资源简介
vs2015 c++ opencv3.3.1 实现 Interactive graph cuts for optimal boundary & region segmentation of objects in N-D images code
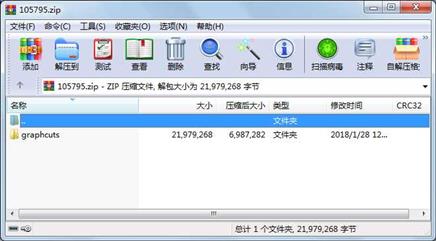
代码片段和文件信息
/* graph.cpp */
#include
#include
#include
#include “graph.h“
template
Graph::Graph(int node_num_max int edge_num_max void (*err_function)(char *))
: node_num(0)
nodeptr_block(NULL)
error_function(err_function)
{
if (node_num_max < 16) node_num_max = 16;
if (edge_num_max < 16) edge_num_max = 16;
nodes = (node*) malloc(node_num_max*sizeof(node));
arcs = (arc*) malloc(2*edge_num_max*sizeof(arc));
if (!nodes || !arcs) { if (error_function) (*error_function)(“Not enough memory!“); exit(1); }
node_last = nodes;
node_max = nodes + node_num_max;
arc_last = arcs;
arc_max = arcs + 2*edge_num_max;
maxflow_iteration = 0;
flow = 0;
}
template
Graph::~Graph()
{
if (nodeptr_block)
{
delete nodeptr_block;
nodeptr_block = NULL;
}
free(nodes);
free(arcs);
}
template
void Graph::reset()
{
node_last = nodes;
arc_last = arcs;
node_num = 0;
if (nodeptr_block)
{
delete nodeptr_block;
nodeptr_block = NULL;
}
maxflow_iteration = 0;
flow = 0;
}
template
void Graph::reallocate_nodes(int num)
{
int node_num_max = (int)(node_max - nodes);
node* nodes_old = nodes;
node_num_max += node_num_max / 2;
if (node_num_max < node_num + num) node_num_max = node_num + num;
nodes = (node*) realloc(nodes_old node_num_max*sizeof(node));
if (!nodes) { if (error_function) (*error_function)(“Not enough memory!“); exit(1); }
node_last = nodes + node_num;
node_max = nodes + node_num_max;
if (nodes != nodes_old)
{
arc* a;
for (a=arcs; a {
a->head = (node*) ((char*)a->head + (((char*) nodes) - ((char*) nodes_old)));
}
}
}
template
void Graph::reallocate_arcs()
{
int arc_num_max = (int)(arc_max - arcs);
int arc_num = (int)(arc_last - arcs);
arc* arcs_old = arcs;
arc_num_max += arc_num_max / 2; if (arc_num_max & 1) arc_num_max ++;
arcs = (arc*) realloc(arcs_old arc_num_max*sizeof(arc));
if (!arcs) { if (error_function) (*error_function)(“Not enough memory!“); exit(1); }
arc_last = arcs + arc_num;
arc_max = arcs + arc_num_max;
if (arcs != arcs_old)
{
node* i;
arc* a;
for (i=nodes; i {
if (i->first) i->first = (arc*) ((char*)i->first + (((char*) arcs) - ((char*) arcs_old)));
}
for (a=arcs; a {
if (a->next) a->next = (arc*) ((char*)a->next + (((char*) arcs) - ((char*) arcs_old)));
a->sister = (arc*) ((char*)a->sister + (((char*) arcs) - ((char*) arcs_old)));
}
}
}
#include “instances.inc“
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2018-01-28 12:39 graphcuts\
目录 0 2018-01-30 18:58 graphcuts\graphcuts\
目录 0 2018-01-28 12:39 graphcuts\graphcuts\.vs\
目录 0 2018-01-28 12:39 graphcuts\graphcuts\.vs\graphcuts\
