-
大小: 5.25MB文件类型: .zip金币: 2下载: 0 次发布日期: 2023-09-22
- 语言: 其他
- 标签: SerialPort 串口 自动发送
资源简介
自己用SerialPort类写的串口程序,适合初学者看看,带有自动发送功能,打开串口,关闭串口以及自动连接串口功能
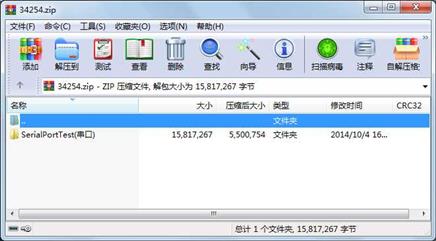
代码片段和文件信息
#include “stdafx.h“
#include “SerialPort.h“
#include
CSerialPort::CSerialPort()
{
m_hComm = NULL;
m_ov.Offset = 0;
m_ov.OffsetHigh = 0;
// create events
m_ov.hEvent = NULL;
m_hWriteEvent = NULL;
m_hShutdownEvent = NULL;
m_szWriteBuffer = NULL;
m_bThreadAlive = FALSE;
m_nWriteSize = 1;
}
//
// Delete dynamic memory
//
CSerialPort::~CSerialPort()
{
do
{
SetEvent(m_hShutdownEvent);
} while (m_bThreadAlive);
if (m_hComm != NULL)
{
CloseHandle(m_hComm);
m_hComm = NULL;
}
// Close Handles
if(m_hShutdownEvent!=NULL)
CloseHandle( m_hShutdownEvent);
if(m_ov.hEvent!=NULL)
CloseHandle( m_ov.hEvent );
if(m_hWriteEvent!=NULL)
CloseHandle( m_hWriteEvent );
TRACE(“Thread ended\n“);
delete [] m_szWriteBuffer;
}
BOOL CSerialPort::InitPort(CWnd* pPortOwner // the owner (CWnd) of the port (receives message)
UINT portnr // portnumber (1..4)
UINT baud // baudrate
char parity // parity
UINT databits // databits
UINT stopbits // stopbits
DWORD dwCommEvents // EV_RXCHAR EV_CTS etc
UINT writebuffersize// size to the writebuffer
DWORD ReadIntervalTimeout
DWORD ReadTotalTimeoutMultiplier
DWORD ReadTotalTimeoutConstant
DWORD WriteTotalTimeoutMultiplier
DWORD WriteTotalTimeoutConstant )
{
// assert(portnr > 0 && portnr < 5);
// assert(pPortOwner != NULL);
// if the thread is alive: Kill
if (m_bThreadAlive)
{
do
{
SetEvent(m_hShutdownEvent);
} while (m_bThreadAlive);
TRACE(“Thread ended\n“);
}
// create events
if (m_ov.hEvent != NULL)
ResetEvent(m_ov.hEvent);
else
m_ov.hEvent = CreateEvent(NULL TRUE FALSE NULL);
if (m_hWriteEvent != NULL)
ResetEvent(m_hWriteEvent);
else
m_hWriteEvent = CreateEvent(NULL TRUE FALSE NULL);
if (m_hShutdownEvent != NULL)
ResetEvent(m_hShutdownEvent);
else
m_hShutdownEvent = CreateEvent(NULL TRUE FALSE NULL);
// initialize the event objects
m_hEventArray[0] = m_hShutdownEvent; // highest priority
m_hEventArray[1] = m_ov.hEvent;
m_hEventArray[2] = m_hWriteEvent;
// initialize critical section
InitializeCriticalSection(&m_csCommunicationSync);
// set buffersize for writing and save the owner
m_pOwner = pPortOwner;
if (m_szWriteBuffer != NULL)
delete [] m_szWriteBuffer;
m_szWriteBuffer = new char[writebuffersize];
m_nPortNr = portnr;
m_nWriteBufferSize = writebuffersize;
m_dwCommEvents = dwCommEvents;
BOOL bResult = FALSE;
char *szPort = new char[50];
char *szBaud = new char[50];
// now it critical!
