资源简介
(使用Python实现,注释详尽)在词法分析器的基础上,采用递归下降的方法实现算术表达式的语法分析器,以加深对自上而下语法分析过程的理解。
1、对算术表达式文法:
E→TE'
E'→+TE'| -TE' |ε
T→FT'
T'→*FT'| /FT' |ε
F→(E) | id |num
构造其递归下降分析程序。
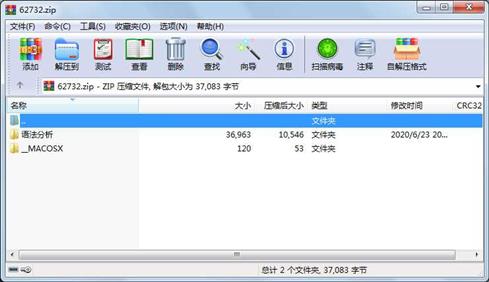
代码片段和文件信息
#!/user/bin/env python3
# -*- coding: utf-8 -*-
‘‘‘
@Copyright:Zhixing Tu
@Date:2020/6/4 6:34 下午
@File:Lab_2.py
@IDE:PyCharm
‘‘‘
‘‘‘
在实验一的基础上进行语法分析
E→TE‘
E‘→+TE‘| -TE‘ |ε
T→FT‘
T‘→*FT‘| /FT‘ |ε
F→(E) | id |num
保留关键字及种别编码
Static_word = {“begin“: 1 “end“: 2 “if“: 3 “then“: 4 “while“: 5
“do“: 6 “const“: 7 “var“: 8 “call“: 9 “procedure“: 10}
算符和界符及种别编码
Punctuation_marks = {“+“: 11 “-“: 12 “*“: 13 “/“: 14 “odd“: 15 “=“: 16 “<>“: 17 “<“: 18 “>“: 19
“<=“: 20 “>=“: 21 “:=“: 22 “(“: 23 “)“: 24 “.“: 25 ““: 26 “;“: 27}
常数的种别编码为28,标识符的种别编码为29,非法字符的种别编码为30
‘‘‘
# 导入词法分析的程序
from PL0_System_Lex import *
# 由于实验二的特殊性,单独把i(id)当做保留字处理
Static_word[“i“] = 0
# i + - * 、 ( ) num的种别编码
Lab2_word = [0 11 12 13 14 23 24 28]
# 出错标志
Err = 0
# 位置标志
Index = 0
# 算术表达式是否符合文法
Correct = “CorrectThe arithmetic expression meets the grammatical requirements!“
Error = “ErrorThe arithmetic expression does not meet the grammatical requirements!“
# 语法分析结果写入文件
def WriteFile(string):
fW = open(pathW ‘a‘)
fW.write(string + “\n“)
fW.close()
# 开始正式语法分析前首先判断该算术表达式中的各个字符以及首尾字符是否符合要求
def First():
index = 0
if (Result_Lex[0][0] in [0 23 28]) and (Result_Lex[0][-1] in [0 24 28]):
for i in Result_Lex[:-2]:
if i not in Lab2_word:
index += 1
break
else:
continue
else:
index += 1
return index
# E→TE‘
def E():
global Err
if Err == 0:
T()
E1()
# E‘→+TE‘| -TE‘ |ε
def E1():
global Err Index
if Err == 0 and Index != len(Result_Lex[0]):
if Result_Lex[0][Index] in [11 12]:
Index += 1
if Index != len(Result_Lex[0]) - 1:
T()
E1()
else:
Index = len(Result_Lex[0])
elif Result_Lex[0][Index] != 24:
Err = 1
# T→FT‘
def T():
global Err
if Err == 0:
F()
T1()
# T‘→*FT‘| /FT‘ |ε
def T1():
global Err Index
if Err == 0 and Index != len(Result_Lex[0]):
if Result_Lex[0][Index] in [13 14]:
Index += 1
if Index != len(Result_Lex[0]) - 1:
F()
T1()
else:
Index = len(Result_Lex[0])
elif Result_Lex[0][Index] not in [11 12 24]:
Err = 1
# F→(E) | id |num
def F():
global Err Index
if Err == 0:
if Result_Lex[0][Index] in [0 28]:
Index += 1
elif Result_Lex[0][Index] == 23:
Index += 1
E()
if Result_Lex[0][Index] != 24:
Err = 1
Index += 1
else:
Err = 1
# 分析主程序
def Analysis_Gs():
global Err Index
if First() == 0:
F()
while Index < len(Result_Lex[0]):
if Result_Lex[0][Index] in [11 12]:
E1()
elif Result_Lex[0][Index] in [13 14]:
T1
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2020-06-23 12:59 璇硶鍒嗘瀽\
文件 6148 2020-06-15 06:06 璇硶鍒嗘瀽\.DS_Store
文件 120 2020-06-15 06:06 __MACOSX\璇硶鍒嗘瀽\._.DS_Store
目录 0 2020-06-23 12:59 璇硶鍒嗘瀽\TestLab2\
目录 0 2020-06-23 12:59 璇硶鍒嗘瀽\__pycache__\
文件 3784 2020-06-18 11:25 璇硶鍒嗘瀽\Lab_2.py
文件 8152 2020-06-04 11:35 璇硶鍒嗘瀽\PL0_System_Lex.py
目录 0 2020-06-23 12:59 璇硶鍒嗘瀽\Result_2\
目录 0 2020-06-23 12:59 璇硶鍒嗘瀽\.idea\
文件 2 2020-06-04 17:34 璇硶鍒嗘瀽\TestLab2\demo6.txt
文件 7 2020-06-04 17:08 璇硶鍒嗘瀽\TestLab2\demo4.txt
文件 5 2020-06-04 17:21 璇硶鍒嗘瀽\TestLab2\demo5.txt
文件 16 2020-06-04 17:06 璇硶鍒嗘瀽\TestLab2\demo1.txt
文件 14 2020-06-18 11:53 璇硶鍒嗘瀽\TestLab2\demo2.txt
文件 25 2020-06-04 17:07 璇硶鍒嗘瀽\TestLab2\demo3.txt
文件 4458 2020-06-04 11:40 璇硶鍒嗘瀽\__pycache__\PL0_System_Lex.cpython-37.pyc
文件 283 2020-06-18 11:56 璇硶鍒嗘瀽\Result_2\5.txt
文件 1037 2020-06-18 11:55 璇硶鍒嗘瀽\Result_2\3.txt
文件 588 2020-06-18 11:54 璇硶鍒嗘瀽\Result_2\2.txt
文件 707 2020-06-18 11:52 璇硶鍒嗘瀽\Result_2\1.txt
目录 0 2020-06-23 12:59 璇硶鍒嗘瀽\.idea\inspectionProfiles\
文件 284 2020-06-01 10:16 璇硶鍒嗘瀽\.idea\璇硶鍒嗘瀽.iml
文件 176 2020-06-01 10:16 璇硶鍒嗘瀽\.idea\.gitignore
文件 10128 2020-06-19 01:47 璇硶鍒嗘瀽\.idea\workspace.xm
文件 276 2020-06-01 10:16 璇硶鍒嗘瀽\.idea\modules.xm
文件 289 2020-06-01 10:16 璇硶鍒嗘瀽\.idea\misc.xm
文件 174 2020-06-01 10:16 璇硶鍒嗘瀽\.idea\inspectionProfiles\profiles_settings.xm
文件 410 2020-06-01 10:16 璇硶鍒嗘瀽\.idea\inspectionProfiles\Project_Default.xm
- 上一篇:用Python网易云音乐
- 下一篇:DNN判断句子的通顺程度.py
评论
共有 条评论