目录 0 2018-01-28 12:39 graphcuts\graphcuts\.vs\graphcuts\v14\
文件 33792 2018-01-30 18:58 graphcuts\graphcuts\.vs\graphcuts\v14\.suo
目录 0 2018-01-28 12:40 graphcuts\graphcuts\Debug\
文件 105984 2018-01-28 12:40 graphcuts\graphcuts\Debug\graphcuts.exe
文件 387060 2018-01-28 12:40 graphcuts\graphcuts\Debug\graphcuts.ilk
文件 921600 2018-01-28 12:40 graphcuts\graphcuts\Debug\graphcuts.pdb
目录 0 2018-01-30 18:58 graphcuts\graphcuts\graphcuts\
文件 1573 2018-01-28 12:43 graphcuts\graphcuts\graphcuts.sln
文件 13398016 2018-01-30 18:58 graphcuts\graphcuts\graphcuts.VC.db
文件 12549 2018-01-29 10:58 graphcuts\graphcuts\graphcuts\0.jpg
文件 129945 2018-01-25 20:52 graphcuts\graphcuts\graphcuts\1.jpg
文件 10743 2018-01-28 14:35 graphcuts\graphcuts\graphcuts\1123.jpg
文件 7220 2018-01-25 19:57 graphcuts\graphcuts\graphcuts\block.h
文件 1302 2018-01-25 19:57 graphcuts\graphcuts\graphcuts\CHANGES.TXT
目录 0 2018-01-28 12:43 graphcuts\graphcuts\graphcuts\Debug\
文件 89316 2018-01-28 12:40 graphcuts\graphcuts\graphcuts\Debug\graph.obj
文件 229 2018-01-28 12:43 graphcuts\graphcuts\graphcuts\Debug\graphcuts.log
目录 0 2018-01-28 12:43 graphcuts\graphcuts\graphcuts\Debug\graphcuts.tlog\
文件 2662 2018-01-28 12:43 graphcuts\graphcuts\graphcuts\Debug\graphcuts.tlog\CL.command.1.tlog
文件 17058 2018-01-28 12:43 graphcuts\graphcuts\graphcuts\Debug\graphcuts.tlog\CL.read.1.tlog
文件 2826 2018-01-28 12:43 graphcuts\graphcuts\graphcuts\Debug\graphcuts.tlog\CL.write.1.tlog
文件 209 2018-01-28 12:43 graphcuts\graphcuts\graphcuts\Debug\graphcuts.tlog\graphcuts.lastbuildstate
文件 1566 2018-01-28 12:40 graphcuts\graphcuts\graphcuts\Debug\graphcuts.tlog\li
文件 3320 2018-01-28 12:40 graphcuts\graphcuts\graphcuts\Debug\graphcuts.tlog\li
文件 806 2018-01-28 12:40 graphcuts\graphcuts\graphcuts\Debug\graphcuts.tlog\li
文件 0 2018-01-28 12:43 graphcuts\graphcuts\graphcuts\Debug\graphcuts.tlog\unsuccessfulbuild
文件 162525 2018-01-28 12:40 graphcuts\graphcuts\graphcuts\Debug\maxflow.obj
............此处省略35个文件信息
相关资源
- Qt画图工具源码(qgraphics draw)
- 基于图割的图像分割OpenCV+MFC实现
- C语言进阶源码---基于graphics实现图书
- C++编写的模拟流体运动
- stm32L系列F系列 加密库Cryptographic lib
- ntGraph.ocx控件
- Boost Graph Library:The User Guide and Referen
- Cryptography in C and C++
- easyx做的时钟
- libbgi.a、BIOS.H和graphics.h
- SelectiveSearch2
- Computer Graphics Programming in OpenGL with C
- 《数据机构与算法》三级项目 - 开发
- c++ 直线裁剪、画矩形等(graphics)
- c语言扫雷游戏graphics.h实现
- Carve CSG 几何造型库
- c语言编写中国象棋人人对战graphic.h
- graphics图像库
- C语言graphics图形库
- graphics.h C语言图形学,画星星,画月
- Graphchi下BFS实现
- gco-v3.0代码
- graphics的随笔画程序基于c语言
- 图像分割—基于图的图像分割Graph-b
- 贪吃蛇C++ graphics.h
- c语言画图及小动画制作
- graphics.h,bios.h和libbgi.a
- graphics+winbgi
- Algorithms.in.C++.Part.5.Graph.Algorithms
- C语言Graphics.h头文件
评论
共有 条评论