资源简介
用python+pygame实现的飞机大战的源码,包含资源,可直接运行。通过鼠标控制。
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 绘画背景
screen.blit(background,(0, 0))
# 检测游戏状态
if not gameover:
# 定位鼠标的x, y坐标
x, y = pygame.mouse.get_pos()
# 发射子弹
interval_b -= 1
if interval_b < 0:
bullets[index_b].restart()
interval_b = 100
index_b = (index_b + 1) % count_b
for b in bullets:
if b.active:
# 检查子弹命中情况
for e in enemies:
# 击中敌机,分数加100
if checkHit(e, b):
score += 100
b.move()
screen.blit(b.image, (b.x, b.y))
# 绘画机群
for e in enemies:
e.move()
screen.blit(e.image, (e.x, e.y))
if checkCrash(e, plane):
gameover = True
e.move()
screen.blit(e.image, (e.x, e.y))
plane.move()
screen.blit(plane.image, (plane.x, plane.y))
# 屏幕左上角显示分数
text = font.render("Score: %d" % score, 1, (0, 0, 0))
screen.blit(text, (0, 0))
else:
text = font.render("Score: %d" % score, 1, (0, 0, 0))
screen.blit(text, (190, 400))
# 游戏结束后,检测鼠标抬起就“重置游戏”
if gameover and event.type == pygame.MOUSEBUTTONUP:
plane.restart()
for e in enemies:
e.restart()
for b in bullets:
b.active = False
score = 0
gameover = False
pygame.display.update()
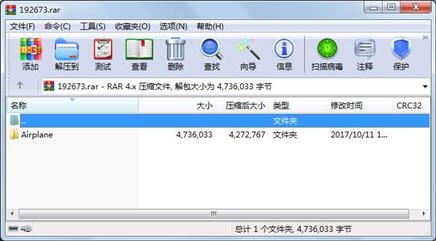
代码片段和文件信息
import pygame
import os
from sys import exit
import random
class Bullet:
def __init__(self):
‘‘‘初始化子弹坐标并加载子弹图片‘‘‘
self.x = 0
self.y = -1
self.image = pygame.image.load(bullet_img).convert_alpha()
self.active = False
def move(self):
‘‘‘移动子弹‘‘‘
if self.active:
self.y -= 3
if self.y < 0:
self.active = False
def restart(self):
mouseX mouseY = pygame.mouse.get_pos()
self.x = x - self.image.get_width()/2
self.y = y - self.image.get_height()/2
self.active = True
class Enemy:
def __init__(self):
self.restart()
self.image = pygame.image.load(enemy_img).convert_alpha()
def restart(self):
self.x = random.randint(50400)
self.y = random.randint(-200-50)
self.speed = random.random() + 0.1
def move(self):
if self.y < 800:
self.y += self.speed
else:
self.restart()
pygame.init()
bg_img = ‘.\\ui\shoot_background\\background.png‘
bg_img2 = ‘bg2.jpg‘
plane_img = ‘.\\ui\\plane.png‘
bullet_img = ‘.\\ui\\bullet.png‘
enemy_img = ‘.\\ui\\enemy.png‘
screen = pygame.display.set_mode((450 800) 0 32)
pygame.display.set_caption(‘Hello World!‘)
background = pygame.image.load(bg_img).convert()
plane = pygame.image.load(plane_img).convert_alpha()
bullet = Bullet()
enemy = Enemy() # 建立敌机
bullets = []
for i in range(5):
bullets.append(Bullet())
count_b = len(bullets) # 子弹的总数
index_b = 0 # 即将激活的子弹序号
interval_b = 0 # 发射子弹的间隔
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 绘画背景
screen.blit(background(0 0))
# 定位鼠标的x y坐标
x y = pygame.mouse.get_pos()
# 绘画敌机到屏幕上
enemy.move()
screen.blit(enemy.image (enemy.x enemy.y))
# 发射子弹
interval_b -= 1
