资源简介
是学习DirectX的一本红龙书的原书代码,里边共含有19个章节的代码可供参考。
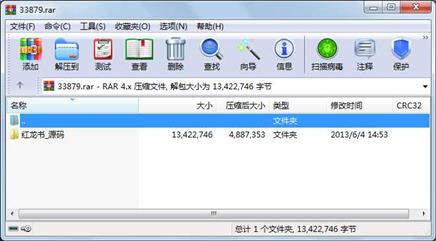
代码片段和文件信息
//////////////////////////////////////////////////////////////////////////////////////////////////
//
// File: d3dinit.cpp
//
// Author: Frank Luna (C) All Rights Reserved
//
// System: AMD Athlon 1800+ XP 512 DDR Geforce 3 Windows XP MSVC++ 7.0
//
// Desc: Demonstrates how to initialize Direct3D how to use the book‘s framework
// functions and how to clear the screen to black. Note that the Direct3D
// initialization code is in the d3dUtility.h/.cpp files.
//
//////////////////////////////////////////////////////////////////////////////////////////////////
#include “d3dUtility.h“
//
// Globals
//
IDirect3DDevice9* Device = 0;
//
// framework Functions
//
bool Setup()
{
// Nothing to setup in this sample.
return true;
}
void Cleanup()
{
// Nothing to cleanup in this sample.
}
bool Display(float timeDelta)
{
if( Device ) // Only use Device methods if we have a valid device.
{
// Instruct the device to set each pixel on the back buffer black -
// D3DCLEAR_TARGET: 0x00000000 (black) - and to set each pixel on
// the depth buffer to a value of 1.0 - D3DCLEAR_ZBUFFER: 1.0f.
Device->Clear(0 0 D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER 0x00000000 1.0f 0);
// Swap the back and front buffers.
Device->Present(0 0 0 0);
}
return true;
}
//
// WndProc
//
LRESULT CALLBACK d3d::WndProc(HWND hwnd UINT msg WPARAM wParam LPARAM lParam)
{
switch( msg )
{
case WM_DESTROY:
::PostQuitMessage(0);
break;
case WM_KEYDOWN:
if( wParam == VK_ESCAPE )
::DestroyWindow(hwnd);
break;
}
return ::DefWindowProc(hwnd msg wParam lParam);
}
//
// WinMain
//
int WINAPI WinMain(HINSTANCE hinstance
HINSTANCE prevInstance
PSTR cmdLine
int showCmd)
{
if(!d3d::InitD3D(hinstance
640 480 true D3DDEVTYPE_HAL &Device))
{
::MessageBox(0 “InitD3D() - FAILED“ 0 0);
return 0;
}
if(!Setup())
{
::MessageBox(0 “Setup() - FAILED“ 0 0);
return 0;
}
d3d::EnterMsgLoop( Display );
Cleanup();
Device->Release();
return 0;
}
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 31823 2003-05-03 15:44 红龙书_源码\Chapter 1\D3D9 Init\chap1_0.jpg
文件 2148 2003-03-08 10:30 红龙书_源码\Chapter 1\D3D9 Init\d3dInit.cpp
文件 4197 2003-03-08 10:32 红龙书_源码\Chapter 1\D3D9 Init\d3dUtility.cpp
文件 1263 2003-03-08 10:16 红龙书_源码\Chapter 1\D3D9 Init\d3dUtility.h
文件 5523 2002-08-08 12:44 红龙书_源码\Chapter 10\D3DXCreateMeshFVF\brick0.jpg
文件 4860 2002-08-08 12:44 红龙书_源码\Chapter 10\D3DXCreateMeshFVF\brick1.jpg
