资源简介
见http://blog.csdn.net/czjxy881/article/details/21228881
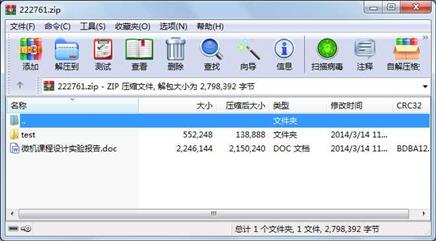
代码片段和文件信息
// Curvefit.cpp: implementation of the CBezierfit class.
//
//////////////////////////////////////////////////////////////////////
#include “stdafx.h“
#include
#include “Curvefit.h“
#ifdef _DEBUG
#undef THIS_FILE
static char THIS_FILE[]=__FILE__;
#define new DEBUG_NEW
#endif
static double DistanceError(const double *x const double *y const double *Rawdata int n);
static void Bezier(double u const double *a const double *b
double x4 double y4 double &z double &s);
void CalcBezier(const double *Rawdata int n double *Control)
{
double x[4];
double y[4];
double e1e2 e3;
int Retry;
double x1ax2ay1ay2a;
x[0] = Rawdata[0];
y[0] = Rawdata[1];
x[1] = Rawdata[2];
y[1] = Rawdata[3];
x[2] = Rawdata[n-4];
y[2] = Rawdata[n-3];
x[3] = Rawdata[n-2];
y[3] = Rawdata[n-1];
// seed with linear interpolation...
x[1] += x[1] - x[0];
y[1] += y[1] - y[0];
x[2] += x[2] - x[3];
y[2] += y[2] - y[3];
e1 = DistanceError(x y Rawdata n);
for (Retry = 1; Retry <= 2; Retry++)
{
// TRACE(“Retry %d\n“ Retry);
// TRACE(“ x1 y2 x2 y2 error\n“);
e3 = 0.5;
x1a = x[1];
while (fabs(e3) >= 0.01)
{
x[1] += (x[1] - x[0])*e3;
e2 = DistanceError(x y Rawdata n);
if (e2 == e1)
break;
if (e2 > e1)
{
x[1] = x1a;
e3 /=-3;
}
else
{
e1 = e2;
x1a = x[1];
}
}
e3 = 0.5;
y1a = y[1];
while (fabs(e3) >= 0.01)
{
y[1] += (y[1] - y[0])*e3;
e2 = DistanceError(x y Rawdata n);
if (e2 == e1)
break;
if (e2 > e1)
{
y[1] = y1a;
e3 /=-3;
}
else
{
e1 = e2;
y1a = y[1];
}
}
e3 = 0.5;
x2a = x[2];
while (fabs(e3) >= 0.01)
{
x[2] += (x[2] - x[3])*e3;
e2 = DistanceError(x y Rawdata n);
if (e2 == e1)
break;
if (e2 > e1)
{
x[2] = x2a;
e3 /=-3;
}
else
{
e1 = e2;
x2a = x[2];
}
}
e3 = 0.5;
y2a = y[2];
while (fabs(e3) >= 0.01)
{
y[2] += (y[2] - y[3])*e3;
e2 = DistanceError(x y Rawdata n);
if (e2 == e1)
break;
if (e2 > e1)
{
y[2] = y2a;
e3 /=-3;
}
else
{
e1 = e2;
y2a = y[2];
}
}
} // for
Control[0] = x[1];
Control[1] = y[1];
Control[2] = x[2];
Control[3] = y[2];
}
double DistanceError(const double *x const double *y
const double *Rawdata int n)
{
int i;
double a[4];
double b[4];
double u u1 u2;
double z z1 z2 s s1;
double temp;
double totalerror;
double stepsize;
double x4 y4;
totalerror = 0;
a[3] = (x[3]-x[0]+3*(x[1]-x[2]))/8;
b[3] = (y[3]-y[0]+3*(y[1]-y[2]))/8;
a[2] = (x[3]+x[0]-x[1]-x[2])*3/8;
