BN_clear_free(*kp);
/* save the pre-computed values */
*rp = r;
*kp = k;
ret = 1;
err:
if (!ret)
{
if
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 1100800 2013-02-06 05:35 sm0\libeay32.dll
文件 40 2013-03-08 22:46 sm0\read me.txt
文件 155683 2013-03-07 16:55 sm0\SM.exe
文件 1069056 2014-01-19 12:43 sm0\SM2\Debug\libeay32.dll
文件 674176 2014-01-19 12:43 sm0\SM2\Debug\libeay32.lib
文件 196668 2014-02-12 11:39 sm0\SM2\Debug\sm2.exe
文件 270016 2014-02-12 11:39 sm0\SM2\Debug\sm2.ilk
文件 33802 2014-02-12 11:39 sm0\SM2\Debug\sm2.obj
文件 4407800 2014-02-12 11:39 sm0\SM2\Debug\sm2.pch
文件 394240 2014-02-12 11:39 sm0\SM2\Debug\sm2.pdb
文件 1272255 2014-02-12 11:39 sm0\SM2\Debug\sm2.sbr
文件 41988 2014-02-12 11:39 sm0\SM2\Debug\sm2test.obj
文件 1163120 2014-02-12 11:39 sm0\SM2\Debug\sm2test.sbr
文件 50176 2014-02-12 11:42 sm0\SM2\Debug\vc60.idb
文件 69632 2014-02-12 11:39 sm0\SM2\Debug\vc60.pdb
文件 1368 2011-11-10 17:49 sm0\SM2\kdf.h
文件 19111 2013-03-08 22:48 sm0\SM2\sm2.c
文件 3611 2014-02-11 21:34 sm0\SM2\sm2.dsp
文件 529 2011-11-09 18:45 sm0\SM2\sm2.dsw
文件 807 2013-03-08 22:48 sm0\SM2\sm2.h
文件 66560 2014-02-12 11:42 sm0\SM2\sm2.ncb
文件 931328 2014-02-12 11:42 sm0\SM2\sm2.opt
文件 240 2014-02-12 11:42 sm0\SM2\sm2.plg
文件 13569 2013-03-08 22:48 sm0\SM2\sm2test.c
文件 945731 2013-01-22 14:51 sm0\SM2_SM3_SM4_技术规范\国密算法SM2-1.pdf
文件 22552 2013-01-22 14:51 sm0\SM2_SM3_SM4_技术规范\国密算法SM2-2.pdf
文件 103677 2013-01-22 14:51 sm0\SM2_SM3_SM4_技术规范\国密算法SM3.pdf
文件 205356 2011-10-12 22:31 sm0\SM2_SM3_SM4_技术规范\国密算法SMS4.pdf
文件 16365 2013-02-26 17:50 sm0\SM3\Debug\sm3.obj
文件 168003 2013-02-26 17:50 sm0\SM3\Debug\sm3test.exe
............此处省略45个文件信息
相关资源
- Openssl给文件传输加密
- openssl 简介(中文)
- SM2国密算法实现基于mircal的实现
- openssl 手册中文版
- centos 7 openssh7.9p 201810月最新版,基于
- win64OpenSSL_Light
- 利用openssl和curl库获取https服务端证书
- linux c 使用openssl实现SHA1WithRSA实现,签
- openssl win7 64位
- indy10以上openssl支持所需要的文件。
- openssl098e-0.9.8e-17.el6.centos.src.rpm
- openssl-0.9.8k_WIN32.rar
- vs2010 win7下编译的openssl-1.0.2n静态库
- OpenSSL-1.0.2m静态库
- 基于OpenSSL库的ECDSA签名与验证和文档
- openssl-0.9.8k_WIN32(RSA密钥生成工具)
- OpenSSL-win64库使用依赖文件
- openssl.exe 0.9.8版本
- windows64的curl库支持openssl)
- openssl(arm64armv7sarmv7x86_64i386)
- 国密算法SM2 SM3 SM4相关算法及推荐参数
- openssl-1.0.2k
- openssl-1.0.2n.tar.gz
- apache-httpd-2.2.24-x64-openssl安装版 msi安装
- vs2013编译好的OpenSSL_1_0_2静态库和动态
- httpd-2.2.22-win32-x86-openssl-0.9.8t.msi
- openssl绿色版
- CentOS-6.6-x86_64 nginx 依赖 pcre-devel zli
- OpenSSL_Light_Win64.msi
- 国密算法SM3验证与SM4文件加密工具C
评论
共有 条评论