资源简介
FFTW ARM cortex-A 平台测试程序
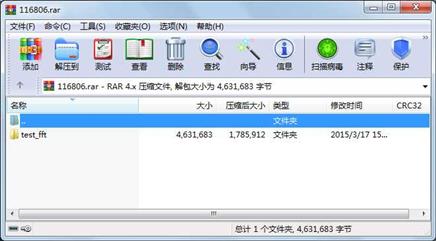
代码片段和文件信息
/*
* test.c
*
* Created on: 2015-3-6
* Author: Sean Zhou
*/
#include
#include
#include
#include
#if 1
#include “fftw3.h“
typedef unsigned long long int u64;
enum {
real_2_complex
complex_2_real
complex_2_complex
};
enum {
FORWARD
BACKWARD
};
typedef struct FFTPlan
{
fftwf_plan planForward;
fftwf_plan planBackward;
fftwf_complex *pIn; // malloc address
fftwf_complex *pOut; // malloc address
int width;
int height;
}FFTPlan;
FFTPlan* fftPlanInit(int width int height)
{
FFTPlan *pPlan = (FFTPlan*)malloc(sizeof(FFTPlan));
pPlan->height = height;
pPlan->width = width;
pPlan->pIn = (fftwf_complex*)fftwf_malloc(width*height*sizeof(fftwf_complex));
pPlan->pOut = (fftwf_complex*)fftwf_malloc(width*height*sizeof(fftwf_complex));
pPlan->planForward = fftwf_plan_dft_2d(height width pPlan->pIn pPlan->pOut FFTW_FORWARD FFTW_ESTIMATE /*FFTW_MEASURE*/);
pPlan->planBackward = fftwf_plan_dft_2d(height width pPlan->pIn pPlan->pOut FFTW_BACKWARD FFTW_ESTIMATE /*FFTW_MEASURE*/);
return pPlan;
}
void fftPlanDestroy(FFTPlan* pPlan)
{
if(pPlan == NULL)
return;
if(pPlan->pIn != NULL)
fftwf_free(pPlan->pIn);
if(pPlan->pOut != NULL)
fftwf_free(pPlan->pOut);
fftwf_destroy_plan(pPlan->planForward);
fftwf_destroy_plan(pPlan->planBackward);
free(pPlan);
}
static void displayOutput(float* pData int width int height)
{
int i j;
printf(“\n displayOutput: \n\n“);
for(i = 0; i < height; i++)
{
for(j = 0; j< width; j++)
{
printf(“%+5f“ *pData++);
printf(“%+5fi “ *pData++);
}
printf(“\n“);
}
}
void fft2(FFTPlan* pPlan float* pIn float* pOut int modeint direct)
{
int i;
int size = pPlan->width * pPlan->height;
float *pTemp;
fftwf_complex *pIn_used = pPlan->pIn;
fftwf_complex *pOut_used = pPlan->pOut;
// input
switch(mode)
{
case real_2_complex:
pTemp = (float*)pIn_used;
for(i = 0; i < size; i++)
{
*pTemp++ = *pIn++;
*pTemp++ = 0;
}
break;
case complex_2_real:
case complex_2_complex:
pTemp = (float*)pIn_used;
memcpy(pTemp pIn size*sizeof(float)*2);
break;
}
// displayInput((float*)pIn_used pPlan->width pPlan->height);
if(direct == FORWARD)
fftwf_execute(pPlan->planForward);
else if(direct == BACKWARD)
fftwf_execute(pPlan->planBackward);
else
{
printf(“Error: invalid parameter \n“);
return;
}
// displayOutput((float*)pOut_used pPlan->width pPlan->height);
// output
switch(mode)
{
case real_2_complex:
case complex_2_complex:
pTemp = (float*)pOut_used;
memcpy(pOut pTemp size*sizeof(float)*2);
break;
case complex_2_real:
pTemp = (float*)pOut_used;
for(i = 0; i < size; i++)
{
*pOut++ = *pTemp;
pTemp += 2;
}
break;
}
}
