资源简介
DES算法的C++实现,VS整个项目打包,包括DES实现类与测试用例,是本人在校的信息安全课程实验之一。IDE使用了Visual Studio 2015 Community。
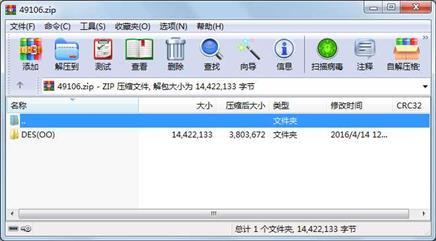
代码片段和文件信息
#include
#include “DES.h“
using namespace std;
#define BIT bool
BIT keys[16][48];
// 函数声明
static void permute(BIT *input BIT *output int *box int length);
static void inital_permute(BIT *input BIT *output);
static void final_permute(BIT *input BIT *output);
static void generate_keys(BIT key[64]);
static void encrypt_every_turn(BIT left_data[32] BIT right_data[32] BIT key[48] int turn);
static void encrypt_or_decrypt(BIT input[64] BIT output[64] BIT key[64] bool isEncrypt);
// 加密
void DES::des_encrypt(BIT input[64] BIT output[64] BIT key[64]) {
encrypt_or_decrypt(input output key true);
}
// 解密
void DES::des_decrypt(BIT input[64] BIT output[64] BIT key[64]) {
encrypt_or_decrypt(input output key false);
}
// 封装好的置换函数
void permute(BIT *input BIT *output int *box int length) {
for (int i = 0; i < length; ++i) {
output[i] = input[box[i] - 1];
}
}
// 初始置换
void inital_permute(BIT *input BIT *output) {
static int IP[64] = {
58 50 42 34 26 18 10 2
60 52 44 36 28 20 12 4
62 54 46 38 30 22 14 6
64 56 48 40 32 24 16 8
57 49 41 33 25 17 9 1
59 51 43 35 27 19 11 3
61 53 45 37 29 21 13 5
63 55 47 39 31 23 15 7 };
permute(input output IP 64);
}
// 最终置换
void final_permute(BIT *input BIT *output) {
static int FP[64] = {
40 8 48 16 56 24 64 32
39 7 47 15 55 23 63 31
38 6 46 14 54 22 62 30
37 5 45 13 53 21 61 29
36 4 44 12 52 20 60 28
35 3 43 11 51 19 59 27
34 2 42 10 50 18 58 26
33 1 41 9 49 17 57 25 };
permute(input output FP 64);
}
// 生成加密密钥
void generate_keys(BIT key[64]) {
// KP:密钥置换选择1(64->56)
static int KP[56] = {
57 49 41 33 25 17 9 1
58 50 42 34 26 18 10 2
59 51 43 35 27 19 11 3
60 52 44 36 63 55 47 39
31 23 15 7 62 54 46 38
30 22 14 6 61 53 45 37
29 21 13 5 28 20 12 4 };
// KM:每轮生成密钥的位移
static int KM[16] = {
1 1 2 2 2 2 2 2
1 2 2 2 2 2 2 1 };
// CP:密钥置换选择2(56->48)
static int CP[48] = {
14 17 11 24 1 5 3 28
15 6 21 10 23 19 12 4
26 8 16 7 27 20 13 2
41 52 31 37 47 55 30 40
51 45 33 48 44 49 39 56
34 53 46 42 50 36 29 32 };
BIT L[60] R[60];
// 1. 密钥置换选择1(64->56)
for (int i = 0; i < 28; ++i) {
L[i + 28] = L[i] = key[KP[i] - 1]
R[i + 28] = R[i] = key[KP[i + 28] - 1];
}
// 2. 密钥位移、置换选择2(56->48)
int shift = 0; // 密钥位移量
for (int i = 0; i < 16; ++i) {
shift += KM[i];
for (int j = 0; j < 48; ++
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2016-04-14 12:44 DES(OO)\
目录 0 2016-04-11 21:47 DES(OO)\.vs\
目录 0 2016-04-11 21:47 DES(OO)\.vs\DES(OO)\
目录 0 2016-04-11 21:47 DES(OO)\.vs\DES(OO)\v14\
文件 31744 2016-04-14 12:44 DES(OO)\.vs\DES(OO)\v14\.suo
目录 0 2016-04-14 12:44 DES(OO)\DES(OO)\
文件 7478 2016-04-11 21:54 DES(OO)\DES(OO)\DES(OO).vcxproj
文件 1148 2016-04-11 21:54 DES(OO)\DES(OO)\DES(OO).vcxproj.filters
文件 7385 2016-04-11 23:13 DES(OO)\DES(OO)\DES.cpp
文件 186 2016-04-14 12:44 DES(OO)\DES(OO)\DES.h
目录 0 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\
文件 289 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\DES(OO).log
目录 0 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\DES(OO).tlog\
文件 1502 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\DES(OO).tlog\CL.command.1.tlog
文件 26354 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\DES(OO).tlog\CL.read.1.tlog
文件 2342 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\DES(OO).tlog\CL.write.1.tlog
文件 227 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\DES(OO).tlog\DES(OO).lastbuildstate
文件 1538 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\DES(OO).tlog\li
文件 3140 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\DES(OO).tlog\li
文件 820 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\DES(OO).tlog\li
文件 42997 2016-04-11 23:13 DES(OO)\DES(OO)\Debug\DES.obj
文件 249541 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\Main.obj
文件 429056 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\vc140.idb
文件 413696 2016-04-12 11:45 DES(OO)\DES(OO)\Debug\vc140.pdb
文件 4127 2016-04-14 12:39 DES(OO)\DES(OO)\Main.cpp
文件 11010048 2016-04-14 12:44 DES(OO)\DES(OO).sdf
文件 1303 2016-04-11 21:47 DES(OO)\DES(OO).sln
目录 0 2016-04-12 11:24 DES(OO)\Debug\
文件 96768 2016-04-12 11:45 DES(OO)\Debug\DES(OO).exe
文件 677324 2016-04-12 11:45 DES(OO)\Debug\DES(OO).ilk
文件 1413120 2016-04-12 11:45 DES(OO)\Debug\DES(OO).pdb
............此处省略0个文件信息
相关资源
- C++中头文件与源文件的作用详解
- C++多线程网络编程Socket
- VC++ 多线程文件读写操作
- CCS FFT c语言算法
- 利用C++哈希表的方法实现电话号码查
- 移木块游戏,可以自编自玩,vc6.0编写
- 小波变换算法 c语言版
- 3des加密算法C语言实现
- C++纯文字DOS超小RPG游戏
- DES加密算法C语言实现
- VC++MFC小游戏实例教程(实例)+MFC类库
- 线性回归算法c语言实现
- 基于C语言的模拟退火算法
- C语言实现的DES对称加密算法
- 用VC6.0实现多边形扫描线填充算法
- c语言编写的货郎担算法
- 连铸温度场计算程序(C++)
- 6自由度机器人运动学正反解C++程序
- Em算法(使用C++编写)
- libstdc++-4.4.7-4.el6.i686.rpm
- VC++实现CMD命令执行与获得返回信息
- 白话C++(全)
- C++标准库第1、2
- 大数类c++大数类
- C++语言编写串口调试助手
- c++素数筛选法
- C++ mqtt 用法
- 商品库存管理系统 C++ MFC
- c++ 多功能计算器
- C++17 In Detail
评论
共有 条评论