EnterCriticalSection(&m_csCommunicationSync);
// if the port is already opened: close it
if (m_hComm != NULL)
{
CloseHandle(m_hComm);
m_hComm = NULL;
}
// prepare port strings
sprintf(szPort _T(“COM%d“) portnr);
// stop is index 0
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2014-10-04 16:59 SerialPortTest(串口)\
目录 0 2014-10-04 16:57 SerialPortTest(串口)\Debug\
文件 31473 2014-09-15 20:14 SerialPortTest(串口)\Debug\SerialPort.obj
文件 0 2014-09-15 20:14 SerialPortTest(串口)\Debug\SerialPort.sbr
文件 5391360 2014-09-22 12:49 SerialPortTest(串口)\Debug\SerialPortTest.bsc
文件 118902 2014-09-22 12:49 SerialPortTest(串口)\Debug\SerialPortTest.exe
文件 357096 2014-09-22 12:49 SerialPortTest(串口)\Debug\SerialPortTest.ilk
文件 15320 2014-09-15 21:20 SerialPortTest(串口)\Debug\SerialPortTest.obj
文件 6932736 2014-09-15 20:14 SerialPortTest(串口)\Debug\SerialPortTest.pch
文件 451584 2014-09-22 12:49 SerialPortTest(串口)\Debug\SerialPortTest.pdb
文件 3028 2014-09-17 21:28 SerialPortTest(串口)\Debug\SerialPortTest.res
文件 0 2014-09-15 21:20 SerialPortTest(串口)\Debug\SerialPortTest.sbr
文件 38783 2014-09-22 12:49 SerialPortTest(串口)\Debug\SerialPortTestDlg.obj
文件 0 2014-09-22 12:49 SerialPortTest(串口)\Debug\SerialPortTestDlg.sbr
文件 105972 2014-09-15 20:14 SerialPortTest(串口)\Debug\StdAfx.obj
文件 1369994 2014-09-15 20:14 SerialPortTest(串口)\Debug\StdAfx.sbr
文件 238592 2014-10-04 16:59 SerialPortTest(串口)\Debug\vc60.idb
文件 372736 2014-09-22 12:49 SerialPortTest(串口)\Debug\vc60.pdb
文件 3723 2014-08-31 19:28 SerialPortTest(串口)\ReadMe.txt
目录 0 2014-10-04 16:57 SerialPortTest(串口)\res\
文件 1106 2014-09-17 21:26 SerialPortTest(串口)\resource.h
文件 1078 2014-08-31 19:28 SerialPortTest(串口)\res\SerialPortTest.ico
文件 406 2014-08-31 19:28 SerialPortTest(串口)\res\SerialPortTest.rc2
文件 17508 2014-09-13 10:00 SerialPortTest(串口)\SerialPort.cpp
文件 3402 2014-09-13 09:25 SerialPortTest(串口)\SerialPort.h
文件 21672 2014-10-04 16:58 SerialPortTest(串口)\SerialPortTest.aps
文件 1679 2014-10-04 16:59 SerialPortTest(串口)\SerialPortTest.clw
文件 2175 2014-08-31 19:28 SerialPortTest(串口)\SerialPortTest.cpp
文件 4436 2014-09-15 20:50 SerialPortTest(串口)\SerialPortTest.dsp
文件 551 2014-08-31 19:28 SerialPortTest(串口)\SerialPortTest.dsw
文件 1414 2014-08-31 20:08 SerialPortTest(串口)\SerialPortTest.h
............此处省略8个文件信息
相关资源
- STM32蓝牙和串口程序
- LCD显示温度+串口接收温度.rar
- WPF USB 网络 串口 通信软件
- DELPHI与西门子200PLC的串口通信实例
- Verilog FPGA UART串口控制器
- USB转串口驱动,FT232R驱动程序,最新
- PC -- 单片机的串口数据传输系统设计
- STM32F103 串口程序(完整版)
- stm32 ds18b20 温度传感器 测试通过
- PC 串口调试软件
- 51单片机读取温度数据存储到SD卡中并
- 单片机与PC机串口通讯仿真
- 串口调试助手V5.0
- 双串口调试助手 V4.0 _ 可同时调试两个
- 读取串口数据并画实时曲线的VC 程序
- 网络(UDP)转串口程序
- 串口绘制曲线 将收到的数据进行曲线
- 基于ARM蓝牙传输源程序
- JS操作本地网页串口源码
- AT89S ISP下载编程软件(串口)
- 串口操作类(justinio)
- 《Visual Basic 串口通信与测控应用技术
- 耀华XK3190-A9地磅串口代码
- ch452串口键盘驱动程序
- 托利多电子秤ind245协议读取串口数据
- 通过串口s19文件源代码
- 友善串口调试助手V3.7.3,带注册码
- win7 64bit 串口调试工具
- SSCOM32
- Mac电脑串口工具(2018)
评论
共有 条评论