资源简介
smppapi-0.3.9.rar
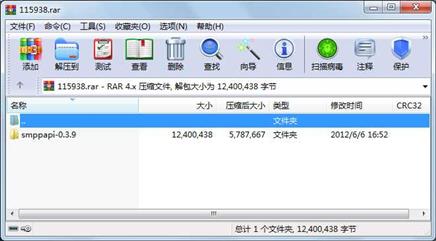
代码片段和文件信息
/*
* Java SMPP API
* Copyright (C) 1998 - 2002 by Oran Kelly
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not write to the Free Software
* Foundation Inc. 59 Temple Place Suite 330 Boston MA 02111-1307 USA
*
* A copy of the LGPL can be viewed at http://www.gnu.org/copyleft/lesser.html
* Java SMPP API author: orank@users.sf.net
* Java SMPP API Homepage: http://smppapi.sourceforge.net/
*
* $Id: stress_recv.c 242 2005-11-14 20:37:19Z orank $
*
*
* stress_recv.c: A simple program to dump a hell of a load of deliver_sm
* messages to a receiver program. Use is pretty straightforward: simply run
* this program from the command line. It sets up a server socket and listens
* for a connection...run your receiver to bind to this program‘s IP/port. If
* the first packet received isn‘t a bind_receiver the program just exits. Once
* the receiver connection is established stress_recv starts sending deliver_sm
* packets to the receiver...the destination address and message text are
* randomly generated the rest remains static (except for incrementing sequence
* numbers). The server will count the number of deliver_sm_resp messages it
* gets back and provides a simple report upon completion. Once all the
* deliver_sm‘s have been sent the server sends an unbind request to the client
* to close the connection.
* Author: Oran Kelly (orank@users.sf.net)
*/
#include
#include
#include
#include
#include
#include
#include
#include
#include “stress_recv.h“
pthread_t sender_thread;
pthread_t recv_thread;
pthread_mutex_t write_lock = PTHREAD_MUTEX_INITIALIZER;
int unbinding = 0;
void *deliver_msgs(void *arg);
void *dump_incoming(void *arg);
void make_random_msg(unsigned char *len char *buf);
void make_random_dest(char *dest);
void *dump_incoming(void *arg);
int read_smpp_packet(int sock char *buf int *buf_size);
void make_bind_receiver(char *buf bind_receiver *r);
void make_deliver_sm(char *buf deliver_sm *d);
void usage(int argc char *argv[]);
/*
* Stucture for arguments to send to the deliver_msgs function.
*/
struct thread_args1
{
int sock;
uint32_t msg_count;
};
int main(int argc char *argv[])
{
int server_sock = -1 sock = -1;
uint32_t msg_count = DEFAULT_MSG_COUNT;
int pak_size = 512 i;
int listen_port = DEFAULT_LISTEN_PORT;
char
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 16384 2009-06-17 03:28 smppapi-0.3.9\.build.xm
文件 387 2009-06-17 03:26 smppapi-0.3.9\.checkst
文件 664 2009-06-17 03:26 smppapi-0.3.9\.classpath
文件 24576 2009-06-17 03:55 smppapi-0.3.9\.pom.xm
文件 366 2009-06-17 03:26 smppapi-0.3.9\.project
文件 620 2009-06-17 03:26 smppapi-0.3.9\.settings\org.eclipse.jdt.core.prefs
文件 11558 2009-06-17 03:26 smppapi-0.3.9\apache-licence.txt
文件 2719 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\Address.class
文件 531 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\AlreadyBoundException.class
文件 1025 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\BadCommandIDException.class
文件 24923 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\Connection.class
文件 1452 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\ErrorAddress.class
文件 298 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\ConnectionObserver.class
文件 466 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\EventDispatcher.class
文件 1762 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\FIFOQueue.class
文件 1519 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\NotificationDetails.class
文件 91 2009-06-17 03:26 smppapi-0.3.9\build\classes\ie\omk\smpp\event\package.html
文件 821 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\QueueFullException.class
文件 938 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\ReceiverExceptionEvent.class
文件 1619 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\ReceiverExitEvent.class
文件 433 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\ReceiverStartEvent.class
文件 4689 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\SimpleEventDispatcher.class
文件 756 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\SMPPEvent.class
文件 6576 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\SMPPEventAdapter.class
文件 7260 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\event\ThreadedEventDispatcher.class
文件 536 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\InvalidOperationException.class
文件 1379 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\message\AlertNotification.class
文件 3927 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\message\Bind.class
文件 433 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\message\BindReceiver.class
文件 722 2012-06-12 09:19 smppapi-0.3.9\build\classes\ie\omk\smpp\message\BindReceiverResp.class
............此处省略822个文件信息
相关资源
- PID_AutoTune_v0.rar
- vspd7.2.308.zip
- 价值2k的H漫画小说系统
- Pythonamp;课堂amp;笔记(高淇amp;400;集第
- ddos压力测试工具99657
- UML建模大全
- 开源1A锂电池充电板TP4056原理图+PCB
- m1卡 ic卡可选择扇区初始化加密软件
- TSCC.exe
- FTP课程设计(服务端+客户端)
- 计算机图形学 边填充算法实现代码
- 电力系统潮流计算程序集合
- oracle数据迁移项目实施方案
- Web Api 通过文件流 文件到本地
- Visio图标-最新最全的网络通信图标库
- Spire API文档
- OpenGL参考手册
- Python中Numpy库最新教程
- SPD博士V5.3.exe
- 直流无刷电机方波驱动 stm32 例程代码
- layui后台管理模板
- 仿知乎界面小程序源代码
- 云平台-阿里云详细介绍
- photoshop经典1000例
- scratch垃圾分类源码(最终版本).sb
- IAR ARM 7.8破解
- TI CCS V5.4 安装步骤及破解文件
- 松下plc FP-XH的驱动
- 局域网硬件信息收集工具
- 加快Windows XP操作系统开机速度
评论
共有 条评论