资源简介
使用python pygame实现的简单的网络游戏客户端和服务器端
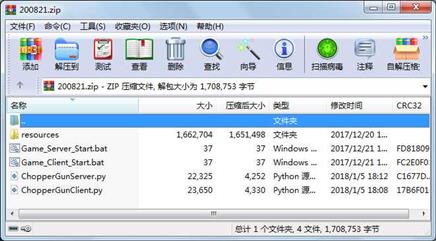
代码片段和文件信息
# coding=utf8
‘‘‘
Game client
‘‘‘
import threading
import time
from random import randint as rint
import pygame
import queue
from pygame.locals import *
from twisted.internet import reactor
from twisted.internet.protocol import Protocol ClientFactory
ctrl_q = queue.Queue()
pos_q = queue.LifoQueue()
enemy_q = queue.Queue()
‘‘‘
The game
‘‘‘
class MySprite(pygame.sprite.Sprite):
def __init__(self):
pygame.sprite.Sprite.__init__(self)
self.master_image = None
self.frame = 0
self.old_frame = -1
self.frame_width = 1
self.frame_height = 1
self.first_frame = 0
self.last_frame = 0
self.columns = 1
self.last_time = 0
self.direction = 0
self.velocity = Point(0.0 0.0)
# X property
def _getx(self):
return self.rect.x
def _setx(self value):
self.rect.x = value
X = property(_getx _setx)
# Y property
def _gety(self):
return self.rect.y
def _sety(self value):
self.rect.y = value
Y = property(_gety _sety)
# position property
def _getpos(self):
return self.rect.topleft
def _setpos(self pos):
self.rect.topleft = pos
position = property(_getpos _setpos)
def load(self filename width height columns):
self.master_image = pygame.image.load(filename).convert_alpha()
self.frame_width = width
self.frame_height = height
self.rect = Rect(0 0 width height)
self.columns = columns
# try to auto-calculate total frames
rect = self.master_image.get_rect()
self.last_frame = (rect.width // width) * (rect.height // height) - 1
def update(self current_time rate=30):
# update animation frame number
if current_time > self.last_time + rate:
self.frame += 1
if self.frame > self.last_frame:
self.frame = self.first_frame
self.last_time = current_time
# build current frame only if it changed
if self.frame != self.old_frame:
frame_x = (self.frame % self.columns) * self.frame_width
frame_y = (self.frame // self.columns) * self.frame_height
rect = Rect(frame_x frame_y self.frame_width self.frame_height)
self.image = self.master_image.subsurface(rect)
self.old_frame = self.frame
def __str__(self):
return str(self.frame) + ““ + str(self.first_frame) + \
““ + str(self.last_frame) + ““ + str(self.frame_width) + \
““ + str(self.frame_height) + ““ + str(self.columns) + \
““ + str(self.rect)
# Point class
class Point(object):
def __init__(self x y):
self.__x = x
self.__y = y
# X property
def getx(self): return self.__x
def setx(self x): self.__x
属性 大小 日期 时间 名称
----------- --------- ---------- ----- ----
文件 23650 2018-01-05 18:08 ChopperGunClient.py
文件 22325 2018-01-05 18:12 ChopperGunServer.py
文件 37 2017-12-21 17:20 Game_Client_Start.bat
文件 37 2017-12-21 17:20 Game_Server_Start.bat
目录 0 2017-12-20 17:19 resources\
目录 0 2017-12-20 18:30 resources\images\
文件 209 2017-12-20 18:27 resources\images\bullet2.png
文件 17179 2017-12-20 18:28 resources\images\enemychopper.png
文件 38767 2017-12-20 18:28 resources\images\flame.png
文件 1864 2017-12-20 18:29 resources\images\missile.png
文件 17850 2017-12-21 11:13 resources\images\pla
文件 650315 2017-12-13 19:44 resources\images\skybg.jpg
文件 814860 2017-12-20 18:30 resources\images\skybg.png
文件 21351 2017-12-20 18:29 resources\images\warship.png
文件 96780 2017-12-20 18:29 resources\images\warship_flame.png
文件 3529 2017-12-20 18:29 resources\images\warship_missile.png
- 上一篇:数据挖掘—数据.rar
- 下一篇:MLP/RNN/LSTM模型进行IMDb情感分析
相关资源
- 二级考试python试题12套(包括选择题和
- pywin32_python3.6_64位
- python+ selenium教程
- PycURL(Windows7/Win32)Python2.7安装包 P
- 英文原版-Scientific Computing with Python
- 7.图像风格迁移 基于深度学习 pyt
- 基于Python的学生管理系统
- A Byte of Python(简明Python教程)(第
- Python实例174946
- Python 人脸识别
- Python 人事管理系统
- 基于python-flask的个人博客系统
- 计算机视觉应用开发流程
- python 调用sftp断点续传文件
- python socket游戏
- 基于Python爬虫爬取天气预报信息
- python函数编程和讲解
- Python开发的个人博客
- 基于python的三层神经网络模型搭建
- python实现自动操作windows应用
- python人脸识别(opencv)
- python 绘图(方形、线条、圆形)
- python疫情卡UN管控
- python 连连看小游戏源码
- 基于PyQt5的视频播放器设计
- 一个简单的python爬虫
- csv文件行列转换python实现代码
- Python操作Mysql教程手册
- Python Machine Learning Case Studies
- python获取硬件信息
评论
共有 条评论