资源简介
基于颜色特征的图像检索系统源代码。积分已调到最低,网站不让免费
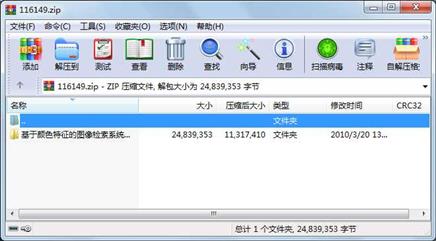
代码片段和文件信息
/******************************************************************
CqOctree.CPP
Performing Color Quantization using Octree algorithm
The 2 functions for global use is
HPALETTE CreateOctreePalette (HBITMAP hImage UINT nMaxColors UINT nColorBits)
HPALETTE CreateOctreePalette (LPSTR lpDIB UINT nMaxColors UINT nColorBits)
For using convenience define it in DIBAPI.H
******************************************************************/
#include “stdafx.h“
#include “dibapi.h“
// structure use internally
// store the necessary info of a node in octree
typedef struct _NODE
{
BOOL bIsLeaf; // TRUE if node has no children
UINT nPixelCount; // Number of pixels represented by this leaf
UINT nRedSum; // Sum of red components
UINT nGreenSum; // Sum of green components
UINT nBlueSum; // Sum of blue components
struct _NODE* pChild[8]; // Pointers to child nodes
struct _NODE* pNext; // Pointer to next reducible node
} NODE;
// Function prototypes
//Global use define it in dibapi.h
//HPALETTE CreateOctreePalette (HDIB hDIB UINT nMaxColors UINT nColorBits)
//HPALETTE CreateOctreePalette (LPSTR lpDIB UINT nMaxColors UINT nColorBits)
//Local use only
HPALETTE BuildOctreePalette(HANDLE hImage UINT nMaxColors UINT nColorBits);
void AddColor (NODE** BYTE BYTE BYTE UINT UINT UINT* NODE**);
NODE* CreateNode (UINT UINT UINT* NODE**);
void ReduceTree (UINT UINT* NODE**);
void DeleteTree (NODE**);
void GetPaletteColors (NODE* PALETTEENTRY* UINT*);
int GetRightShiftCount (DWORD);
int GetLeftShiftCount (DWORD);
// Function body
/*************************************************************************
*
* CreateOctreePalette()
*
* Parameters:
*
* HDIB hDIB - Handle to source DIB
* UINT nMaxColors - destination color number
* UINT nColorBits - destination color bits
*
* Return Value:
*
* HPALETTE - Handle to the result palette
*
* Description:
*
* This function use Octree color quantization algorithm to get
* optimal m kinds of color to represent total n kinds of color
* in a DIB and use the m kinds of color to build a palette.
* With the palette we can display the DIB on reasonable accuracy.
*
************************************************************************/
HPALETTE CreateOctreePalette(HDIB hDIB UINT nMaxColors UINT nColorBits)
{
HANDLE hImage;
hImage = DIBToDIBSection(hDIB);
if (! hImage)
return NULL;
return BuildOctreePalette(hImage nMaxColors nColorBits);
}
/*************************************************************************
*
* CreateOctreePalette()
*
* Parameters:
*
* LPBYTE lpDIB - Pointer to DIB data buffer
* UINT nMaxColors - destination color number
* UINT nColorBits - destination color bits
*
* Return Value:
*
* HPALETTE
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2010-03-20 13:33 基于颜色特征的图像检索系统源代码\
目录 0 2010-06-18 09:33 基于颜色特征的图像检索系统源代码\image.orig\
文件 35612 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\0.jpg
文件 24182 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\1.jpg
文件 32244 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\10.jpg
文件 46832 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\100.jpg
文件 28823 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\101.jpg
文件 26552 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\102.jpg
文件 24453 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\103.jpg
文件 35137 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\104.jpg
文件 30241 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\105.jpg
文件 28958 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\191.jpg
文件 24858 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\192.jpg
文件 45954 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\193.jpg
文件 23703 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\194.jpg
文件 27456 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\195.jpg
文件 49191 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\196.jpg
文件 27869 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\197.jpg
文件 28652 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\198.jpg
文件 18617 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\199.jpg
文件 42708 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\2.jpg
文件 28978 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\20.jpg
文件 28968 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\200.jpg
文件 24443 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\201.jpg
文件 25964 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\202.jpg
文件 35462 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\203.jpg
文件 35069 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\204.jpg
文件 26014 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\205.jpg
文件 32661 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\206.jpg
文件 23251 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\207.jpg
文件 24239 2001-07-07 04:02 基于颜色特征的图像检索系统源代码\image.orig\208.jpg
............此处省略197个文件信息
- 上一篇:基于STM32F4的霍尔编码器解析程序
- 下一篇:HCNA_R&S 题库
相关资源
- bp神经网络源代码,可直接运行
- 仿知乎界面小程序源代码
- 贪吃蛇源代码.fla
- dotnet 写字板 实验 源代码 不好请要不
- 图像二维小波变换的实现源代码
- 八三编码器设计 VHDL代码 简单,包附
- linux应用层的华容道游戏源代码
- 网上拍卖系统完整源代码
- CSMA/CD等动画演示加源代码
- silicon lab公司的收音IC SI47XX全套开发工
- 合同管理系统的源代码(附数据库)
- 用VC 编写的仿QQ聊天室程序源代码
- STM32F103 串口程序(完整版)
- VPC3_DPV1源代码,Profibus
- PB做的托盘程序(最小化后在左下角显
- 透明加密源码及说明
- 排队机叫号 源代码
- 五子棋C 源代码
- CAD LISP24个源代码
- 二叉树基本操作源代码
- 推箱子及人工智能寻路C 源代码
- opengl轮廓字体源代码
- 冈萨雷斯 数字图像处理 源代码(m文
- 直流伺服电机电路原理图(内附单片
- 哈哈冒险岛登入器源代码
- midi电子琴简单设计(附源代码).ra
- PESQ C源代码
- 画图程序MFC/VC/VC CRectTracker 串行化
- 莱卡 全站仪数据格式转换程序,有源
- HEX到Bin文件源代码
评论
共有 条评论