资源简介
<>医学图像分割,是由Yuri Y. Boykov和Marie-Pierre Jolly在IEEE发表的。
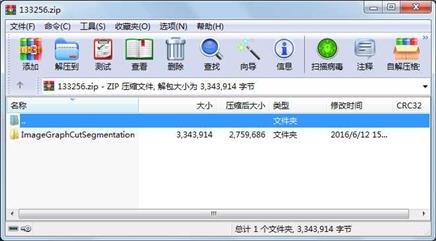
代码片段和文件信息
/*
Copyright (C) 2012 David Doria daviddoria@gmail.com
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation either version 3 of the License or
(at your option) any later version.
This program is distributed in the hope that it will be useful
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not see .
*/
#include “ImageGraphCut.h“
// Submodules
#include “Mask/ITKHelpers/Helpers/Helpers.h“
#include “Mask/ITKHelpers/ITKHelpers.h“
#include “itkImage.h“
#include “itkImageFileReader.h“
#include “itkImageFileWriter.h“
#include “itkImageRegionConstIteratorWithIndex.h“
#include “itkVectorImage.h“
int main(int argc char*argv[])
{
// Verify arguments
if(argc != 5)
{
std::cerr << “Required: image.png foregroundMask.png backgroundMask.png output.png“ << std::endl;
return EXIT_FAILURE;
}
// Parse arguments
std::string imageFilename = argv[1];
// This image should have white pixels indicating foreground pixels and be black elsewhere.
std::string foregroundFilename = argv[2];
// This image should have white pixels indicating background pixels and be black elsewhere.
std::string backgroundFilename = argv[3];
std::string outputFilename = argv[4]; // Foreground/background segment mask
// Output arguments
std::cout << “imageFilename: “ << imageFilename << std::endl
<< “foregroundFilename: “ << foregroundFilename << std::endl
<< “backgroundFilename: “ << backgroundFilename << std::endl
<< “outputFilename: “ << outputFilename << std::endl;
// The type of the image to segment
typedef itk::VectorImage ImageType;
// Read the image
typedef itk::ImageFileReader ReaderType;
ReaderType::Pointer reader = ReaderType::New();
reader->SetFileName(imageFilename);
reader->Update();
// Read the foreground and background stroke images
Mask::Pointer foregroundMask = Mask::New();
foregroundMask->ReadFromImage(foregroundFilename);
Mask::Pointer backgroundMask = Mask::New();
backgroundMask->ReadFromImage(backgroundFilename);
// Perform the cut
ImageGraphCut GraphCut;
GraphCut.SetImage(reader->GetOutput());
GraphCut.SetNumberOfHistogramBins(20);
GraphCut.SetLambda(.01);
std::vector > foregroundPixels = ITKHelpers::GetNonZeroPixels(foregroundMask.GetPointer());
std::vector > backgroundPixels = ITKHelpers::GetNonZeroPixels(backgroundMask.GetPointer());
GraphCut.SetSources(foregroundPixels);
GraphCut.SetSinks(backgroundPixels);
GraphCut.PerformSegmentation();
//
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2016-06-12 15:19 ImageGraphCutSegmentation\
文件 79 2016-06-12 10:44 ImageGraphCutSegmentation\.gitmodules
文件 2428 2016-06-12 10:45 ImageGraphCutSegmentation\CMakeLists.txt
目录 0 2016-06-12 15:17 ImageGraphCutSegmentation\data\
