资源简介
FreeType 结合opencv –> 在图像上显示中文
代码编写参考:
http://blog.csdn.net/huixingshao/article/details/43563853#comments
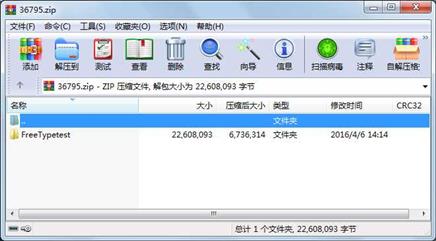
代码片段和文件信息
#include
#include
#include
#include
#include “CvxText.h“
//====================================================================
//====================================================================
// 打开字库
CvxText::CvxText(const char *freeType)
{
assert(freeType != NULL);
// 打开字库文件 创建一个字体
if(FT_Init_FreeType(&m_library)) throw;
if(FT_New_Face(m_library freeType 0 &m_face)) throw;
// 设置字体输出参数
restoreFont();
// 设置C语言的字符集环境
setlocale(LC_ALL ““);
}
// 释放FreeType资源
CvxText::~CvxText()
{
FT_Done_Face (m_face);
FT_Done_FreeType(m_library);
}
// 设置字体参数:
//
// font - 字体类型 目前不支持
// size - 字体大小/空白比例/间隔比例/旋转角度
// underline - 下画线
// diaphaneity - 透明度
void CvxText::getFont(int *type CvScalar *size bool *underline float *diaphaneity)
{
if(type) *type = m_fontType;
if(size) *size = m_fontSize;
if(underline) *underline = m_fontUnderline;
if(diaphaneity) *diaphaneity = m_fontDiaphaneity;
}
void CvxText::setFont(int *type CvScalar *size bool *underline float *diaphaneity)
{
// 参数合法性检查
if(type)
{
if(type >= 0) m_fontType = *type;
}
if(size)
{
m_fontSize.val[0] = fabs(size->val[0]);
m_fontSize.val[1] = fabs(size->val[1]);
m_fontSize.val[2] = fabs(size->val[2]);
m_fontSize.val[3] = fabs(size->val[3]);
}
if(underline)
{
m_fontUnderline = *underline;
}
if(diaphaneity)
{
m_fontDiaphaneity = *diaphaneity;
}
FT_Set_Pixel_Sizes(m_face (int)m_fontSize.val[0] 0);
}
// 恢复原始的字体设置
void CvxText::restoreFont()
{
m_fontType = 0; // 字体类型(不支持)
m_fontSize.val[0] = 20; // 字体大小
m_fontSize.val[1] = 0.5; // 空白字符大小比例
m_fontSize.val[2] = 0.1; // 间隔大小比例
m_fontSize.val[3] = 0; // 旋转角度(不支持)
m_fontUnderline = false; // 下画线(不支持)
m_fontDiaphaneity = 1.0; // 色彩比例(可产生透明效果)
// 设置字符大小
FT_Set_Pixel_Sizes(m_face (int)m_fontSize.val[0] 0);
}
// 输出函数(颜色默认为黑色)
int CvxText::putText(IplImage *img const char *text CvPoint pos)
{
return putText(img text pos CV_RGB(255255255));
}
int CvxText::putText(IplImage *img const wchar_t *text CvPoint pos)
{
return putText(img text pos CV_RGB(255255255));
}
//
int CvxText::putText(IplImage *img const char *text CvPoint pos CvScalar color)
{
if(img == NULL) return -1;
if(text == NULL) return -1;
//
int i;
for(i = 0; text[i] != ‘\0‘; ++i)
{
wchar_t wc = text[i];
// 解析双字节符号
if(!isascii(wc)) mbtowc(&wc &text[i++] 2);
// 输出当前的字符
putWChar(img wc pos color);
}
return i;
}
int CvxText::putText(IplImage *img const wchar_t *text CvPoint pos CvScalar color)
{
if(img == NULL) return -1;
if(text == NULL) return -1;
//
int i;
for(i = 0; text[i] != ‘\0‘; ++i)
{
// 输出当前的字符
putWChar(img text[i] pos color);
}
return i;
}
// 输出当前字符 更新m_pos位置
void CvxText::putWChar(IplImage *img wchar_t wc CvPoint &pos CvScalar color)
{
// 根据unicode生成字体的二值位图
