资源简介
使用WiringPi可以驱动树莓派硬件底层,包含GPIO,usb,uart
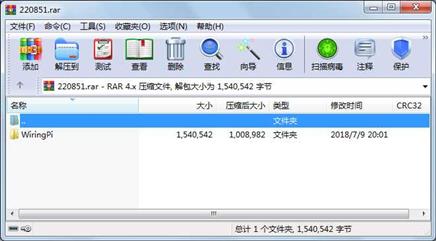
代码片段和文件信息
/*
* ds1302.c:
* Real Time clock
*
* Copyright (c) 2013 Gordon Henderson.
***********************************************************************
* This file is part of wiringPi:
* https://projects.drogon.net/raspberry-pi/wiringpi/
*
* wiringPi is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation either version 3 of the License or
* (at your option) any later version.
*
* wiringPi is distributed in the hope that it will be useful
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with wiringPi. If not see .
***********************************************************************
*/
#include
#include
#include
#include
#include
#include “ds1302.h“
// Register defines
#define RTC_SECS 0
#define RTC_MINS 1
#define RTC_HOURS 2
#define RTC_DATE 3
#define RTC_MONTH 4
#define RTC_DAY 5
#define RTC_YEAR 6
#define RTC_WP 7
#define RTC_TC 8
#define RTC_BM 31
// Locals
static int dPin cPin sPin ;
/*
* dsShiftIn:
* Shift a number in from the chip LSB first. Note that the data is
* sampled on the trailing edge of the last clock so it‘s valid immediately.
*********************************************************************************
*/
static unsigned int dsShiftIn (void)
{
uint8_t value = 0 ;
int i ;
pinMode (dPin INPUT) ; delayMicroseconds (1) ;
for (i = 0 ; i < 8 ; ++i)
{
value |= (digitalRead (dPin) << i) ;
digitalWrite (cPin HIGH) ; delayMicroseconds (1) ;
digitalWrite (cPin LOW) ; delayMicroseconds (1) ;
}
return value;
}
/*
* dsShiftOut:
* A normal LSB-first shift-out just slowed down a bit - the Pi is
* a bit faster than the chip can handle.
*********************************************************************************
*/
static void dsShiftOut (unsigned int data)
{
int i ;
pinMode (dPin OUTPUT) ;
for (i = 0 ; i < 8 ; ++i)
{
digitalWrite (dPin data & (1 << i)) ; delayMicroseconds (1) ;
digitalWrite (cPin HIGH) ; delayMicroseconds (1) ;
digitalWrite (cPin LOW) ; delayMicroseconds (1) ;
}
}
/*
* ds1302regRead: ds1302regWrite:
* Read/Write a value to an RTC Register or RAM location on the chip
*********************************************************************************
*/
static unsigned int ds1302regRead (const int reg)
{
unsigned int data ;
digitalWrite (sPin HIGH) ; delayMicroseconds (1) ;
dsShiftOut (reg) ;
data = dsShiftIn () ;
digitalWrite (sPin LOW) ; delayMicroseconds (1) ;
return data ;
}
static void ds1302regWrite (const int reg const unsign
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 266 2018-07-09 19:26 WiringPi\.git\config
文件 73 2018-07-09 19:25 WiringPi\.git\desc
文件 23 2018-07-09 19:26 WiringPi\.git\HEAD
文件 478 2018-07-09 19:25 WiringPi\.git\hooks\applypatch-msg.sample
文件 896 2018-07-09 19:25 WiringPi\.git\hooks\commit-msg.sample
文件 189 2018-07-09 19:25 WiringPi\.git\hooks\post-update.sample
文件 424 2018-07-09 19:25 WiringPi\.git\hooks\pre-applypatch.sample
文件 1642 2018-07-09 19:25 WiringPi\.git\hooks\pre-commit.sample
文件 1348 2018-07-09 19:25 WiringPi\.git\hooks\pre-push.sample
文件 4898 2018-07-09 19:25 WiringPi\.git\hooks\pre-reba
文件 1239 2018-07-09 19:25 WiringPi\.git\hooks\prepare-commit-msg.sample
文件 3610 2018-07-09 19:25 WiringPi\.git\hooks\update.sample
文件 17930 2018-07-09 19:26 WiringPi\.git\index
文件 240 2018-07-09 19:25 WiringPi\.git\info\exclude
文件 192 2018-07-09 19:26 WiringPi\.git\logs\HEAD
文件 192 2018-07-09 19:26 WiringPi\.git\logs\refs\heads\master
文件 192 2018-07-09 19:26 WiringPi\.git\logs\refs\remotes\origin\HEAD
文件 38424 2018-07-09 19:26 WiringPi\.git\ob
文件 719285 2018-07-09 19:26 WiringPi\.git\ob
文件 107 2018-07-09 19:26 WiringPi\.git\packed-refs
文件 41 2018-07-09 19:26 WiringPi\.git\refs\heads\master
文件 32 2018-07-09 19:26 WiringPi\.git\refs\remotes\origin\HEAD
文件 88 2018-07-09 19:26 WiringPi\.gitignore
文件 67 2018-07-09 20:01 WiringPi\.vscode\settings.json
文件 5162 2018-07-09 19:26 WiringPi\build
文件 7651 2018-07-09 19:26 WiringPi\COPYING.LESSER
文件 116 2018-07-09 19:26 WiringPi\debian\.gitignore
文件 191 2018-07-09 19:26 WiringPi\debian\changelog
文件 2 2018-07-09 19:26 WiringPi\debian\compat
文件 909 2018-07-09 19:26 WiringPi\debian\control
............此处省略232个文件信息
- 上一篇:优卡服装CAD
- 下一篇:SmartFoxServer中文教程及API
相关资源
- 树莓派B+_入门手册().pdf
- x264源码及其配置文件,用于配置树莓
-
Jli
nkV622c_ARM驱动 - 基于树莓派的可视化可远程遥控网络
- 树莓派运用CV摄像头、视觉巡线.zip
- MT7601(小度wifi360wifimiwif) staap linux驱
- 树莓派3b+学习使用教程
- 树莓派3b+装mate启动文件
- 婴幼儿监护系统的设计与实现
- wiringPi用户手册.pdf
- 最新树莓派开源原理图汇总
- 在树莓派上实现神经计算棒中
- 树莓派3B+原理图
- opencv-3.4.0编译失败需要的boostdesc_bgm
- 树莓派基础.pdf
- 清风带你学蓝牙 NRF51822 底层驱动详
- 树莓派麦克风模块—Adafruit I2S MEMS M
- 树莓派开源原理图汇总
- h5py-树莓派4B.zip
- 内存卡修复工具SDFormatter4.0树莓派玩家
- paho.mqtt.c 树莓派交叉编译版本SDK
- 支持科大讯飞语音识别的树莓派版本
- 树莓派3B+使用手册
- BCM2837- 树莓派3B 芯片手册.pdf
- 安卓端遥控树莓派小车APP
- Xware1.0.31_armel_v5te_glibc.zip
- 树莓派3b+Ubuntumate16.04彩虹屏解决办法
- Onboard SDK开发流程202006111606.pdf
- 2711_1p0- 树莓派4B 芯片手册.pdf
- Ubuntu mate 树莓派引导root文件
评论
共有 条评论