文件 41376 2003-05-03 15:57 红龙书_源码\Chapter 10\D3DXCreateMeshFVF\chap10_0.jpg
文件 14550 2002-10-09 18:25 红龙书_源码\Chapter 10\D3DXCreateMeshFVF\checker.jpg
文件 5828 2003-03-08 18:51 红龙书_源码\Chapter 10\D3DXCreateMeshFVF\d3dUtility.cpp
文件 2530 2003-03-08 18:51 红龙书_源码\Chapter 10\D3DXCreateMeshFVF\d3dUtility.h
文件 12262 2003-04-22 12:48 红龙书_源码\Chapter 10\D3DXCreateMeshFVF\d3dxcreatemeshfvf.cpp
文件 2574 2003-05-03 14:02 红龙书_源码\Chapter 10\D3DXCreateMeshFVF\Mesh Dump.txt
文件 52 2002-08-08 12:54 红龙书_源码\Chapter 10\D3DXCreateMeshFVF\texture credit.txt
文件 480915 2002-09-20 15:06 红龙书_源码\Chapter 11\Bounding Volumes\bigship1.x
文件 7705 2003-05-03 15:48 红龙书_源码\Chapter 11\Bounding Volumes\boundingvolumes.cpp
文件 41567 2003-05-03 15:57 红龙书_源码\Chapter 11\Bounding Volumes\chap11_2.jpg
文件 6340 2003-03-08 19:22 红龙书_源码\Chapter 11\Bounding Volumes\d3dUtility.cpp
文件 2985 2003-03-08 19:22 红龙书_源码\Chapter 11\Bounding Volumes\d3dUtility.h
文件 480915 2002-09-20 15:06 红龙书_源码\Chapter 11\Progressive Mesh\bigship1.x
文件 59454 2003-05-03 15:57 红龙书_源码\Chapter 11\Progressive Mesh\chap11_1.jpg
文件 5828 2003-03-08 19:19 红龙书_源码\Chapter 11\Progressive Mesh\d3dUtility.cpp
文件 2530 2003-03-08 19:19 红龙书_源码\Chapter 11\Progressive Mesh\d3dUtility.h
文件 7022 2003-05-03 15:48 红龙书_源码\Chapter 11\Progressive Mesh\pmesh.cpp
文件 480915 2002-09-20 15:06 红龙书_源码\Chapter 11\XFile\bigship1.x
文件 47023 2003-05-03 15:57 红龙书_源码\Chapter 11\XFile\chap11_0.jpg
文件 5828 2003-03-08 19:14 红龙书_源码\Chapter 11\XFile\d3dUtility.cpp
文件 2530 2003-03-08 19:14 红龙书_源码\Chapter 11\XFile\d3dUtility.h
文件 5239 2003-04-22 12:53 红龙书_源码\Chapter 11\XFile\xfile.cpp
文件 3822 2003-03-08 20:04 红龙书_源码\Chapter 12\Camera\camera.cpp
文件 1329 2003-03-08 20:04 红龙书_源码\Chapter 12\Camera\camera.h
............此处省略292个文件信息
相关资源
- 编译原理龙书答案
- 粒子系统画的心形线
- 龙书《编译原理》(Compilers:Principle
- Microsoft DirectX 8.0
- 20101028 DirectX(粒子系统改)
- 编译原理课件(龙书为教材).ppt267
- 编译原理龙书部分答案1-9
- 编译原理 第二版 龙书 习题答案
- DirectX 材质 模型 工具汉化版
- Directx 3D游戏 遥控飞机
- Dark GDK
- 运用DirectX9绘制太阳系
- Introduction to 3D Game Programming with Direc
- Directx12 游戏开发
- directx 10 龙书 中文版
- DirectX3D太阳系
- DirectX.9.0SDK中文版帮助文档
- 屏幕融合软件源码
- Microsoft DirectX SDK (June 2010)
- DelphiX 2000.07.17 For D7 (DirectX)
- DirectX 太阳系
- directX8.0 SDK
- DirectX.Capture ffdshow摄像头录制视频拍照
- 《DirectX特效游戏程序设计》中文版全
- Directx9 SDK
- [未整理]Direct X 11 3D 游戏开发编程基础
- DirectX版半条命MDL文件查看器
- DirectX 9.0 中文版传说中的龙书
- 实现三维图形绘制
- 太阳地球月亮旋转公转自转
评论
共有 条评论