u64 getCurrentTime()
{
u64 currentTime;
struct timeval tv;
ge
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
....... 25413 2015-03-17 18:44 test_fft\fftw3\include\fftw3q.f03
....... 17900 2015-03-17 18:44 test_fft\fftw3\include\fftw3.h
....... 26820 2015-03-17 18:44 test_fft\fftw3\include\fftw3l.f03
....... 2447 2015-03-17 18:44 test_fft\fftw3\include\fftw3.f
....... 54273 2015-03-17 18:44 test_fft\fftw3\include\fftw3.f03
....... 652 2015-03-17 18:44 test_fft\fftw3\share\info\dir
....... 6170 2015-03-17 18:44 test_fft\fftw3\share\info\fftw3.info
....... 77145 2015-03-17 18:44 test_fft\fftw3\share\info\fftw3.info-2
....... 298053 2015-03-17 18:44 test_fft\fftw3\share\info\fftw3.info-1
....... 3597 2015-03-17 18:44 test_fft\fftw3\share\man\man1\fftw-wisdom-to-conf.1
....... 6901 2015-03-17 18:44 test_fft\fftw3\share\man\man1\fftwf-wisdom.1
文件 1673724 2015-03-17 18:44 test_fft\fftw3\bin\fftwf-wisdom
文件 2279 2015-03-17 18:44 test_fft\fftw3\bin\fftw-wisdom-to-conf
....... 263 2015-03-17 18:44 test_fft\fftw3\lib\pkgconfig\fftw3f.pc
文件 917 2015-03-17 18:44 test_fft\fftw3\lib\libfftw3f.la
....... 2412920 2015-03-17 18:44 test_fft\fftw3\lib\libfftw3f.a
..A..H. 12270 2015-03-06 15:22 test_fft\.cproject
文件 4993 2015-03-18 10:10 test_fft\test.c
..A..H. 838 2015-03-06 15:22 test_fft\.project
文件 1268 2015-03-17 14:42 test_fft\CamLinux.mk
文件 551 2015-03-17 15:59 test_fft\Debug\sources.mk
文件 239 2015-03-17 15:58 test_fft\Debug\ob
文件 1381 2015-03-17 15:59 test_fft\Debug\makefile
文件 669 2015-03-17 15:59 test_fft\Debug\subdir.mk
目录 0 2015-03-17 14:04 test_fft\fftw3\share\man\man1
目录 0 2015-03-17 14:04 test_fft\fftw3\share\info
目录 0 2015-03-17 14:04 test_fft\fftw3\share\man
目录 0 2015-03-17 14:04 test_fft\fftw3\lib\pkgconfig
目录 0 2015-03-17 14:04 test_fft\fftw3\include
目录 0 2015-03-17 14:04 test_fft\fftw3\share
............此处省略8个文件信息
- 上一篇:Cunit单元测试工具的实践入门
- 下一篇:遗传算法约束条件的处理
相关资源
- IAR For ARM 7.3最新注册机
- 郭天祥ARM9视频教程
- IAR ARM 7.8破解
- IAR For ARM V5.5 注册机
- IAR for ARM 7.40 破解
- IAR For ARM 7.4 破解
- arm触摸屏与LCD校准程序三点校准法
- ARM嵌入式项目实战
- 基于ARM蓝牙传输源程序
- USB 驱动 让电脑与arm进行通信
- arm flash 烧写程序源码
- 基于LW IP的嵌入式串口服务器的设计与
- Studies In Vitro and In Vivo of Pharmacologica
- Research on pharmacological effects of lycorin
- ARM64 完整指令集,学习必备
- WINCC报表(OLE-DB Tag and Alarm Export_V8.x
- ARM9指令cache的verilog代码
- 基于ARM7处理器的CAN总线网络设计
- 基于ARM和GPRS的无线通信系统设计
- 关于proteus仿真arm7出现错误的问题
- 基于ARM7的LCD显示电压示波系统的设计
- 基于ARM7和DSP的逆变电源设计电路
- 基于ARM开发板的车辆检测系统控制单
- ARM7TDMI-S在嵌入式系统中的Bootloader代码
- ARM汇编指令集PDF
- 基于ARM7 PWM定时器的图像传感器时序信
- 基于ARM7的分布式远程测控系统设计
- LPC2100系列ARM7微控制器加密ARM芯片
- ARM7内核的中断屏蔽方法
- 基于ARM7TDMI微处理器的矿用电子皮带秤
评论
共有 条评论