if interval_b < 0:
bullets[index_b].restart()
interval_b = 100
index_b = (index_b + 1) % count_b
for b in bullets:
if b.active:
b.move()
screen.blit(b.image (b.x b.y))
# 定位并绘画飞机到屏幕上
x -= plane.get_width()/2
y -= plane.get_height()/2
screen.blit(plane (x y))
pygame.display.update()
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 4714 2014-07-17 15:26 Airplane\121.png
文件 68134 2014-12-27 16:16 Airplane\bg1.jpg
文件 27996 2014-12-27 16:10 Airplane\bg2.jpg
文件 1679 2013-08-29 08:17 Airplane\font\font.fnt
文件 5193 2013-08-29 08:17 Airplane\font\font.png
文件 1437502 2014-12-27 19:35 Airplane\game04.gif
文件 6590 2014-07-17 15:58 Airplane\game_Reagain.png
文件 6871 2014-07-17 15:57 Airplane\game_start.png
文件 1278671 2014-12-27 19:19 Airplane\PyGame系列-12.pdf
文件 2553 2017-10-11 14:17 Airplane\pygam_hello.py
文件 2979 2017-10-11 14:19 Airplane\pygam_hello_10.py
文件 4102 2017-10-11 14:19 Airplane\pygam_hello_11.py
文件 4903 2017-10-11 15:05 Airplane\pygam_hello_12.py
文件 2553 2017-10-11 14:18 Airplane\pygam_hello_8.py
文件 2625 2017-10-11 14:19 Airplane\pygam_hello_9.py
文件 8831 2013-08-29 08:17 Airplane\sound\achievement.mp3
文件 12327 2013-08-29 08:17 Airplane\sound\big_spaceship_flying.mp3
文件 7751 2013-08-29 08:17 Airplane\sound\bullet.mp3
文件 6815 2013-08-29 08:17 Airplane\sound\button.mp3
文件 11287 2013-08-29 08:17 Airplane\sound\enemy1_down.mp3
文件 12847 2013-08-29 08:17 Airplane\sound\enemy2_down.mp3
文件 9415 2013-08-29 08:17 Airplane\sound\enemy3_down.mp3
文件 117728 2013-08-29 08:17 Airplane\sound\game_music.mp3
文件 14615 2013-08-29 08:17 Airplane\sound\game_over.mp3
文件 9653 2013-08-29 08:17 Airplane\sound\get_bomb.mp3
文件 13494 2013-08-29 08:17 Airplane\sound\get_double_laser.mp3
文件 10693 2013-08-29 08:17 Airplane\sound\out_porp.mp3
文件 10703 2013-08-29 08:17 Airplane\sound\use_bomb.mp3
文件 1378 2014-07-21 09:00 Airplane\ui\bkmusic_close.png
文件 1398 2014-07-21 09:00 Airplane\ui\bkmusic_play.png
............此处省略62个文件信息
- 上一篇:ArcGIS_Python入门指南
- 下一篇:python知乎评论爬虫源代码
相关资源
- 二级考试python试题12套(包括选择题和
- pywin32_python3.6_64位
- python+ selenium教程
- PycURL(Windows7/Win32)Python2.7安装包 P
- 英文原版-Scientific Computing with Python
- 7.图像风格迁移 基于深度学习 pyt
- 基于Python的学生管理系统
- A Byte of Python(简明Python教程)(第
- Python实例174946
- Python 人脸识别
- Python 人事管理系统
- 基于python-flask的个人博客系统
- 计算机视觉应用开发流程
- python 调用sftp断点续传文件
- python socket游戏
- 基于Python爬虫爬取天气预报信息
- python函数编程和讲解
- Python开发的个人博客
- 基于python的三层神经网络模型搭建
- python实现自动操作windows应用
- python人脸识别(opencv)
- python 绘图(方形、线条、圆形)
- python疫情卡UN管控
- python 连连看小游戏源码
- 基于PyQt5的视频播放器设计
- 一个简单的python爬虫
- csv文件行列转换python实现代码
- Python操作Mysql教程手册
- Python Machine Learning Case Studies
- python获取硬件信息
评论
共有 条评论