资源简介
南开大学的编译原理期末作业,实现一个编译器,可以实现将程序源代码转换为汇编程序然后执行
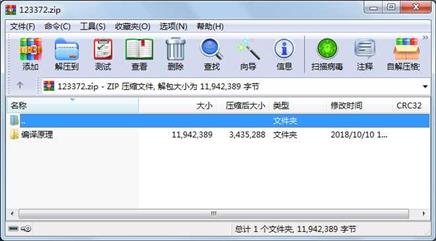
代码片段和文件信息
#include
#include
#include
#include
#include
#include
#include
#include
#include
using namespace std;
/**********类编号***********/
#define AC 1<<30
#define Keyword 1 //关键字
#define Identifier 2 //标识符
#define Integer 3 //无符号整数
#define Float 4 //无符号浮点数
#define ConstChar 5 //字符常量
#define ConstString 6 //字符串常量
#define Operator 7 //操作符
#define Punctuation 8 //标点符号
#define Logical 9 //逻辑运算符
#define Relational 10 //关系运算符
#define Comments 0 //注释
#define IllegalIdentifier -2 //非法标识符
#define IllegalFloat -4 //非法无符号浮点数
#define IllegalConstChar -5 //非法字符常量
#define IllegalConstString -6 //非法字符串常量
#define IllegalChar -8 //非法字符
const int number_of_keywords = 36; //关键字数目
const int max_of_argv = 20; //参数字符串最长长度
const int max_of_operator = 10; //操作符字符串最长长度
const int number_of_char = 15; //文法中出现的符号数量
const int number_of_grammer = 20; //文法数量
typedef struct KeyWordsTrie { //字典树结构体
int flag; //标记尾部
struct KeyWordsTrie* next[26]; //下一级指针
} KeyWordsTrie;
typedef struct Quadruple {
char op[max_of_operator]; //操作符字符串
char argv1[max_of_argv]; //参数1字符串
char argv2[max_of_argv]; //参数2字符串
char result[max_of_argv]; //结果字符串
}Quad;
typedef struct Word {//单词结构体
int type; //单词类型
char code; //单词代号
string value; //单词真值
bool operator==(struct Word ot) { //运算符重载 判断两个单词是否相等
if(type == ot.type && value == ot.value) {
return true;
}
return false;
}
}Word;
typedef struct Symbol { //符号结构体
Word word; //单词
int Place; //在符号表中位置
int TC; //真链
int FC; //假链
int Chain; //链,一般为假链
int LoopStart; //循环开始
}Sym;
/*********词法分析变量***********/
string text; //读入的代码
KeyWordsTrie *keywords; //关键字字典树
/*********语法分析变量***********/
string *G; //候选式内容
char *reduction; //第i个产生式应该被规约成的非终结符
int **SLR_Table; //负数:Sj正数:Rj零:error
char ch_num[128]; //存储不同字符对应的SLR(1)表的列
/*********语义分析变量**********/
int NXQ = 1; //当前四元式序号
int Ntemp = 0; //临时变量个数
vector quadList; //四元式列表
vector symbol_table; //符号表
Sym symbols[number_of_char];//归约符号表
void (*func[number_of_grammer+1])();//语义处理函数指针
/**************词法分析函数声明**************/
KeyWordsTrie* getKeyWordsTrie(); //构造关键字字典树
bool isKeyword(KeyWordsTrie* const string);//判断当前单词是否是关键字
bool isPunctuation(const char); //判断当前单词是否是标点符号
char getcharFrom(FILE*); //从源代码文件中获取一个字符
void retract(FILE*); //回退一个字符
Word Scanner(FILE*); //词法分析函数
/**************语法分析函数声明**************/
