资源简介
树莓派摄像头资料和例程。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。。
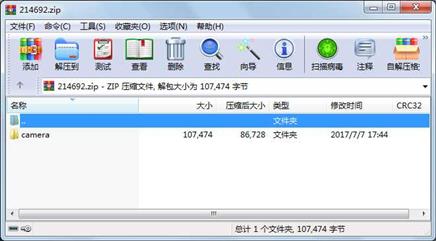
代码片段和文件信息
# Point-and-shoot camera for Raspberry Pi w/camera and Adafruit PiTFT.
# This must run as root (sudo python cam.py) due to framebuffer etc.
#
# Adafruit invests time and resources providing this open source code
# please support Adafruit and open-source development by purchasing
# products from Adafruit thanks!
#
# http://www.adafruit.com/products/998 (Raspberry Pi Model B)
# http://www.adafruit.com/products/1367 (Raspberry Pi Camera Board)
# http://www.adafruit.com/products/1601 (PiTFT Mini Kit)
# This can also work with the Model A board and/or the Pi NoIR camera.
#
# Prerequisite tutorials: aside from the basic Raspbian setup and
# enabling the camera in raspi-config you should configure WiFi (if
# using wireless with the Dropbox upload feature) and read these:
# PiTFT setup (the tactile switch buttons are not required for this
# project but can be installed if you want them for other things):
# http://learn.adafruit.com/adafruit-pitft-28-inch-resistive-touchscreen-display-raspberry-pi
# Dropbox setup (if using the Dropbox upload feature):
# http://raspi.tv/2013/how-to-use-dropbox-with-raspberry-pi
#
# Written by Phil Burgess / Paint Your Dragon for Adafruit Industries.
# BSD license all text above must be included in any redistribution.
import atexit
import cPickle as pickle
import errno
import fnmatch
import io
import os
import picamera
import pygame
import stat
import threading
import time
import yuv2rgb
from pygame.locals import *
from subprocess import call
# UI classes ---------------------------------------------------------------
# Small resistive touchscreen is best suited to simple tap interactions.
# Importing a big widget library seemed a bit overkill. Instead a couple
# of rudimentary classes are sufficient for the UI elements:
# Icon is a very simple bitmap class just associates a name and a pygame
# image (PNG loaded from icons directory) for each.
# There isn‘t a globally-declared fixed list of Icons. Instead the list
# is populated at runtime from the contents of the ‘icons‘ directory.
class Icon:
def __init__(self name):
self.name = name
try:
self.bitmap = pygame.image.load(iconPath + ‘/‘ + name + ‘.png‘)
except:
pass
# Button is a simple tappable screen region. Each has:
# - bounding rect ((XYWH) in pixels)
# - optional background color and/or Icon (or None) always centered
# - optional foreground Icon always centered
# - optional single callback function
# - optional single value passed to callback
# Occasionally Buttons are used as a convenience for positioning Icons
# but the taps are ignored. Stacking order is important; when Buttons
# overlap lowest/first Button in list takes precedence when processing
# input and highest/last Button is drawn atop prior Button(s). This is
# used for example to center an Icon by creating a passive Button the
# width of the full screen but with other buttons left or right that
# may take input precedence (e.g. the Effect label
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2017-07-07 17:44 camera\
文件 35 2015-03-06 15:28 camera\Camera
文件 1301 2015-03-06 15:28 camera\LICENSE
文件 376 2015-03-06 15:28 camera\Makefile
文件 249 2015-03-06 15:28 camera\README.md
文件 64 2015-03-06 15:28 camera\cam.pkl
文件 23125 2015-03-06 15:28 camera\cam.py
目录 0 2017-07-07 17:44 camera\icons\
文件 1324 2015-03-06 15:28 camera\icons\delete.png
文件 1135 2015-03-06 15:28 camera\icons\done.png
文件 6505 2015-03-06 15:28 camera\icons\empty.png
文件 1366 2015-03-06 15:28 camera\icons\fx-blur.png
文件 2023 2015-03-06 15:28 camera\icons\fx-cartoon.png
文件 2438 2015-03-06 15:28 camera\icons\fx-colorswap.png
文件 2076 2015-03-06 15:28 camera\icons\fx-denoise.png
文件 1748 2015-03-06 15:28 camera\icons\fx-emboss.png
文件 2005 2015-03-06 15:28 camera\icons\fx-film.png
文件 2307 2015-03-06 15:28 camera\icons\fx-gpen.png
文件 2171 2015-03-06 15:28 camera\icons\fx-hatch.png
文件 2195 2015-03-06 15:28 camera\icons\fx-negative.png
文件 1658 2015-03-06 15:28 camera\icons\fx-none.png
文件 1921 2015-03-06 15:28 camera\icons\fx-oilpaint.png
文件 1674 2015-03-06 15:28 camera\icons\fx-pastel.png
文件 2026 2015-03-06 15:28 camera\icons\fx-posterise.png
文件 2029 2015-03-06 15:28 camera\icons\fx-posterize.png
文件 1800 2015-03-06 15:28 camera\icons\fx-sketch.png
文件 1996 2015-03-06 15:28 camera\icons\fx-solarize.png
文件 2270 2015-03-06 15:28 camera\icons\fx-washedout.png
文件 2332 2015-03-06 15:28 camera\icons\fx-watercolor.png
文件 755 2015-03-06 15:28 camera\icons\fx.png
文件 1750 2015-03-06 15:28 camera\icons\gear.png
............此处省略40个文件信息
- 上一篇:国网GDW376.1规约报文解析工具
- 下一篇:爱尔兰损失B表计算
相关资源
- 树莓派B+_入门手册().pdf
- x264源码及其配置文件,用于配置树莓
-
Jli
nkV622c_ARM驱动 - 基于树莓派的可视化可远程遥控网络
- 树莓派运用CV摄像头、视觉巡线.zip
- MT7601(小度wifi360wifimiwif) staap linux驱
- 树莓派3b+学习使用教程
- 树莓派3b+装mate启动文件
- 婴幼儿监护系统的设计与实现
- wiringPi用户手册.pdf
- 最新树莓派开源原理图汇总
- 在树莓派上实现神经计算棒中
- 树莓派3B+原理图
- 树莓派底层驱动WiringPi代码 .rar
- opencv-3.4.0编译失败需要的boostdesc_bgm
- 树莓派基础.pdf
- 树莓派麦克风模块—Adafruit I2S MEMS M
- 树莓派开源原理图汇总
- h5py-树莓派4B.zip
- 内存卡修复工具SDFormatter4.0树莓派玩家
- paho.mqtt.c 树莓派交叉编译版本SDK
- 支持科大讯飞语音识别的树莓派版本
- 树莓派3B+使用手册
- BCM2837- 树莓派3B 芯片手册.pdf
- 安卓端遥控树莓派小车APP
- Xware1.0.31_armel_v5te_glibc.zip
- 树莓派3b+Ubuntumate16.04彩虹屏解决办法
- Onboard SDK开发流程202006111606.pdf
- 2711_1p0- 树莓派4B 芯片手册.pdf
- Ubuntu mate 树莓派引导root文件
评论
共有 条评论