FT_UInt glyph_index = FT_Get_Char_Index(m_face wc);
FT_Load_Glyph(m_face
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2016-04-06 14:14 FreeTypetest\
目录 0 2016-04-06 14:13 FreeTypetest\FTtest\
文件 885 2016-04-06 13:43 FreeTypetest\FTtest.sln
文件 12800 2016-04-06 14:13 FreeTypetest\FTtest.suo
文件 4234 2016-04-06 13:48 FreeTypetest\FTtest\CvxText.cpp
文件 5681 2016-04-06 13:57 FreeTypetest\FTtest\CvxText.h
目录 0 2016-04-06 13:51 FreeTypetest\FTtest\ft251_slib\
目录 0 2016-04-06 13:51 FreeTypetest\FTtest\ft251_slib\include\
目录 0 2016-04-06 13:51 FreeTypetest\FTtest\ft251_slib\include\config\
文件 24206 2013-11-12 19:24 FreeTypetest\FTtest\ft251_slib\include\config\ftconfig.h
文件 26419 2013-11-12 19:27 FreeTypetest\FTtest\ft251_slib\include\config\ftheader.h
文件 1431 2009-03-14 21:45 FreeTypetest\FTtest\ft251_slib\include\config\ftmodule.h
文件 53774 2013-11-12 19:26 FreeTypetest\FTtest\ft251_slib\include\config\ftoption.h
文件 7487 2012-09-29 15:42 FreeTypetest\FTtest\ft251_slib\include\config\ftstdlib.h
文件 256336 2013-11-21 22:42 FreeTypetest\FTtest\ft251_slib\include\freetype.h
文件 2425 2013-11-13 16:46 FreeTypetest\FTtest\ft251_slib\include\ft2build.h
文件 10758 2013-09-03 17:02 FreeTypetest\FTtest\ft251_slib\include\ftadvanc.h
文件 13258 2013-09-03 17:03 FreeTypetest\FTtest\ft251_slib\include\ftautoh.h
文件 5347 2013-09-03 17:03 FreeTypetest\FTtest\ft251_slib\include\ftbbox.h
文件 6958 2009-03-14 21:45 FreeTypetest\FTtest\ft251_slib\include\ftbdf.h
文件 14225 2013-05-29 15:24 FreeTypetest\FTtest\ft251_slib\include\ftbitmap.h
文件 4409 2011-01-01 00:44 FreeTypetest\FTtest\ft251_slib\include\ftbzip2.h
文件 58586 2013-09-03 17:05 FreeTypetest\FTtest\ft251_slib\include\ftcache.h
文件 10140 2013-10-24 03:31 FreeTypetest\FTtest\ft251_slib\include\ftcffdrv.h
文件 8556 2013-09-03 17:08 FreeTypetest\FTtest\ft251_slib\include\ftchapters.h
文件 5745 2009-07-03 21:28 FreeTypetest\FTtest\ft251_slib\include\ftcid.h
文件 12648 2013-05-29 05:00 FreeTypetest\FTtest\ft251_slib\include\fterrdef.h
文件 9490 2013-05-29 05:00 FreeTypetest\FTtest\ft251_slib\include\fterrors.h
文件 4630 2012-01-17 18:32 FreeTypetest\FTtest\ft251_slib\include\ftgasp.h
文件 40439 2013-09-03 17:10 FreeTypetest\FTtest\ft251_slib\include\ftglyph.h
文件 12954 2013-09-03 17:10 FreeTypetest\FTtest\ft251_slib\include\ftgxval.h
............此处省略90个文件信息
相关资源
- MiniGUI库文件之带TTF- libminigui-1.6.10-t
- OpenGL+FreeType渲染宋体中文
- freetype 矢量字体
- 国内首篇利用freetype的跨平台truetype字
- freetype-1.3.1.tar.gz
- freetype2.6.2
- QT下自定义控件的拖动,标尺,控件的
- freetype-2.9.1
- freetype2+SDL+SDL_ttf源码包及字符串转b
- QT实现基于freetype的TTF字体拆解
- Qt使用FreeType字轮廓线动画
- linux下用freetype2显示汉字
- freetype的Lib、头文件及调用样例
- Freetype 中文教程
- OpenGL、FreeType 中文纹理绘制
- freetype-2.4.8.tar.bz2
- freetype-2.8
- FreeType库
- 使用Qt(mingw)编译FreeType为 .a 静态库
- freetype-2.10.0.tar.gz最新版高速
- freetype_SDL_Dl_ttf_debug.tar.gz
- stm32矢量字体freetype.rar
评论
共有 条评论