资源简介
计算机图形学(第三版)三维空间的几何变换代码:代码运行软件版本(Visual Studio 2015)【软件安装教程百度“VS2015安装+OpenGL环境配置及测试”】
参考书本代码227~229页代码,稍作修改,实现三维图形平移,旋转,缩放等几何变换。
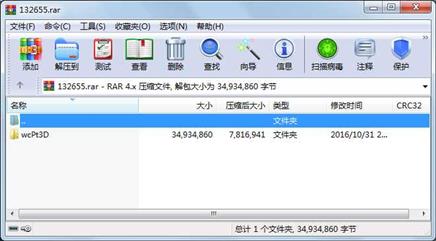
代码片段和文件信息
#include “Dependencies\glew\glew.h“
#include “Dependencies\freeglut\freeglut.h“
#include
#include
GLsizei winWidth = 600 winHeight = 600;
GLfloat xwcMin = 0.0 xwcMax = 225.0;
GLfloat ywcMin = 0.0 ywcMax = 225.0;
class wcPt3D {
public:
GLfloat x y z;
};
typedef GLfloat Matrix4x4[4][4];
Matrix4x4 matComposition;
const GLdouble pi = 3.14159;
void init(void) {
/*设置窗口显示颜色*/
glClearColor(0.0 0.0 0.0 0.0);
}
/*创建4X4的单位矩阵*/
void Matrix4x4SetIndentity(Matrix4x4 matIndent3x3)
{
GLint row col;
for (row = 0; row < 4; row++)
for (col = 0; col < 4; col++)
matIndent3x3[row][col] = (row == col);
}
/*矩阵m1左乘矩阵m2结果存储在m2中*/
void Matrix4x4PreMultiply(Matrix4x4 m1 Matrix4x4 m2)
{
GLint row col;
Matrix4x4 matTemp;
for (row = 0; row < 4; row++)
for (col = 0; col < 4; col++)
matTemp[row][col] = m1[row][0] * m2[0][col] + m1[row][1] * m2[1][col] + m1[row][2] * m2[2][col]+ m1[row][3] * m2[3][col];
for (row = 0; row < 4; row++)
for (col = 0; col < 4; col++)
m2[row][col] = matTemp[row][col];
}
void translate3D(GLfloat tx GLfloat ty GLfloat tz)
{
Matrix4x4 matTrans3D;
/*初始化一个单位矩阵*/
Matrix4x4SetIndentity(matTrans3D);
matTrans3D[0][2] = tx;
matTrans3D[1][2] = ty;
matTrans3D[2][3] = tz;
/*复合三维平移*/
Matrix4x4PreMultiply(matTrans3D matComposition);
}
void rotate3D(wcPt3D p1 wcPt3D p2GLfloat radianAngle)
{
Matrix4x4 matQuatRot;
GLfloat axisVectLength = sqrt((p2.x - p1.x)*(p2.x - p1.x) + (p2.y - p1.y)*(p2.y - p1.y) + (p2.z - p1.z)*(p2.z - p1.z));
GLfloat cosA = cosf(radianAngle);
GLfloat oneC = 1 - cosA;
GLfloat sinA = sinf(radianAngle);
GLfloat ux = (p2.x - p1.x) / axisVectLength;
GLfloat uy = (p2.y - p1.y) / axisVectLength;
GLfloat uz = (p2.z - p1.z) / axisVectLength;
//p1点平移至原点
translate3D(-p1.x -p1.y -p1.z);
/*初始化一个单位矩阵*/
Matrix4x4SetIndentity(matQuatRot);
matQuatRot[0][0] = ux*ux*oneC + cosA;
matQuatRot[0][1] = ux*uy*oneC - uz*sinA;
matQuatRot[0][2] = ux*uz*oneC + uy*sinA;
matQuatRot[1][0] = uy*ux*oneC + uz*sinA;
matQuatRot[1][1] = uy*uy*oneC + cosA;
matQuatRot[1][2] = uy*uz*oneC - ux*sinA;
matQuatRot[2][0] = uz*ux*oneC - uy*sinA;
matQuatRot[2][1] = uz*uy*oneC + ux*sinA;
matQuatRot[2][2] = uz*uz*oneC + cosA;
/*复合三维旋转*/
Matrix4x4PreMultiply(matQuatRot matComposition);
//逆平移
translate3D(p1.x p1.y p1.z);
}
void scale3D(GLfloat sx GLfloat sy GLfloat sz wcPt3D fixedPt)
{
Matrix4x4 matScale3D;
/*初始化一个单位矩阵*/
Matrix4x4SetIndentity(matScale3D);
matScale3D[0][0] = sx;
matScale3D[0][3] = (1 - sx)*fixedPt.x;
matScale3D[1][1] = sy;
matScale3D[1][3] = (1 - sy)*fixedPt.y;
matScale3D[2][2] = sz;
matScale3D[2][3] = (1 - sz)*fixedPt.z;
/*复合二维缩放*/
Matrix4x4PreMultiply(matScale3D matComposition);
}
/*利用复合矩阵计算改变的坐标*/
void transformVerts3D(GLint nVerts wcPt3D *verts)
{
GLint k;
GLfloat temp;
for (k = 0; k < nVerts; k++)
{
temp = mat
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
..A..H. 27648 2016-10-31 20:37 wcPt3D\.vs\wcPt3D\v14\.suo
文件 61440 2016-10-26 22:42 wcPt3D\Debug\wcPt3D.pdb
文件 330752 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\vc140.idb
文件 94208 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\vc140.pdb
文件 3225 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.log
文件 26785 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.obj
文件 754 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\CL.command.1.tlog
文件 14668 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\CL.read.1.tlog
文件 668 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\CL.write.1.tlog
文件 2 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\li
文件 2 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\li
文件 2 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\li
文件 2 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\li
文件 2 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\li
文件 2 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\li
文件 2 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\li
文件 0 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\unsuccessfulbuild
文件 226 2016-10-26 22:42 wcPt3D\wcPt3D\Debug\wcPt3D.tlog\wcPt3D.lastbuildstate
文件 703 2015-03-14 15:34 wcPt3D\wcPt3D\Dependencies\freeglut\freeglut.h
文件 36518 2015-03-14 15:24 wcPt3D\wcPt3D\Dependencies\freeglut\freeglut.lib
文件 10682 2015-03-14 15:34 wcPt3D\wcPt3D\Dependencies\freeglut\freeglut_ext.h
文件 27470 2015-07-22 08:45 wcPt3D\wcPt3D\Dependencies\freeglut\freeglut_std.h
文件 660 2015-03-14 15:34 wcPt3D\wcPt3D\Dependencies\freeglut\glut.h
文件 1038562 2015-08-10 21:54 wcPt3D\wcPt3D\Dependencies\glew\glew.h
文件 609776 2015-08-10 21:53 wcPt3D\wcPt3D\Dependencies\glew\glew32.lib
文件 74912 2015-08-10 21:54 wcPt3D\wcPt3D\Dependencies\glew\glxew.h
文件 64836 2015-08-10 21:54 wcPt3D\wcPt3D\Dependencies\glew\wglew.h
文件 6686 2016-10-31 20:37 wcPt3D\wcPt3D\wcPt3D.cpp
文件 8045 2016-10-26 22:44 wcPt3D\wcPt3D\wcPt3D.vcxproj
文件 958 2016-10-26 22:42 wcPt3D\wcPt3D\wcPt3D.vcxproj.filters
............此处省略36个文件信息
- 上一篇:架构师之路58沈剑
- 下一篇:BullseyeCoverage
评论
共有 条评论