b[2] = (y[3]+y[0]-y[1]-y[2])*3/8;
a[1] = (x[3]-x[0])/2 -a[3];
b[1] = (y[3]-y[0])/2 -b[3];
a[0] = (x[3]+x[0])/2 -a[2];
b[0] = (y[3]+y[0])/2 -b[2];
stepsize = 2.0/(n);
s = u1 = z1 = s1 = 0;
for (i = 2; i
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2014-03-14 11:36 test\
目录 0 2014-03-14 11:36 test\test\
文件 4373 2001-01-23 21:20 test\test\Curvefit.cpp
文件 504 2000-01-14 15:44 test\test\Curvefit.h
目录 0 2014-03-14 11:36 test\test\Debug\
文件 89804 2011-03-09 16:14 test\test\Debug\testDlg.obj
文件 31261 2014-03-07 11:18 test\test\Digistatic.cpp
文件 6863 2014-03-07 12:41 test\test\Digistatic.h
文件 2966 2002-09-03 13:09 test\test\MemDC.h
文件 36864 2014-03-04 21:09 test\test\PCI9052Dll.dll
文件 286 2014-03-04 21:09 test\test\PCI9052Dll.h
文件 3808 2014-03-04 21:09 test\test\PCI9052Dll.lib
文件 2815 2011-03-09 15:37 test\test\ReadMe.txt
目录 0 2014-03-14 11:36 test\test\Release\
文件 129306 2011-03-09 16:13 test\test\Release\testDlg.obj
文件 2915 2014-03-08 13:11 test\test\Resource.h
文件 1532 2000-01-21 11:35 test\test\Rgbcolor.h
目录 0 2014-03-14 11:36 test\test\res\
文件 45718 2014-03-07 11:07 test\test\res\CrossRoad.bmp
文件 598 2014-03-07 00:06 test\test\res\GreenLight.bmp
文件 8958 2014-03-06 23:36 test\test\res\NSGo.bmp
文件 598 2014-03-07 00:07 test\test\res\RedLight.bmp
文件 1270 2014-03-06 23:36 test\test\res\Stop.bmp
文件 12800 2011-03-09 16:11 test\test\res\Thumbs.db
文件 8958 2014-03-06 23:36 test\test\res\WEGo.bmp
文件 598 2014-03-07 00:08 test\test\res\YellowLight.bmp
文件 1270 2014-03-06 23:31 test\test\res\bitmap2.bmp
文件 566 2014-03-06 23:42 test\test\res\bitmap3.bmp
文件 822 2014-03-07 16:07 test\test\res\test.bmp
文件 4286 2014-03-07 16:08 test\test\res\test.ico
文件 396 2014-03-06 21:41 test\test\res\test.rc2
............此处省略14个文件信息
- 上一篇:VINS论文推倒及代码解析
- 下一篇:雷达测角方法研究
相关资源
- 基于proteus的单片机仿真--交通灯控制
- 基于单片机交通灯设计
- 基于VHDL的交通灯控制器设计
- 数字电路课程设计—交通灯
- 8086交通灯 Proteus原理图及程序
- Quartus EDA交通灯控制电路的设计实训报
- 扎努西电气机械天津压缩机有限公司
- 8086微机应用DAC0832控制小直流电机转速
- 微机原理及应用(黄冰覃伟年黄知超
- 微机课程设计_移动靶射击
- Quartusii十字交叉路口三色交通灯设计
- 微机原理 十字路口交通灯控制 prot
- 基于Verilog的交通灯设计EDA课程设计
- 数电交通灯 Multisim 13.0 仿真程序已验
- 基于51单片机的交通灯设计
- 交通灯原理图、PCB3d图、Solidworks外壳
- 基于AT89C51的十字路口交通灯设计pro
- 微机实验,交通信号灯控制系统
- 交通灯模拟程序十字路口
- 十字路口交通灯设计
- 基于Multisim10的十字路口交通灯控制器
- 基于51单片机的智能十字路口交通灯系
- 十字路口交通灯.pdsprj
- 交通灯控制实验(计算机组成原理)
- 数电设计 交通灯控制逻辑电路设计
- 基于LabVIEW的交通灯设计,报告,程序
- 王忠民版微机原理复习要点
- 西电人工智能课件
- 基于Multisim10的十字路口交通灯控制器
- 微机原理的课设
评论
共有 条评论