void readSLR(); //读入语法和它的SLR(1)信息
void freeMemeory(); //释放内存空间
void read(queue& FILE*);//读入要识别的句子
void grammar_analysis(FILE*);//语法分析总控程序
/*************语义处理函数声明***************/
int NewTemp(); //申请临时变量
int Entry(Word); //查询符号表,不存在则
void Gen(const char* int int int); //生成四元式
void BackPatch(int int); //回填
int Merge(int int); //归并真假链
int getIndex(char); //获得全局符号
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2018-10-10 14:11 编译原理\
目录 0 2018-10-09 14:39 编译原理\综合\
文件 24552 2018-10-09 13:58 编译原理\综合\Complier.cpp
文件 2208245 2018-10-09 14:39 编译原理\综合\Complier.exe
文件 61 2018-10-09 13:58 编译原理\综合\code.in
文件 5296 2018-10-09 14:40 编译原理\综合\complier.out
文件 1008 2018-10-09 13:59 编译原理\综合\ex
目录 0 2018-10-09 14:38 编译原理\词法分析\
文件 12367 2018-10-09 14:00 编译原理\词法分析\WordAnlayse.cpp
文件 1937148 2018-10-09 14:38 编译原理\词法分析\WordAnlayse.exe
文件 112 2018-10-09 14:39 编译原理\词法分析\code.in
文件 243 2018-10-09 14:39 编译原理\词法分析\words.out
目录 0 2018-10-09 13:59 编译原理\语义分析\
文件 24475 2018-10-09 13:59 编译原理\语义分析\SemanticAnlayse.cpp
文件 2136053 2018-10-09 13:59 编译原理\语义分析\SemanticAnlayse.exe
文件 24 2018-10-09 13:59 编译原理\语义分析\code.in
文件 1008 2018-10-09 13:59 编译原理\语义分析\ex
文件 490838 2018-10-09 13:58 编译原理\语义分析\语义分析.zip
目录 0 2018-10-09 14:00 编译原理\语法分析\
文件 16931 2018-10-09 13:59 编译原理\语法分析\GrammarAnlayse.cpp
文件 2080436 2018-10-09 14:00 编译原理\语法分析\GrammarAnlayse.exe
文件 24651 2018-10-09 13:59 编译原理\语法分析\SLR自动生成.cpp
文件 2470581 2018-10-09 14:00 编译原理\语法分析\SLR自动生成.exe
文件 26 2018-10-09 14:00 编译原理\语法分析\code.in
文件 124 2018-10-09 14:00 编译原理\语法分析\data.in
文件 22542 2018-10-09 13:59 编译原理\语法分析\data.out
文件 1008 2018-10-09 13:59 编译原理\语法分析\ex
文件 1772 2018-10-09 13:59 编译原理\语法分析\grammar.out
文件 2002 2018-10-09 13:59 编译原理\语法分析\if-else.info
文件 1923 2018-10-09 13:59 编译原理\语法分析\while.info
文件 478963 2018-10-09 13:59 编译原理\语法分析\语法分析.zip
............此处省略0个文件信息
相关资源
- 微软masm汇编编译器
- 编译原理实验工具及参考源码(lex&
- 类pascal语言编译器(编译原理实验)
- 编译原理课程设计:词法语法编译器
- 中科院 编译原理 习题及解答
- 编译原理四元式和逆波兰式
- 汇编语言编译器masm5.0
- 《编译原理》清华大学版中的pl0扩充
- PL/0功能扩充break功能
- 编译原理LR(0)语法分析
- 编译原理中间代码生成程序
- 编译原理:LR分析程序
- C编译器源代码(超级牛b).rar
- 编译原理实验:词法分析,语法分析
- 吉林大学编译原理课件
- 编译原理龙书答案
- 编译原理 第三章课后习题答案
- 易语言变量和数组的编译原理
- 编译原理语法分析器、词法分析器
- 山东大学编译原理PL/0语言 compiler实验
- FOR循环语句的翻译程序设计简单优先
- NFA的确定化NFA->DFA完整可运行代码
- pl/0编译器 语法分析
- 哈工大威海编译原理实验报告和源代
- 哈工大威海-编译原理实验报告和源码
- 编译原理课设c编译器
- 赋值语句翻译四元式
- 河北工业大学编译原理实验代码及实
- 完整C-编译器源代码
- TINY+编译器 改编自原版TINY
评论
共有 条评论