-
大小: 8.38MB文件类型: .zip金币: 2下载: 0 次发布日期: 2023-10-07
- 语言: Python
- 标签: tensorflow 目标检测
资源简介
tensorflow目标检测代码,从摄像头或者视频源进行任务目标检测
python2.7
tensorflow
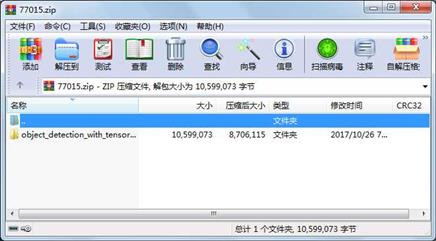
代码片段和文件信息
import numpy as np
import os
import six.moves.urllib as urllib
import sys
import tarfile
import tensorflow as tf
import zipfile
import multiprocessing
from multiprocessing import Queue
import time
import argparse
import cv2
from myutil import downloadutil fps_measure
from object_detection.utils import label_map_util
from object_detection.utils import visualization_utils as vis_util
arg_parser = argparse.ArgumentParser()
arg_parser.add_argument(‘-v‘ ‘--video‘ type=str required=True
help=“video file for detection“)
args = arg_parser.parse_args()
# What model to download.
MODEL_NAME = ‘ssd_mobilenet_v1_coco_11_06_2017‘
def load_graph(model_name=‘ssd_mobilenet_v1_coco_11_06_2017‘):
MODEL_FILE = MODEL_NAME + ‘.tar.gz‘
DOWNLOAD_base = ‘http://download.tensorflow.org/models/object_detection/‘
# Path to frozen detection graph. This is the actual model that is used for the object detection.
PATH_TO_CKPT = MODEL_NAME + ‘/frozen_inference_graph.pb‘
downloadutil.maybe_download(os.getcwd() MODEL_FILE
DOWNLOAD_base+MODEL_FILE)
tar_file = tarfile.open(MODEL_FILE)
for file in tar_file.getmembers():
file_name = os.path.basename(file.name)
if ‘frozen_inference_graph.pb‘ in file_name:
tar_file.extract(file os.getcwd())
# load graph
detection_graph = tf.Graph()
with detection_graph.as_default():
od_graph_def = tf.GraphDef()
with tf.gfile.GFile(PATH_TO_CKPT ‘rb‘) as fid:
serialized_graph = fid.read()
od_graph_def.ParseFromString(serialized_graph)
tf.import_graph_def(od_graph_def name=‘‘)
return detection_graph
NUM_CLASSES = 90
def load_label_map(label_map_name num_class):
# List of the strings that is used to add correct label for each box.
PATH_TO_LABELS = os.path.join(‘data‘ label_map_name)
# load label map
label_map = label_map_util.load_labelmap(PATH_TO_LABELS)
categories = label_map_util.convert_label_map_to_categories(label_map
max_num_classes=num_class use_display_name=True)
category_index = label_map_util.create_category_index(categories)
return category_index
# def load_image_into_numpy_array(image):
# # import pdb; pdb.set_trace()
# # (im_width im_height) = image.size
# (im_width im_height) = image.shape
# # return np.array(image.getdata()).reshape(
# # (im_height im_width 3)).astype(np.uint8)
# return image.reshape((im_height im_width 3)).astype(np.uint8)
# def detect_object(detection_graph image category_index):
# with detection_graph.as_default():
# with tf.Session(graph=detection_graph) as sess:
# # Definite input and output Tensors for detection_graph
# image_tensor = detection_graph.get_tensor_by_name(‘image_tensor:0‘)
# # Each box represents a part of the image where a particular object was detected.
# detection_boxes
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
目录 0 2017-10-26 07:36 ob
文件 1192 2017-10-26 07:36 ob
目录 0 2017-10-26 07:36 ob
文件 5056 2017-10-26 07:36 ob
文件 705 2017-10-26 07:36 ob
文件 1532 2017-10-26 07:36 ob
目录 0 2017-10-26 07:36 ob
文件 1827353 2017-10-26 07:36 ob
文件 1512000 2017-10-26 07:36 ob
文件 876595 2017-10-26 07:36 ob
文件 1071 2017-10-26 07:36 ob
目录 0 2017-10-26 07:36 ob
文件 733 2017-10-26 07:36 ob
文件 543 2017-10-26 07:36 ob
文件 427 2017-10-26 07:36 ob
目录 0 2017-10-26 07:36 ob
目录 0 2017-10-26 07:36 ob
文件 1267 2017-10-26 07:36 ob
文件 8499 2017-10-26 07:36 ob
文件 2950 2017-10-26 07:36 ob
文件 12824 2017-10-26 07:36 ob
文件 11513 2017-10-26 07:36 ob
文件 0 2017-10-26 07:36 ob
目录 0 2017-10-26 07:36 ob
文件 2281 2017-10-26 07:36 ob
文件 3837 2017-10-26 07:36 ob
文件 4213 2017-10-26 07:36 ob
文件 6346 2017-10-26 07:36 ob
文件 5900 2017-10-26 07:36 ob
文件 2364 2017-10-26 07:36 ob
文件 2396 2017-10-26 07:36 ob
............此处省略261个文件信息
相关资源
- DeepLabV3-Tensorflow-master
- 基于TensorFlow实现CNN文本分类实验指导
- 图片分类,图像识别,目标检测
- tensorflow2.0 yolo3目标检测算法
- tensorflow制作自己的灰度图像数据集并
- anaconda下安装tensorflow(注:不同版本
- 北京大学曹健老师-人工智能实践:
- Deep Learning With Python - Jason Brownlee
- Python-自然场景文本检测PSENet的一个
- Python-高效准确的EAST文本检测器的一个
- Python-TensorFlow弱监督图像分割
- Python-基于tensorflow实现的用textcnn方法
- Python-subpixel利用Tensorflow的一个子像素
- 【官方文档】TensorFlow Python API docume
-
tensorflow画风迁移代码 st
yle transfer - 10行Python代码实现目标检测
- 简单粗暴 TensorFlow
- 5. 深度学习中的目标检测 python代码实
- [PDF] Reinforcement Learning With Open AI Tens
- 基于Python的手写字体识别系统
- 基于Tensorflow的人脸识别源码
- python TensorFlow 官方文档中文版
- Python-在TensorFlow中实现实现图像卷积网
- tensorflow-1.9.0-cp37-cp37m-win_amd64.whl
- Faster-RCNN-TensorFlow-Python3.5-master
- 聊天机器人tensorflow
- caffe模型转化为tensorflow模型
- Python-一个非常简单的BiLSTMCRF模型用于
- Python-Tensorflow仿AlphaGo框架实现的AI围棋
- yolo目标检测、识别、跟踪
评论
共有 条评论