文件 1407 2016-06-12 10:40 ImageGraphCutSegmentation\data\background.png
文件 900 2016-06-12 10:41 ImageGraphCutSegmentation\data\foreground.png
文件 328509 2016-06-12 10:41 ImageGraphCutSegmentation\data\soldier.png
文件 2158 2016-06-12 10:41 ImageGraphCutSegmentation\data\soldier_ba
目录 0 2016-06-12 15:17 ImageGraphCutSegmentation\Examples\
文件 182 2016-06-12 10:23 ImageGraphCutSegmentation\Examples\CMakeLists.txt
文件 3125 2016-06-12 10:24 ImageGraphCutSegmentation\Examples\ImageGraphCutSegmentationExample.cpp
文件 4551 2016-06-12 10:45 ImageGraphCutSegmentation\ImageGraphCut.h
文件 17292 2016-06-12 10:46 ImageGraphCutSegmentation\ImageGraphCut.hpp
文件 2505274 2016-06-12 10:13 ImageGraphCutSegmentation\InteractiveImageGraphCutSegmentation.pdf
目录 0 2016-06-12 15:17 ImageGraphCutSegmentation\Kolmogorov\
文件 8622 2016-06-12 10:31 ImageGraphCutSegmentation\Kolmogorov\block.h
文件 89 2016-06-12 10:29 ImageGraphCutSegmentation\Kolmogorov\CMakeLists.txt
文件 2594 2016-06-12 10:31 ImageGraphCutSegmentation\Kolmogorov\graph.cpp
文件 6215 2016-06-12 10:32 ImageGraphCutSegmentation\Kolmogorov\graph.h
文件 11602 2016-06-12 10:34 ImageGraphCutSegmentation\Kolmogorov\maxflow.cpp
目录 0 2016-06-12 15:17 ImageGraphCutSegmentation\Kolmogorov\Tests\
文件 265 2016-06-12 10:27 ImageGraphCutSegmentation\Kolmogorov\Tests\CMakeLists.txt
文件 789 2016-06-12 10:28 ImageGraphCutSegmentation\Kolmogorov\Tests\MaxflowTest.cpp
目录 0 2016-06-12 15:17 ImageGraphCutSegmentation\Mask-8a8f2b7\
文件 6 2012-11-12 23:24 ImageGraphCutSegmentation\Mask-8a8f2b7\.gitignore
文件 96 2012-11-12 23:24 ImageGraphCutSegmentation\Mask-8a8f2b7\.gitmodules
文件 2840 2012-11-12 23:24 ImageGraphCutSegmentation\Mask-8a8f2b7\CMakeLists.txt
目录 0 2016-06-12 15:17 ImageGraphCutSegmentation\Mask-8a8f2b7\ITKHelpers @ d852923\
文件 31 2012-11-10 05:50 ImageGraphCutSegmentation\Mask-8a8f2b7\ITKHelpers @ d852923\.gitignore
文件 87 2012-11-10 05:50 ImageGraphCutSegmentation\Mask-8a8f2b7\ITKHelpers @ d852923\.gitmodules
文件 2374 2012-11-10 05:50 ImageGraphCutSegmentation\Mask-8a8f2b7\ITKHelpers @ d852923\CMakeLists.txt
............此处省略56个文件信息
- 上一篇:稳健的自适应波束形成
- 下一篇:ITK入门中文教程1
相关资源
- 基于OpenCV的数字识别468815
- 带式输送机托辊红外图像分割与定位
- 基于libsvm的图像分割代码
- 基于朴素贝叶斯分类法的图像分割
- 复杂背景与天气条件下的棉花叶片图
- 舌图像分割
- 图像分割方法在遥感图像分析中的应
-
Accurate subpixel edge location ba
sed on pa - 图像分割算法研究区域分割,数学形
- 基于图切算法的交互式图像分割技术
- 纹理图像分割论文+代码
- Dence CRF 条件随机场图像分割
- 图像分割-章毓晋
- Matalb图像分割边缘检测算子比较
- 交互式图像分割——算法与系统
- ITK入门教程_医学图像分割与配准_高清
- Canny算子分割遥感影像
- 分水岭图像分割算法C++程序源代码
- 基于形态学的图像分割 图片版
- CrackForest数据集
- 基于粒子群优算法的最大熵多阈值图
- 医学图像分割与配准(ITK实现 全2册)
- 章毓晋《图像分割》.PDF
- image segmentor source code and release 图像分
- 图像分割算法的实现
- 经典纹理图像分割论文+代码
- 基于PCNN的彩色图像自动分割毕业论文
- CUDA的图像分割并行算法的设计与实现
- 马尔科夫随机场图像分割ICM代码
- 纹理图像分割算法
评论
共有 条评论